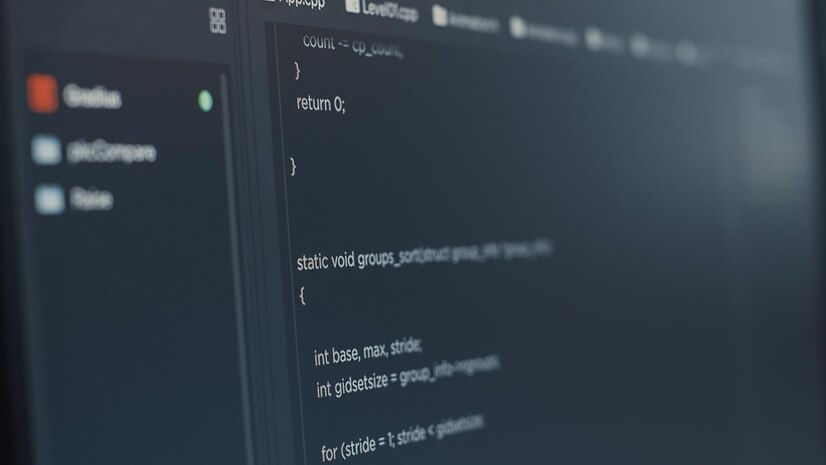
SOAP (Simple Object Access Protocol) stands as the foundational protocol for the structured exchange of information among web services. This tutorial focuses on PHP’s built-in SOAP extension, a crucial tool for seamless interaction with SOAP clients and servers. Whether you’re creating or consuming SOAP web services, a solid command of the PHP SOAP library is essential for effective web development practices.
Before delving into the specifics, ensure PHP is installed on your system to follow along with our guide.
Installing the PHP SOAP Extension
To kick start the process, confirm whether the SOAP extension is both installed and enabled on your PHP setup. Execute the following command:
php -m | grep -i soap
If the command returns “soap,” the extension is ready; otherwise, installation is required. On Linux systems, utilize:
sudo apt-get install php-soap
For Windows, uncomment the following line in your php.ini file:
extension=php_soap.dll
Creating a SOAP Server
The creation of a SOAP server involves defining a WSDL file and implementing a PHP class with the desired functionality. As an example, let’s design a server that facilitates the addition of two numbers.
Create “calculator.wsdl”:
<!-- WSDL content →
Implement the PHP class "Calculator":
class Calculator {
public function addNumbers($num1, $num2) {
return $num1 + $num2;
}
}
Finally, craft "soap-server.php":
// Server setup
Creating a SOAP Client
For a SOAP client, leverage PHP’s built-in SoapClient class. In “soap-client.php”:
$client = new SoapClient('http://localhost/calculator.wsdl');
$result = $client->addNumbers(3, 5);
echo "The sum is: " . $result;
Error Handling and Debugging
Effectively handle SOAP errors using a try-catch block:
try {
// SOAP client logic
} catch (SoapFault $e) {
echo "Error: " . $e->getMessage();
}
Debugging is facilitated with "__getLastRequest()" and "__getLastResponse()" methods:
// Debugging SOAP requests and responses
Expanding SOAP in PHP: Advanced Techniques
Uncover the capabilities of custom SOAP headers to augment your web services’ functionality. Learn to implement and utilize these headers for a more tailored SOAP experience in PHP.
Example: Implementing Custom SOAP Headers
// Custom SOAP header implementation
Comprehend the nuances of document/literal style in SOAP web services. Navigate through the implementation and benefits of this style, optimizing your PHP SOAP development for improved performance.
Unlock the secrets of numeric conversion with our guide on intval in PHP.
Enhancing SOAP Security: WS-Security in PHP
In the realm of SOAP web services, ensuring robust security is paramount.
Example: Implementing WS-Security in PHP
// WS-Security implementation for secure SOAP communication
Optimizing Performance with Asynchronous SOAP Requests
Efficiency is a key concern in web service development.
Example: Asynchronous SOAP Request Handling
// Asynchronous SOAP request handling for improved performance
Handling Complex Data Structures in SOAP
Real-world applications often involve intricate data structures. Understand how PHP’s SOAP library accommodates complex data types and structures.
Example: Managing Complex Data Structures in SOAP
// Handling complex data structures within SOAP services
Integrating SOAP with Modern PHP Frameworks
Explore seamless integration between SOAP and contemporary PHP frameworks. Understand how to incorporate SOAP web services within frameworks like Laravel or Symfony.
Example: Integrating SOAP with Laravel
// Integration of SOAP services within Laravel framework
Conclusion
Mastering security implementations, optimizing performance, and integrating with modern frameworks further solidifies your expertise. When seeking PHP developers, prioritize those equipped with a comprehensive understanding of these advanced SOAP practices for effective and nuanced web service development.