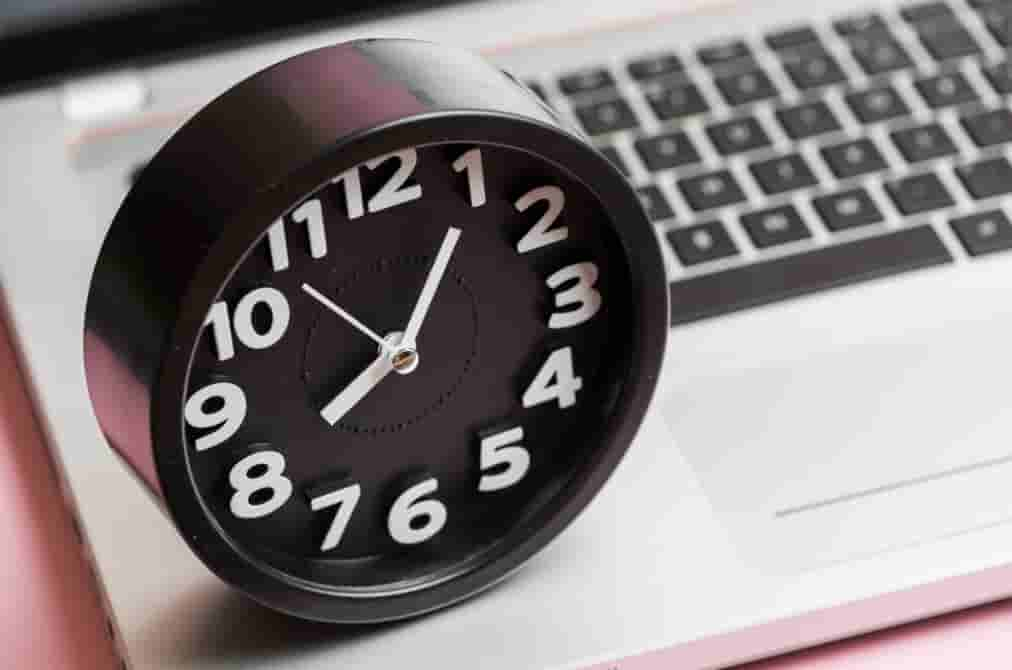
This guide will teach you how to handle date and time in PHP using the DateTime and Timezone classes. It explains the practical steps and functions needed to change, organize, and perform calculations with date and time values.
If you work with spreadsheets in PHP, understanding the DateTime class is essential for effectively managing dates and times. You might also find our article on PHP spreadsheets useful, as it covers how to manipulate and format spreadsheet data using PHP.
DateTime and Timezone classes
- In PHP, the DateTime class provides an object-oriented solution for efficiently managing date and time operations. This class offers comprehensive features such as precise date and time formatting, parsing, and modification capabilities, facilitating tasks such as calculating time differences, generating timestamps, or adapting date strings to specific formats;
- The Timezone class complements the DateTime class by equipping developers with essential tools for handling timezones. Given the global nature of web applications, managing timezones is vital. The Timezone class simplifies this by enabling seamless conversions between different timezones, ensuring accurate representation and processing of time-related data, regardless of user locations. It enhances synchronization in a globally interconnected environment.
Together, the DateTime and Timezone classes serve as crucial components within PHP, delivering essential functionality for controlling date and time operations in web development. Proficiency in these classes is imperative for achieving precise and reliable temporal data management in web applications, ensuring user-friendly and internationally accessible functionality.
Implementation and Initialization of DateTime Objects in PHP
In PHP, the instantiation of DateTime objects is achieved through the use of the DateTime constructor or the static method DateTime::createFromFormat(). This process is essential for representing date and time values in object-oriented programming.
