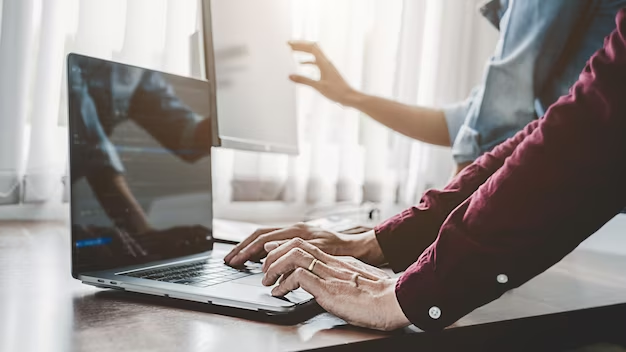
cURL, an acronym for “Client for URLs,” stands as a versatile command-line tool and library in PHP for data transfer using a variety of network protocols. It is highly regarded for its capability to handle HTTP requests and responses programmatically, making it an indispensable tool for PHP developers.
Configuring cURL in PHP
To leverage cURL in PHP, it’s essential to ensure that the cURL extension is activated. In shared hosting environments, this extension is often pre-enabled. To verify and enable cURL:
if (function_exists(‘curl_version’)) { echo “cURL is enabled”;} else { echo “cURL is not enabled”;} |
For activation, locate the php.ini file and uncomment the line containing extension=curl or extension=php_curl.dll. Save changes and restart your server. Follow the official PHP documentation for manual installation if needed.
Implementing Basic HTTP Requests with cURL
cURL in PHP facilitates both GET and POST requests, among others.
- HTTP GET Request: Used to retrieve information from a server, a GET request is straightforward to implement using cURL.
$url = “https://api.example.com/data”;$curl = curl_init($url);curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);$response = curl_exec($curl);curl_close($curl);echo $response; |
- HTTP POST Request: To send data to a server, the POST request is executed with additional options like CURLOPT_POST and CURLOPT_POSTFIELDS.
$url = “https://api.example.com/data”;$data = [“key1” => “value1”, “key2” => “value2”];$curl = curl_init($url);curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);curl_setopt($curl, CURLOPT_POST, true);curl_setopt($curl, CURLOPT_POSTFIELDS, http_build_query($data));$response = curl_exec($curl);curl_close($curl);echo $response; |
Efficiently Managing HTTP Responses
Proper handling of HTTP responses includes error checking and processing different response formats. Use curl_error() and curl_errno() for error detection. For varied response formats like JSON or XML, appropriate parsing methods should be applied.
Exploring Advanced cURL Functionalities
cURL offers a plethora of options to fine-tune HTTP requests, such as:
- CURLOPT_FOLLOWLOCATION: For redirect following;
- CURLOPT_TIMEOUT: To set request timeout;
- CURLOPT_CONNECTTIMEOUT: For connection timeout;
- CURLOPT_HTTPHEADER: To customize HTTP headers;
- CURLOPT_COOKIE: For cookie handling;
- CURLOPT_USERAGENT: To set user agent string;
- SSL/TLS settings: CURLOPT_SSL_VERIFYPEER and CURLOPT_SSL_VERIFYHOST.
Comparative Table: GET vs. POST Requests in cURL
Feature | HTTP GET Request with cURL | HTTP POST Request with cURL |
---|---|---|
Purpose | Primarily used to retrieve data from a server. | Mainly used to submit data to a server for processing. |
cURL Options | Uses curl_init() with URL. CURLOPT_RETURNTRANSFER often set to true. | Requires setting CURLOPT_POST to true and CURLOPT_POSTFIELDS with data. |
Data Handling | Data is sent as part of the URL, typically as query strings. | Data is sent in the request body, allowing more data to be transferred. |
Usage Scenario | Ideal for requesting data or documents, where data is not sensitive. | Preferred for form submissions, especially when handling sensitive data. |
Security | Less secure as data is exposed in URL. | More secure as data is not exposed in URL. |
Example | php curl_setopt($curl, CURLOPT_URL, “http://example.com/api?param=value”); | php curl_setopt($curl, CURLOPT_POSTFIELDS, “param=value”); |
PHP Array Map: Elevating Array Manipulation in PHP
The array_map() function in PHP stands as a pivotal tool for array manipulation, offering a streamlined, functional approach to modifying array elements. This function applies a user-defined callback to each element of one or more arrays, returning a new array of elements obtained by applying the callback.
Functionality and Usage
The array_map() function shines in scenarios where each element of an array requires a transformation or operation. It simplifies code, enhances readability, and maintains the principle of immutability in functional programming.
Syntax:
array_map(callback, array1, array2, …) |
In this syntax, callback is the function applied to each element, and array1, array2, etc., are the arrays passed to the function.
Example of Single Array Manipulation:
function square($num) { return $num * $num;} $numbers = [1, 2, 3, 4, 5];$squared = array_map(‘square’, $numbers);print_r($squared); |
Conclusion
This comprehensive guide to cURL in PHP equips developers with the knowledge to execute HTTP requests efficiently and handle responses effectively. Understanding cURL’s capabilities is crucial for developers aiming to build sophisticated PHP applications that require interaction with web services and APIs. For projects demanding skilled PHP developers proficient in cURL, Reintech offers a platform to connect with experienced professionals.