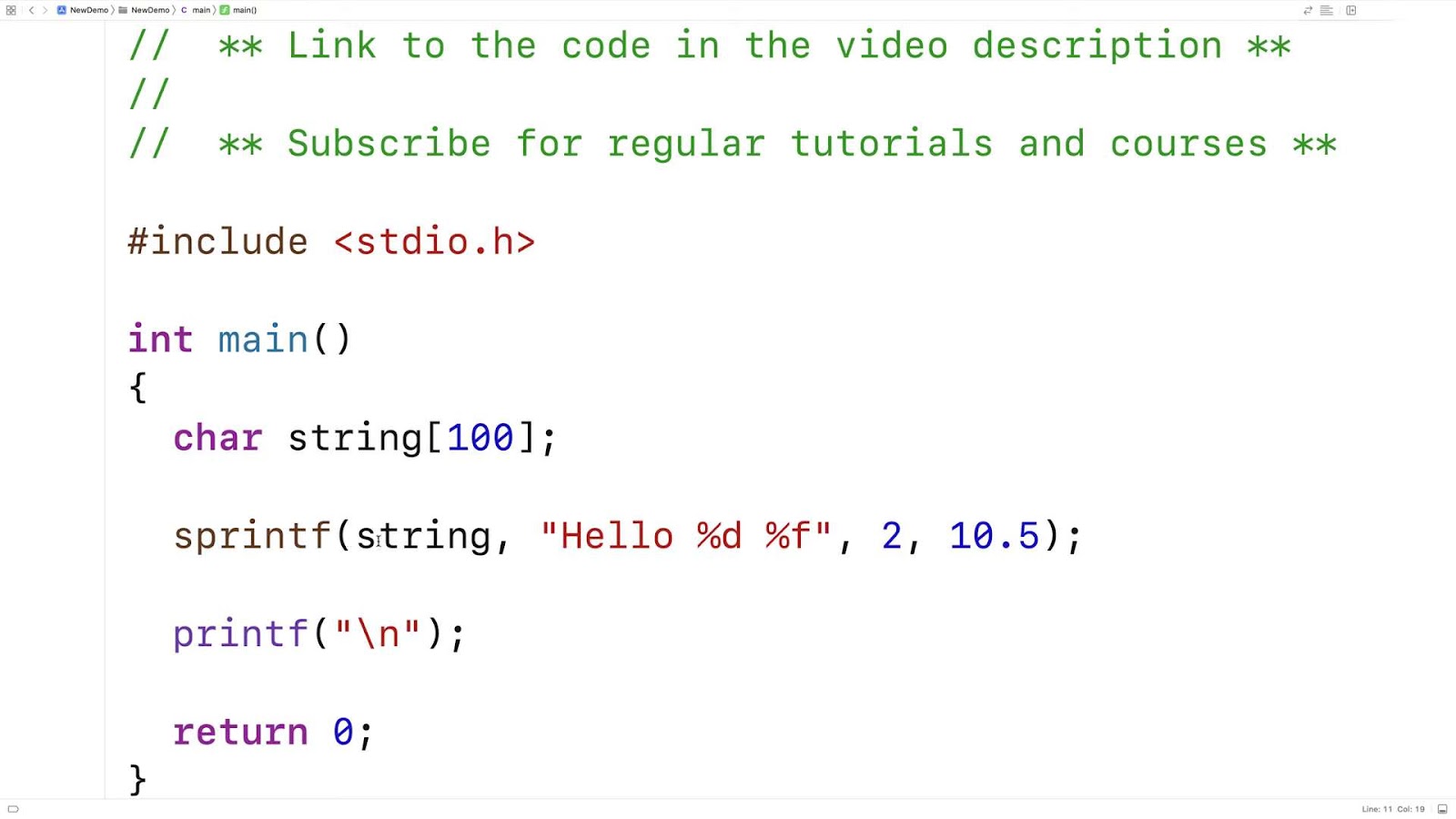
PHP’s sprintf() function stands as a robust utility for manipulating strings with finesse, enabling developers to craft well-structured and easily maintainable code. This versatile function accommodates a plethora of input types and offers an extensive array of formatting possibilities. In the forthcoming tutorial, we shall delve into the fundamentals of the sprintf() function, extol its advantages, and furnish you with illustrative instances to demonstrate its efficacious application within your PHP endeavors.
