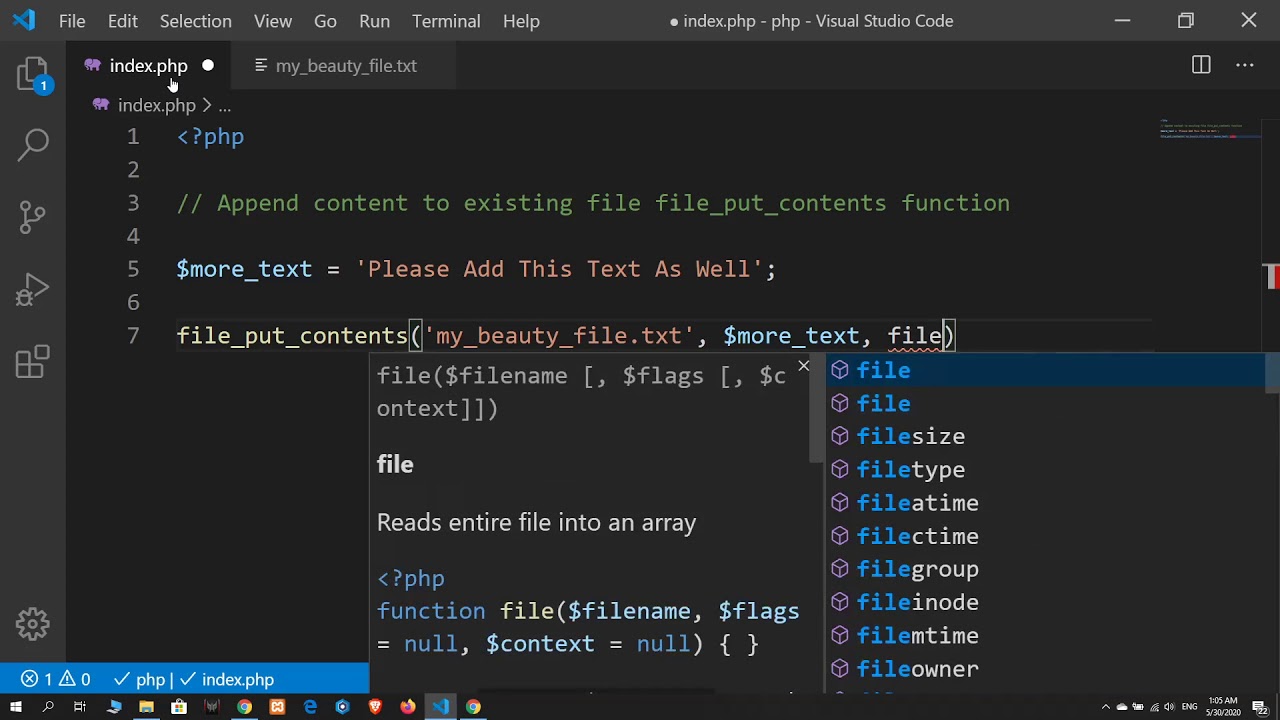
A fundamental task in PHP development is writing data to files. Knowing how to effectively write to files is critical, whether you’re creating logs, generating reports, or storing user data. Enter file_put_contents, a powerful function that simplifies file writing and gives you a great deal of control over the process.
The Essentials of file_put_contents:
At its core, file_put_contents takes two arguments: the filename you want to write to and the data you want to write. It handles opening the file, writing the data, and closing the file, making it a convenient one-stop solution for basic file writing.
Here’s an example of its basic usage:
PHP
$filename = "data.txt";
$data = "This is the content to be written to the file.";
file_put_contents($filename, $data);
Beyond the Basics:
While the basic usage is straightforward, file_put_contents offers a plethora of powerful options:
- Appending content: Use the FILE_APPEND flag to add data to the end of an existing file instead of overwriting it.
PHP
file_put_contents($filename, $data, FILE_APPEND);
- Error handling: Catch potential errors like non-existent directories or permission issues with try-catch blocks.
PHP
try {
file_put_contents($filename, $data);
echo "Data written successfully!";
} catch (Exception $e) {
echo "Error writing to file: " . $e->getMessage();
}
- Locking files: Prevent concurrency issues by using the LOCK_EX flag to lock the file while writing.
PHP
file_put_contents($filename, $data, LOCK_EX);
- Advanced options: Fine-tune behavior with additional flags like FILE_USE_INCLUDE_PATH and context streams.
These options give you granular control over how you write to files, making file_put_contents a versatile tool for diverse requirements.
Real-World Applications
Mastering file_put_contents opens doors to numerous practical applications:
- Logging application activity: Write debug messages or system events to log files for later analysis;
- Generating dynamic content: Create HTML files with custom data for personalized user experiences;
- Exporting data: Save database information to CSV files for easy analysis and sharing;
- Storing user uploads: Securely store files uploaded by users on your website.
These are just a few examples; the possibilities are limited only by your imagination.
Best Practices and Tips
To ensure efficient and secure file writing with file_put_contents:
- Validate user input before writing it to files to prevent data leakage or injection vulnerabilities;
- Check for and create directories if they don’t exist before writing to avoid errors;
- Use error handling to gracefully handle potential issues and provide informative feedback;
- Consider alternative functions like fwrite for scenarios requiring lower-level file manipulation.
Conclusion
file_put_contents is a powerful and versatile tool for handling file writing in PHP. You can effortlessly write files, unlock innovative capabilities, and take your projects to the next level by understanding its core functionality, advanced options, and best practices. So get out there, explore the depths of file_put_contents, and conquer the world of file handling in PHP!