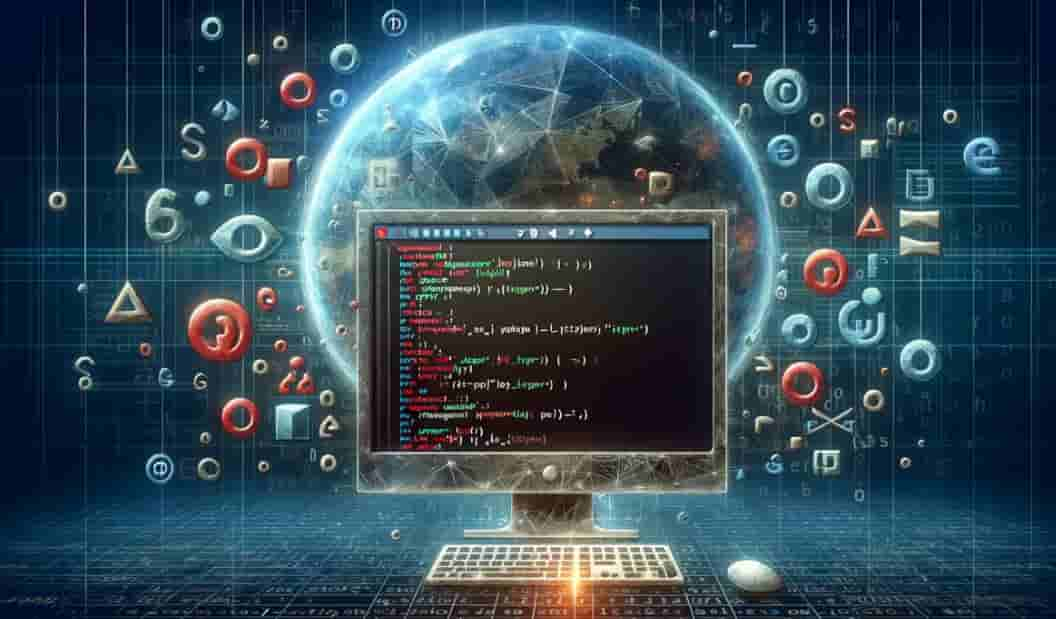
This comprehensive guide is crafted to explore the nuances of the `gettype()` function in PHP. The `gettype()` function is pivotal in PHP programming, particularly for debugging and validating data types. Readers will gain insights into its syntax, practical applications, and several illustrative examples, enhancing their proficiency in utilizing this function.
Understanding the `gettype()` Function in PHP
In PHP, a language that does not require explicit variable type declaration, the `gettype()` function emerges as a crucial tool. It allows programmers to ascertain the data type of a variable, which is essential in various operations and debugging processes. The syntax for employing the `gettype()` function is straightforward:
```php
// Syntax
$type = gettype($variable);
```
`$variable` represents the variable whose data type is being queried.
Exploring PHP Variable Types
In PHP, a language known for its flexibility, the understanding of variable types is crucial for effective coding practices. The `gettype()` function serves as a bridge to this understanding, offering a way to identify the specific type of a given variable. This is particularly important in PHP, a loosely-typed language, where variables do not require explicit declaration of their types, leading to potential ambiguity in data handling.
The types of variables that PHP supports are diverse:
- Integer: This type represents whole numbers;
- Float (Double): Used for decimal and floating-point numbers;
- Boolean: This type has two values `true` and `false`;
- String: A sequence of characters, used for text;
- Array: A collection of values, where each value is identified by a key;
- Object: An instance of a class, representing complex data structures with attributes and methods;
- Resource: A special type representing external resources, like database connections;
- NULL: A type indicating the absence of a value.
Each of these types plays a vital role in the way PHP handles data. For instance, knowing whether a variable is an integer or a float can significantly impact mathematical operations. Similarly, distinguishing between an array and an object is crucial for data manipulation. The `gettype()` function, by providing the ability to discern these types, empowers developers to write more robust, error-free code. It ensures that operations on variables are appropriate for their types, thereby reducing bugs and enhancing code reliability. As such, a deep understanding of these variable types and the adept use of `gettype()` is an indispensable skill for any PHP programmer.
Practical Applications of the `gettype()` Function
Example 1: Basic Implementation
This example demonstrates basic usage of `gettype()` to determine the types of various variables:
```php
<?php
// Different variable types
$integer = 10; $float = 3.14; $boolean = true;
$string = "Hello, world!"; $array = [1, 2, 3];
$object = new stdClass(); $resource = fopen('test.txt', 'r');
$null = null;
// Displaying the types
echo 'Type of $integer: ' . gettype($integer) . '<br>';
// Additional lines follow for each variable
?>
```
Running this code reveals the data type of each variable.
Example 2: Validating User Input
Here, `gettype()` is utilized to validate user inputs for an addition operation:
```php
<?php
function add_numbers($a, $b) {
if (gettype($a) !== 'integer' || gettype($b) !== 'integer') {
echo 'Error: Inputs must be integers.<br>';
return;
}
echo 'Sum: ' . ($a + $b) . '<br>';
}
add_numbers(10, 20); // Valid input
add_numbers(10, 'hello'); // Invalid input
?>
```
This example highlights the use of `gettype()` in ensuring input data types.
Conclusion
The exploration of PHP’s `gettype()` function in this guide illuminates its critical role in software development, particularly in the realms of debugging and data validation. Understanding the nature of variables is paramount in PHP, as it directly influences the logic and functionality of the code. The `gettype()` function acts as a diagnostic tool, enabling developers to swiftly identify and rectify type-related errors, thereby streamlining the development process.
Moreover, the examples presented here not only demonstrate the function’s theoretical aspects but also its practical application in everyday coding situations. These real-life scenarios showcase how `gettype()` can be effectively employed to ensure data integrity and to facilitate type-specific operations, which are common challenges in PHP programming.
For organizations and individuals seeking proficient PHP developers, platforms like Reintech are invaluable. They provide a gateway to a pool of experienced and skilled PHP programmers who are adept at utilizing functions like `gettype()` to their full potential. This access to top-tier talent is essential for projects demanding high-quality PHP development, ensuring that the code is not only functional but also robust and efficient. In essence, this guide not only educates about the `gettype()` function but also highlights the importance of skilled developers who can apply such knowledge to meet diverse project requirements effectively.