For instance, to instantiate a DateTime object representing the current date and time, the following code can be employed:
$now = new DateTime();
echo $now->format('Y-m-d H:i:s');
Alternatively, to initialize a DateTime object with a specific date and time, a formatted string can be passed to the constructor, as demonstrated here:
$date = new DateTime('2022-01-01 12:00:00');
echo $date->format('Y-m-d H:i:s');
Furthermore, for cases requiring a DateTime object based on a custom format, the DateTime::createFromFormat() method is utilized. This method enables the creation of a DateTime instance from a date string that conforms to a specified format pattern:
$date = DateTime::createFromFormat('d/m/Y H:i', '01/01/2022 12:00');
echo $date->format('Y-m-d H:i:s');
Formatting Date and Time in PHP Using
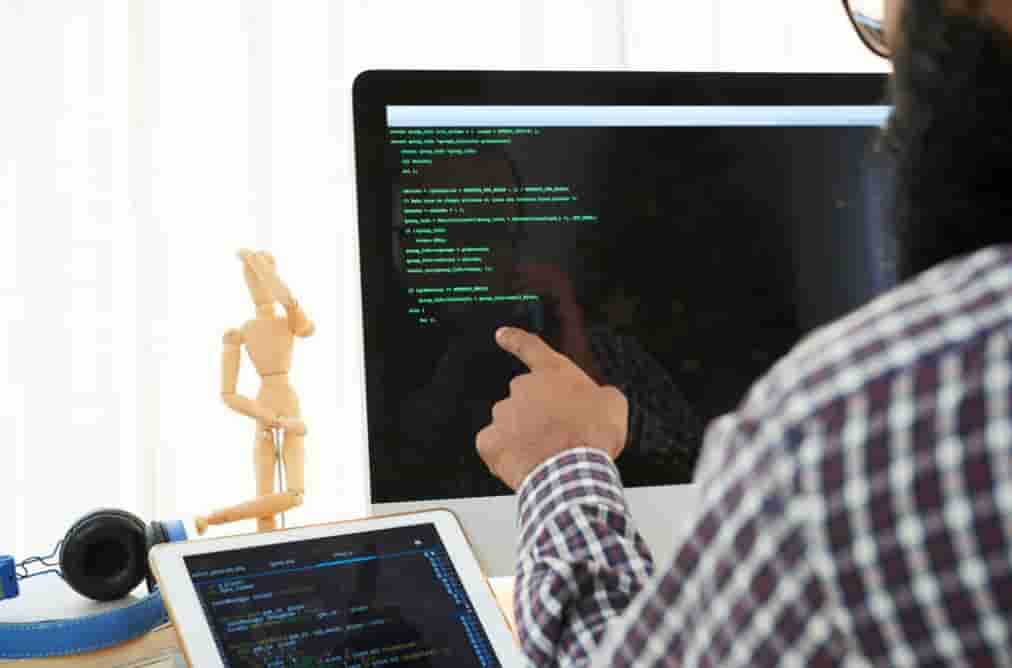
The DateTime::format() method in PHP enables the conversion of DateTime objects into string formats using specific codes. This function is essential for representing date and time data in various formats, as required in different programming contexts.
Formatting Code | Description |
---|---|
Y | Represents a four-digit year (e.g., 2022). |
m | Denotes a two-digit month (e.g., 01). |
d | Specifies a two-digit day (e.g., 31). |
H | Indicates a two-digit hour (00-23). |
i | Represents a two-digit minute (00-59). |
s | Signifies a two-digit second (00-59). |
Here is an illustration of how to convert a DateTime object into a string format:
$date = new DateTime('2022-01-01 12:00:00');
echo $date->format('Y-m-d H:i:s'); // Output: 2022-01-01 12:00:00
PHP Timezone Management with DateTime and DateTimeZone
The PHP DateTime::setTimezone() method facilitates the setting of timezones for DateTime objects. This is complemented by the DateTimeZone class, which enables the creation of Timezone objects using specific timezone identifiers.
For example, to alter the timezone of a DateTime object, one can employ the following code:
$date = new DateTime('2022-01-01 12:00:00', new DateTimeZone('UTC'));
echo $date->format('Y-m-d H:i:s T'); // Output: 2022-01-01 12:00:00 UTC
$newTimezone = new DateTimeZone('America/New_York');
$date->setTimezone($newTimezone);
echo $date->format('Y-m-d H:i:s T'); // Output: 2022-01-01 07:00:00 EST
The DateTimeZone::listIdentifiers() method in PHP is a functional tool that retrieves an array of all supported timezone identifiers. This functionality is critical for maintaining compatibility and precision in applications where time calculations are sensitive to regional differences. The following code demonstrates its usage:
$timezones = DateTimeZone::listIdentifiers();
print_r($timezones);
This method is key in providing a reliable means to manage timezone-related operations in PHP, especially for applications that operate across multiple geographic regions and require accurate time zone handling.