Delving into the sprintf() Function: A PHP Essential
The sprintf() function in PHP is a versatile tool for developers. It is a built-in function designed to format strings by employing place holders. This incredibly handy feature allows developers to create more dynamic and flexible code. Similar in function to the printf() command, the difference lies in the output. While printf() delivers the result, sprintf() returns the formatted string, providing the flexibility to store it in a variable or use it for additional processing.
The versatility of sprintf() allows developers to manipulate strings, numbers or any other data types within PHP, saving time and simplifying the code. It can be put to use in a myriad of situations such as:
- Formatting text for display;
- Customizing string output;
- Generating specific string formats.
Exploring the Syntax of sprintf()
The structure or syntax of sprintf() function in PHP is as follows:
string sprintf ( string $format [, mixed $arg1 [, mixed $... ]] )
In this syntax, the sprintf() function requires a format string as its primary argument. Following this, a variable number of arguments can be added, designed to substitute the placeholders in the format string. The result is a meticulously formatted string returned by the function.
Understanding the syntax of sprintf() requires knowing that:
- string $format is the initial string which includes placeholders;
- mixed $arg1 represents the first argument to be inserted at the first placeholder in the format string;
- mixed $… is a variable argument list that corresponds to the placeholders within the format string.
Learning how to properly use sprintf() syntax can:
- Reduce potential errors;
- Create cleaner, more efficient code;
- Enhance your PHP coding skills.
Remember, practice is key to mastering this built-in function, leading to better coding proficiency and effectiveness in PHP.
A Detailed Look at the sprintf() Function: A Key PHP Tool
The sprintf() function is an integral part of PHP’s inbuilt tools, playing a vital role in forming strings through the use of placeholders. These placeholders are an embodiment of the variables input by the developer. Echoing the functionality of the printf() function, sprintf() however, deviates in its output methodology. Instead of directly providing the result, it yields a string that has undergone formatting as per the designated input format while being augmented by a set of variables. This formatted string is now at the developer’s disposal to be stored in a different variable or for further processing as required.
Crucially, sprintf() affords developers the liberty to create more dynamic and flexible code, thereby opening avenues for its application in a host of situations. The function’s broad-ranging usage primarily includes:
- Displaying formatted text: It offers an efficient way to rightly format text for display needs;
- Manipulating string output: It provides the capability to customize the string output resulting in controlled and desired string outcomes;
- Generating specific string formats: Its ability to deliver particular string formats makes sprintf() a valuable tool for developers.
Decoding the sprintf() Function Syntax
The sprintf() function in PHP follows a particular syntax, created to accommodate the unique requirements of this function:
string sprintf ( string $format [, mixed $arg1 [, mixed $... ]] )
At a granular level, this syntax entails a format string as its primary argument with a succeeding variable list of arguments to replace the placeholders in the format string. The outcome is a precisely formatted string.
Key elements of the sprintf() syntax include:
- string $format: This is the base string that integrates the placeholders;
- mixed $arg1: This is the inaugural argument meant to replace the first placeholder in the format string;
- mixed $…: This represents a variable argument list designed to replace placeholders in the string.
The sprintf() function’s syntax, when adequately grasped and implemented, can significantly enhance coding efficiency. It minimizes errors, ensures cleaner code, and broadens your PHP skillset. Mastering the sprintf() function can be a game-changer for developers, leading to better coding efficiency and efficacy.
Operating the sprintf() Function
Utilizing the sprintf() function involves the provision of a format string that includes placeholders, followed by their corresponding variables for replacement. The placeholders are encapsulated by a percentage symbol (%) succeeded by a specifier. The specifier’s role is to stipulate the type and formatting preferences of the variable meant to replace the placeholder.
Various specifiers have distinct roles and applications:
- %s: Denotes a String;
- %d: Represents a Signed decimal integer;
- %f: Refers to a Floating-point number;
- %u: Symbolizes an Unsigned decimal integer;
- %o: Stands for an Unsigned octal integer;
- %x: Signifies an Unsigned hexadecimal integer (using lowercase);
- %X: Means an Unsigned hexadecimal integer (in uppercase);
- %c: Marks a Single character;
- %%: Depicts a Literal percent sign.
By understanding and leveraging these specifiers, developers can effectively manipulate outputs to align with their specific needs and requirements. This further exemplifies the power and flexibility of the sprintf() function in PHP.
