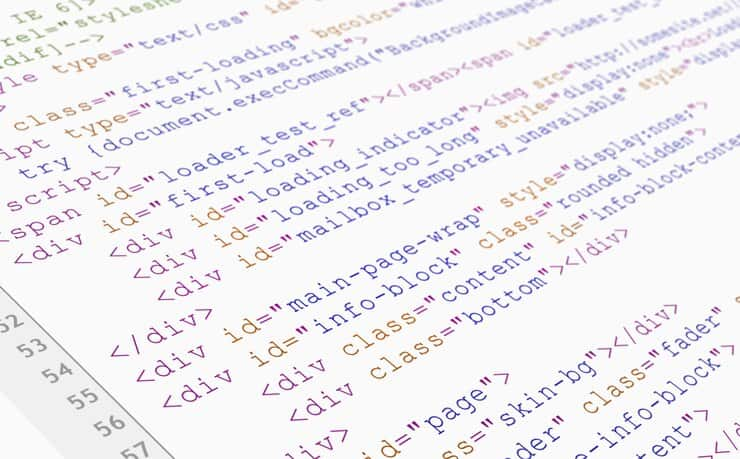
In this exploration, we will shift our focus from the intricacies of `strpos()` and `stripos()` functions to the sorting of multidimensional arrays in PHP. Elevating your expertise in array manipulation is crucial for effective data organization and retrieval within your PHP applications.
Precision Sorting by a Specific Column
Achieving precision in multidimensional array sorting by a specific column is effortlessly accomplished using `array_multisort()` coupled with `array_column()`:
array_multisort(array_column($inventory, 'quantity'), $inventory);
This example effortlessly sorts the array based on the ‘quantity’ column, providing a streamlined approach to organize your data.
Descending Order Mastery
To harness the power of descending order sorting, a simple modification to the `array_multisort()` call is all it takes:
array_multisort(array_column($inventory, 'quantity'), SORT_DESC, $inventory);
Now, your array is arranged in descending order, allowing for versatile data presentation tailored to your specific requirements.
Master advanced search techniques by exploring the power of strpos() in PHP.
Implementing Custom Sorting Criteria
When your sorting needs become intricate, turn to `usort()` for implementing custom sorting logic. This example demonstrates sorting users based on age and activity score:
usort($users, function ($a, $b) {
if ($a['age'] == $b['age']) {
return $b['activity_score'] - $a['activity_score'];
}
return $a['age'] - $b['age'];
});
Custom criteria offer flexibility, enabling tailored sorting that goes beyond conventional methods.
Key Preservation with `uasort()`
Maintaining the association between keys and values is critical during sorting. Employ `uasort()` for user-defined sorting with key preservation:
uasort($students, function ($a, $b) {
return $a['roll_number'] - $b['roll_number'];
});
By doing so, the original keys in the array retain their significance, ensuring the integrity of your data structure.
Streamlined Key-Based Sorting
For associative arrays where keys hold equal importance as values, `ksort()` and `krsort()` prove invaluable:
ksort($grades); // Ascending order by keys
krsort($grades); // Descending order by keys
These functions facilitate straightforward key-based sorting, simplifying the process for enhanced data organization.
Conclusion
Mastery in sorting multidimensional arrays is a cornerstone of PHP development, providing the means to efficiently organize and retrieve data. Whether your focus is on numerical sorting, preserving original keys, or implementing custom criteria, PHP offers an array of functions tailored to diverse sorting needs.
By seamlessly integrating these advanced techniques, developers can elevate the functionality and performance of their PHP applications. As you seek PHP developers for your projects, prioritize candidates with proven proficiency in employing these array sorting methods, ensuring optimal data manipulation.