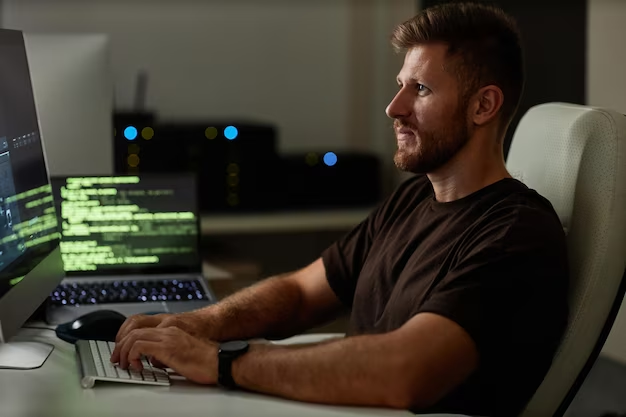
PHP’s array_slice() function is a pivotal tool for developers, designed to segment arrays efficiently. This function is instrumental in extracting specific portions of an array, based on specified indices and lengths, optimizing data handling in PHP programming.
Parameters of array_slice()**
The array_slice()` function is characterized by its versatile parameters:
array_slice(array $array, int $offset, int $length = null, bool $preserve_keys = false) |
- $array: The target array for segmentation;
- $offset: Starting index for extraction. Positive values start from the beginning, while negative values count from the end of the array;
- $length (optional): Number of elements to extract. The absence of this parameter leads to extraction till the array’s end. Negative values exclude elements from the array’s end;
- $preserve_keys (optional): When true, maintains original array keys in the extracted segment.
Basic Application of `array_slice()
For a basic use case, consider extracting a segment starting from a specific index:
$array = [“apple”, “banana”, “cherry”, “date”, “fig”, “grape”];$portion = array_slice($array, 2);print_r($portion); |
- Output:
Array( [0] => cherry [1] => date [2] => fig [3] => grape) |
Advanced Utilization: Specifying Length
To control the length of the extracted segment:
$array = [“apple”, “banana”, “cherry”, “date”, “fig”, “grape”];$portion = array_slice($array, 2, 3);print_r($portion); |
- Output:
Array( [0] => cherry [1] => date [2] => fig) |
Negative Indexing in array_slice()
Utilizing negative values for $offset and $length offers reverse indexing:
$array = [“apple”, “banana”, “cherry”, “date”, “fig”, “grape”];$portion = array_slice($array, -3, -1);print_r($portion); |
- Output:
Array( [0] => date [1] => fig) |
Maintaining Key Integrity with array_slice()**
Preserve original array keys by setting $preserve_keys` to true:
$array = [“apple”, “banana”, “cherry”, “date”, “fig”, “grape”];$portion = array_slice($array, 2, 3, true);print_r($portion); |
- Output:
Array( [2] => cherry [3] => date [4] => fig) |
Comparative Table: Different Uses of array_slice() in PHP
Parameter Scenario | Description | Example Input | Example Output |
---|---|---|---|
Positive Offset | Extracts from the specified index to the end or specified length | array_slice($array, 2) | Extracts elements starting from index 2 |
Negative Offset | Starts extraction from the end of the array | array_slice($array, -3) | Extracts the last three elements |
Specified Length | Limits the number of elements extracted | array_slice($array, 2, 3) | Extracts three elements starting from index 2 |
Negative Length | Excludes elements from the end | array_slice($array, 2, -1) | Extracts elements starting from index 2, excluding the last element |
Preserve Keys (True) | Maintains original array keys | array_slice($array, 2, 3, true) | Extracts three elements starting from index 2 with original keys |
Preserve Keys (False) | Re-indexes the extracted portion | array_slice($array, 2, 3, false) | Extracts three elements starting from index 2 and re-indexes them |
PHP Hash Password: Securing User Data with Robust Hashing Techniques
In the realm of web development, safeguarding user passwords is paramount. PHP addresses this necessity by offering robust functions for password hashing and verification. These functions ensure that passwords are securely stored in a hashed format, greatly enhancing security.
PHP’s Built-in Password Hashing Functions:
PHP provides several built-in functions for secure password handling:
- password_hash(): Generates a secure hash of a user’s password;
- password_verify(): Validates a submitted password against its hashed version;
- password_needs_rehash(): Determines if an existing hash meets current security standards.
Using password_hash()
The password_hash() function is straightforward to use. It requires a password and a hashing algorithm, typically PASSWORD_DEFAULT, which PHP automatically updates to newer, more secure algorithms over time.
Example:
$password = “userpassword”;$hash = password_hash($password, PASSWORD_DEFAULT); |
Validating with password_verify()
To verify a password during user login, password_verify() compares the submitted password against the stored hash.
Example:
if (password_verify($password, $storedHash)) { // Password is valid} else { // Invalid password} |
Conclusion
The array_slice() function is an essential component in PHP for effective array manipulation. This guide has illuminated its functionality with practical examples, emphasizing its importance in PHP development. For organizations seeking PHP developers, a thorough understanding of array_slice() is a testament to their proficiency in array handling.