A Practical Guide to sprintf() Application
To apply sprintf(), it is necessary to deliver a format string that accommodates placeholders, supplemented by respective variables tailored to replace these placeholders. The placeholders are identified by the percentage symbol (%) leading into a specifier that dictates the nature and structuring of the variable set to replace the placeholder.
The sprintf() function provides various specifiers, each serving unique purposes:
- %s: Represents a String;
- %d: Denotes a Signed decimal integer;
- %f: Illustrates a Floating-point number;
- %u: Signifies an Unsigned decimal integer;
- %o: Defines an Unsigned octal integer;
- %x: Refers to an Unsigned hexadecimal integer (lowercase);
- %X: Represents an Unsigned hexadecimal integer (uppercase);
- %c: Marks a Single character;
- %%: Stands for a Literal percent sign.
Understanding the role and functionality of these specifiers allows developers to combine them creatively to generate desired outputs.
Illustrating sprintf() Function: A Practical Example
Exemplifying the application illustrates the prowess of sprintf(). Consider a scenario where there’s a need to introduce a person’s name and age in a formatted manner. The sprintf() function can be a smart way to achieve this:
$name = "John";
$age = 30;
$introduction = sprintf("Hello! Meet %s. He is %d years old.", $name, $age);
echo $introduction; // Output: Hello! Meet John. He is 30 years old.
In this example, %s and %d are placeholders for a string and a decimal integer, respectively. The variables $name and $age provide the respective values for these placeholders. Consequently, sprintf() returns a neatly formatted string which introduces John and mentions his age, creating an effective introduction without any unnecessary concatenation or complex syntax. Understanding practical examples like these can enrich developers’ experience with sprintf(), making it a valuable addition to their PHP toolkit.
A Deeper Dive into sprintf(): A Basic Implementation Example
The sprintf() function proves its usefulness with its enormous adaptability to differing scenarios. Consider an instance when one needs to introduce a person’s name and age accurately. This function is a lucid and straightforward way to get this done:
$individual = "John Doe";
$years = 30;
$introduction = sprintf("Meet %s, who is %d years young.", $individual, $years);
echo $introduction; // Output: Meet John Doe, who is 30 years young.
In this example, the %s and %d placeholders are assigned to a string and a decimal integer, respectively. The variables $individual and $years serve as the corresponding values for these placeholders. The outcome, produced by sprintf(), is a skillfully formatted string introducing John and expressing his age effectively without superfluous concatenations or complicated syntaxes.
This practical demonstration of the sprintf() function encapsulates the ease of its application and its ability to simplify code and boost readability.
Another Illustration: Harnessing sprintf() for Number Formatting
sprintf() also shines when it comes to precision in formatting numbers, particularly when handling monetary values. Consider the following example:
$cost = 1234.56;
$priceTag = sprintf("Total: $%.2f", $cost);
echo $priceTag; // Output: Total: $1234.56
Here, the %.2f specifier is the star of the show. It formats the floating-point number $cost ensuring precision up to two decimal places, and smartly positions a dollar sign before the figure, thus adding a conventional touch to the displayed price.
This characteristic capability of sprintf() makes it instrumental in dealing with financial calculations and displays, thereby adding another feather to its cap. Conclusively, the sprintf() function is indeed a powerful tool in a PHP developer’s arsenal. It exemplifies streamlined coding and precision, rendering it a must-know for coders seeking to excel in PHP.
A Closer Inspection: Implementing Padding and Alignment with sprintf()
The sprintf() function shines in its ability to format strings with specific padding and alignment. By adding specifier details, developers can control the layout of their string outputs to suit their needs accurately.
Consider an array of programming languages that need to be printed with left alignment and padded to ensure a uniform width for each string. This is how sprintf() can make it happen:
$programming_languages = array("PHP", "JavaScript", "Python");
foreach ($programming_languages as $language) {
echo sprintf("%-12s", $language);
}
In this snippet, %-12s is the specifier playing a dual role: it ensures the string $language is left-aligned and also mandates the inclusion of padding such that the minimum width of the string turns out to be 12 characters.
The result is a neatly formatted list of programming languages aligned to the left, each in its space with a uniform width, which enhances readability and visual appeal. This feature can be particularly useful in applications where data needs to be displayed neatly, like in tables or aligned lists.
