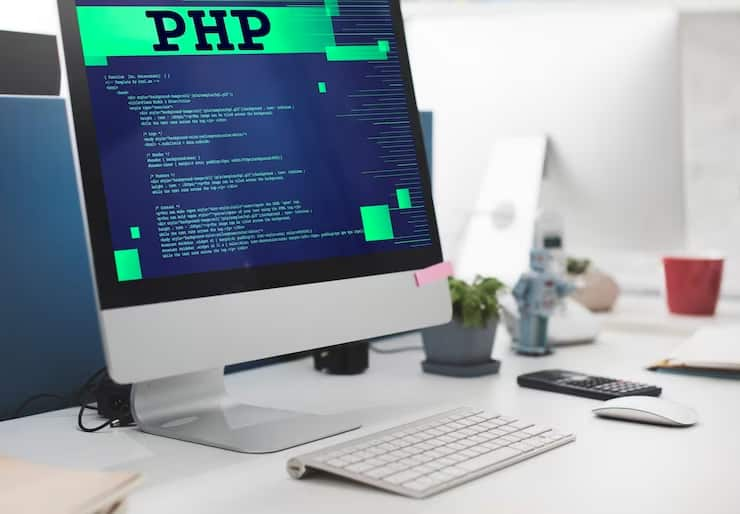
As a software developer navigating the PHP landscape, mastering array manipulation is indispensable. This tutorial dives into a pivotal PHP function for array manipulation: array_column().
This comprehensive guide not only explains the function’s nuances but also provides additional insights and advanced techniques for harnessing its power.
What is array_column()?
The array_column() function, intrinsic to PHP, serves as a linchpin for extracting specific column values from multi-dimensional arrays or arrays of objects. Its versatility shines through by allowing developers to index the resulting array with another column, unleashing a myriad of possibilities.
Function Signature
array_column(array $input, mixed $columnKey, mixed $indexKey = null)
Parameters:
- $input: The multi-dimensional array or array of objects from which to extract a column;
- $columnKey: The key representing the column to extract (or property name for objects);
- $indexKey (optional): The key (or property name) to use as the index/keys for the resulting array. If omitted or set to null, numeric keys are assigned.
Return Value
The return value is an array containing the extracted values, with the option to index them using the provided $indexKey. This versatility makes array_column() indispensable for developers seeking efficient array manipulation.
Usage Examples
Let’s venture into practical examples to grasp the versatility and power of array_column().
Example 1: Basic Usage with a Numerical Array
Consider a scenario where we have a multidimensional array representing users. Using array_column(), we effortlessly extract an array of ages.
Unleash advanced search techniques with strpos() in PHP.
Example 2: Using an Index Key
In this example, we aim to extract user names while indexing the resulting array by user IDs. This showcases the flexibility of array_column().
Example 3: Working with an Array of Objects
Demonstrating its adaptability, array_column() seamlessly works with an array of objects. Explore how it extracts ages while indexing by user IDs.
Example 4: Complex Data Structures
Consider a scenario with a more intricate array structure:
$employees = [
['id' => 101, 'name' => 'John Doe', 'salary' => 5000, 'department' => 'IT'],
['id' => 102, 'name' => 'Jane Smith', 'salary' => 6000, 'department' => 'HR'],
// ...more entries
];
If we aim to extract the salaries indexed by the employee IDs, array_column() effortlessly accommodates:
$salaries = array_column($employees, 'salary', 'id');
print_r($salaries);
Output:
Array
(
[101] => 5000
[102] => 6000
// ...more entries
)
Example 5: Advanced Object Manipulation
Extend the application of array_column() to objects with calculated properties:
class Employee {
public $id;
public $name;
public $salary;
public function __construct($id, $name, $salary) {
$this->id = $id;
$this->name = $name;
$this->salary = $salary;
}
public function getBonus() {
// Example of a calculated property
return $this->salary * 0.1; // 10% bonus
}
}
$employeeObjects = [
new Employee(201, 'Michael Johnson', 5500),
new Employee(202, 'Emily Davis', 6500),
// ...more instances
];
// Extracting salary and bonus using array_column()
$earnings = array_column($employeeObjects, function($employee) {
return $employee->salary + $employee->getBonus();
}, 'id');
print_r($earnings);
Output:
Array
(
[201] => 6050
[202] => 7150
// ...more entries
)
Best Practices Reinforcement
Incorporating array_column() into your arsenal warrants a mindful approach. Here are some additional best practices:
Custom Transformations:
Utilize anonymous functions for custom transformations. This opens avenues for dynamic data manipulations, enhancing the function’s adaptability.
$transformedData = array_column($input, function($item) {
// Custom transformation logic
return $item['value'] * 2;
}, 'id');
Conclusion
Expanding your proficiency with the array_column() function equips you with a potent tool for array manipulation in PHP. By incorporating diverse examples and best practices, this guide empowers developers to wield this function effectively.
Elevate your coding prowess, experiment with real-world scenarios, and embrace the enhanced capabilities that array_column() brings to your PHP projects. If you’re hiring PHP developers, ensuring their familiarity with this essential function is a key criterion for a robust development team.