Calculating Date and Time Differences in PHP
In PHP, the DateTime::diff() method is a specialized function designed to compute the difference between two DateTime objects, returning a DateInterval object that encapsulates the duration between the two specified dates.
The following code snippet exemplifies the process of determining the time interval between two distinct dates:
$date1 = new DateTime('2022-01-01');
$date2 = new DateTime('2022-12-31');
$interval = $date1->diff($date2);
echo $interval->format('%y years, %m months, %d days'); // Output: 0 years, 11 months, 30 days
This method is particularly useful in scenarios where precise measurement of the elapsed time between two points is necessary, such as in event scheduling, age calculation, or time-sensitive transaction processing. The DateTime::diff() method thus provides an essential tool for accurate and efficient time difference calculations in PHP programming.
Adjusting DateTime Objects in PHP
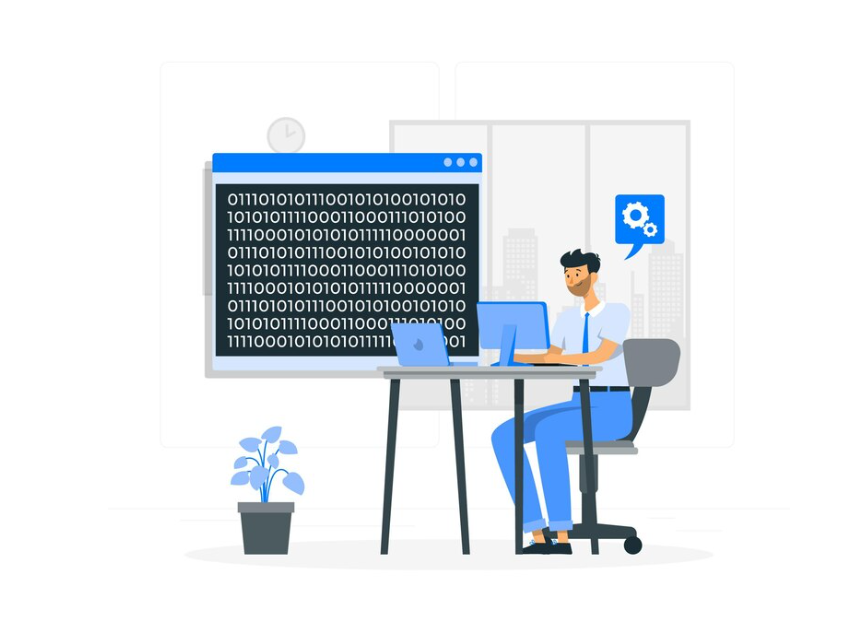
In PHP, the DateTime::modify() method is utilized for altering DateTime objects. This method requires a string parameter that explicitly defines the desired modification.
The following code illustrates how to apply modifications to a DateTime object:
$date = new DateTime('2022-01-01 12:00:00');
echo $date->format('Y-m-d H:i:s'); // Output: 2022-01-01 12:00:00
$date->modify('+1 day');
echo $date->format('Y-m-d H:i:s'); // Output: 2022-01-02 12:00:00
This method is particularly useful for dynamic date and time adjustments in applications, such as scheduling systems or time-based calculations, providing a straightforward approach to manipulating DateTime instances in PHP.
Conclusion
This guide has covered the practical use of PHP’s DateTime and Timezone classes for managing date and time tasks. We’ve discussed various methods and functions for creating, formatting, manipulating, and conducting calculations with date and time data. To become proficient in handling date and time operations in PHP, it’s essential to practice the examples provided here and further explore the DateTime and Timezone classes. This hands-on experience will help you master these tools effectively.