Mastering the use of padding and alignment with sprintf() opens new avenues for customization and formatting solutions, solidifying its position as an essential tool for PHP developers.
Note: The number 12 used in the “%-12s” specifier can be swapped with any other number to specify the desired character width of the string. The minus sign – implies left-alignment. Remove it to achieve right alignment. Always remember to experiment with different specifiers to discover innovative uses for sprintf().
Unveiling the Advantages of Leveraging sprintf()
The integration of the sprintf() function in PHP code can bring notable benefits to the table, enhancing development proficiency and code quality. Some advantages of using the sprintf() function include:
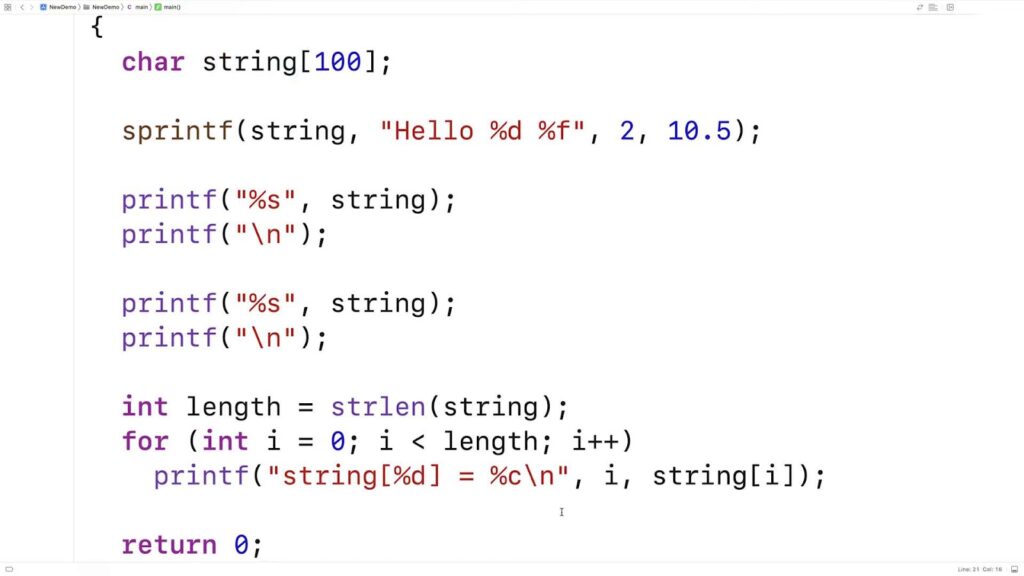
Enhanced Code Clarity:
Incorporating placeholders in a format string refines code clarity and readability. This is particularly advantageous when juggling multiple variables in a string. With sprintf(), placeholders eliminate the need for convoluted concatenation or complex variable placements in the text, thereby creating a cleaner, more comprehensible codebase.
Uniformity in Output Formatting:
sprintf() is a reliable tool for enforcing consistency in output formats. Regardless of the values being processed, a consistent formatting structure can be maintained in the output, simplifying debugging and code maintenance tasks. When the format is predefined, developers can easily diagnose erroneous outputs by comparing them with the expected format.
Localization Efficiency:
The use of placeholders adds a layer of separation between the formatting and the content of a string. This separation becomes beneficial when applications are being localized or translated. The format string, with its placeholders, can remain consistent, while the variables (content) can be replaced as per localization needs.
In addition to these benefits, sprintf() also offers:
- Precision Control: With specifiers like %.2f, developers can control the precision of the numerical values in their outputs, especially beneficial while dealing with financial or mathematical computations;
- Greater Flexibility: The user-defined variable number of arguments feature of sprintf() offers higher adaptability in creating dynamic output formats.
Conclusively, the sprintf() function is not just a feature of PHP; it’s an enabler, boosting coding efficiency, readability, and precision. Whether for simple string formatting needs or complex localization tasks, sprintf() proves to be an invaluable tool in a PHP developer’s repertoire.
Conclusion
The sprintf() function in PHP stands as a robust and adaptable instrument for formatting strings. Its capabilities extend to delivering a streamlined and effective means of structuring strings replete with placeholders, a feature that renders your code more easily comprehensible and maintainable. By mastering and incorporating the sprintf() function, you can elevate the caliber of your PHP endeavors, simplifying the process of recruiting PHP developers who can readily grasp and engage with your codebase.