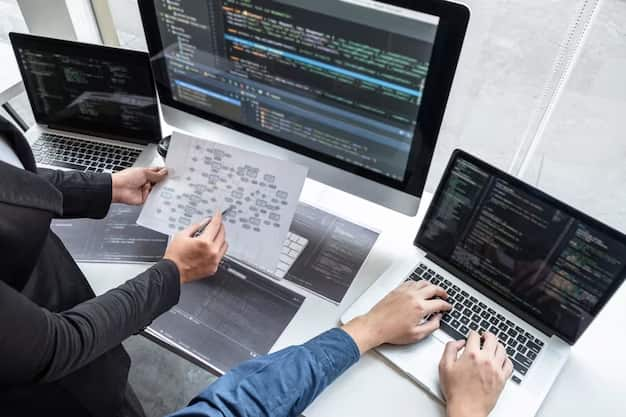
Password hashing is an essential component of web application security. It involves converting plain-text passwords into a cryptic string, a process vital to safeguarding user data. PHP, with its robust library, offers efficient means to hash passwords, thereby fortifying the security of web applications.
PHP’s Integral Password Hashing Functions
PHP comes equipped with a suite of functions dedicated to password hashing and verification. These include:
- password_hash(): Generates a secure hash of a password;
- password_verify(): Confirms the authenticity of a hashed password;
- password_needs_rehash(): Determines the necessity of rehashing a password, typically for enhanced security or algorithm updates.
Implementing the password_hash() Function
The password_hash() function in PHP creates a secure hash using robust algorithms.
- Syntax:
string password_hash ( string $password , int $algo [, array $options ] ) |
- Example:
$password = “mysecurepassword”;$hash = password_hash($password, PASSWORD_DEFAULT);echo $hash; |
Validating Passwords with password_verify()
To authenticate a user’s password against its hash, password_verify() is used.
- Syntax:
bool password_verify ( string $password , string $hash ) |
- Example:
$password = “mysecurepassword”;$storedHash = ‘…’; // Retrieved from database if (password_verify($password, $storedHash)) { echo “Password is valid!”;} else { echo “Invalid password!”;} |
Updating Password Hashes: The Role of password_needs_rehash()
The password_needs_rehash() function is critical for maintaining updated, and secure password hashes.
- Example:
if (password_needs_rehash($storedHash, PASSWORD_DEFAULT, [‘cost’ => 12])) { $newHash = password_hash($password, PASSWORD_DEFAULT, [‘cost’ => 12]); // Update stored hash in the database} |
Comparative Table: PHP Password Hashing Functions
Function | Purpose | Usage | Example Scenario |
---|---|---|---|
password_hash() | Generates a secure hash of a password. | Used when storing a new password or updating an existing one. | When a user registers or changes their password. |
password_verify() | Validates a password against a stored hash. | Used during user authentication to verify the password entered by the user. | When a user logs in to the system. |
password_needs_rehash() | Checks if a password hash needs to be rehashed. | Used to determine if the hashing algorithm or cost factor has changed and the password hash needs to be updated. | When enhancing the security of stored passwords or after an update in PHP’s hashing algorithm. |
cURL in PHP: Mastering Web Data Transfer (200 words)
cURL, standing for ‘Client URL’, is an incredibly versatile tool in PHP, used extensively for sending and receiving data via various network protocols. Its primary strength lies in handling HTTP requests, making it indispensable for web development.
Functionality and Usage
cURL in PHP is instrumental for interacting with APIs, downloading files, and submitting form data remotely. It offers developers the flexibility to tailor HTTP requests, manage cookies, handle sessions, and securely transfer data.
Syntax and Usage
The basic syntax for using cURL in PHP involves initializing a cURL session, setting options for the transfer, executing the session, and then closing it. Here’s a simplified overview:
- Initialize cURL session: $curl = curl_init();
- Set options: curl_setopt($curl, CURLOPT_OPTION_NAME, value);
- Execute session: $response = curl_exec($curl);
- Close session: curl_close($curl).
Example: Making a GET Request
$curl = curl_init(“http://example.com/api/data”);curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);$response = curl_exec($curl);curl_close($curl); |
Example: Making a POST Request
$curl = curl_init(“http://example.com/api/data”);$postData = [‘key1’ => ‘value1’, ‘key2’ => ‘value2’];curl_setopt($curl, CURLOPT_POST, true);curl_setopt($curl, CURLOPT_POSTFIELDS, http_build_query($postData));$response = curl_exec($curl);curl_close($curl); |
Conclusion
Secure password hashing and verification are paramount in web application security. PHP’s built-in functions provide a robust and easy-to-implement solution for managing password security. For developers and organizations seeking to enhance their web applications’ security, expertise in PHP’s password hashing functions is essential.