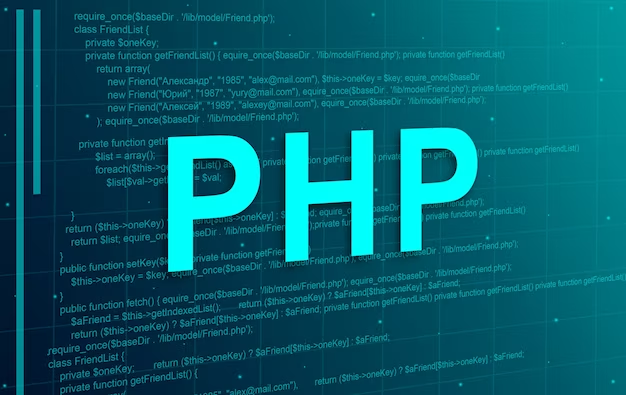
Object-oriented programming (OOP) stands as a dominant paradigm in software development, renowned for fostering organized, efficient, and modular coding practices. PHP’s support for OOP makes it a versatile choice for web application development. This tutorial is designed for both novice and seasoned developers, emphasizing the importance of understanding classes and objects in PHP, a critical skill set for scalable web application development.
Fundamentals of PHP Classes and Objects
In PHP, a class serves as a template for generating objects, encapsulating a set of attributes (properties) and behaviors (methods). An object represents an instantiation of a class, possessing unique properties and methods as defined in the class blueprint.
Constructing a PHP Class
A PHP class is declared using the class keyword, followed by a descriptive, CamelCase name. The class encapsulates its properties and methods, defining the core structure of the objects it will create.
class MyClass { // Property and method definitions} |
Defining Properties and Methods in Classes
Properties, akin to variables in a class, and methods, similar to functions, are defined with access modifiers (public, private, or protected). This encapsulation dictates their accessibility within and outside the class.
class MyClass { public $property1; private $property2; protected $property3; public function method1() { // Implementation } private function method2() { // Implementation } protected function method3() { // Implementation }} |
Instantiating Objects from PHP Classes
Objects are instantiated using the new keyword, followed by the class name and parentheses. This process creates a new instance of the class with its defined properties and methods.
$object1 = new MyClass(); |
Interacting with Object Properties and Methods
Access and manipulate an object’s properties and methods using the arrow operator (->). Note that only public and protected members are accessible from outside the class.
$object1->property1 = “Hello, world!”;echo $object1->property1; // Outputs: Hello, world!$object1->method1(); |
Practical Example: Implementing a Person Class in PHP
Here’s a simple implementation of a Person class, illustrating the use of properties and methods within a PHP class.
class Person { public $firstName; public $lastName; public function getFullName() { return $this->firstName . ‘ ‘ . $this->lastName; } public function sayHello() { echo “Hello, my name is ” . $this->getFullName() . “.”; }} $person1 = new Person();$person1->firstName = “John”;$person1->lastName = “Doe”;$person1->sayHello(); // Output: Hello, my name is John Doe. |
Comparative Table: Properties vs. Methods in PHP Classes
Aspect | Properties in PHP Classes | Methods in PHP Classes |
---|---|---|
Definition | Variables that belong to a class, representing the attributes or characteristics of an object. | Functions that belong to a class, representing the actions or behaviors of an object. |
Declaration | Declared with access modifiers (public, private, protected) followed by the variable name. | Declared with access modifiers, followed by the function name and parentheses for parameters. |
Accessibility | Can be accessed and modified (subject to access level) directly using the object. | Invoked using the object, can accept parameters, and return values. |
Usage | Used to store and maintain the state of an object. | Used to perform operations, computations, or actions, potentially altering the state of an object. |
Example | public $firstName; | public function getFullName() { return $this->firstName . ‘ ‘ . $this->lastName; } |
Conclusion
This tutorial has demystified the creation and utilization of PHP classes and objects, laying the foundation for building structured, efficient, and modular applications. Understanding these principles is pivotal for any PHP developer, especially when considering hiring for web development projects.