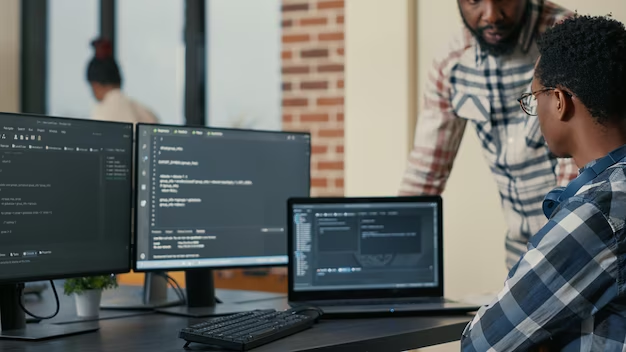
File handling skills are indispensable in software development. PHP, a dynamic and extensively utilized programming language, endows developers with potent file management functions. This article delves into the nuances of PHP file handling, particularly focusing on the functions fopen(), fwrite(), and fclose(), offering valuable insights for both beginners and seasoned developers.
Employing fopen() for File Access
fopen() in PHP is pivotal for opening files or URLs, necessitating the file name (filename) and access mode (mode). The chosen mode determines the file’s accessibility:
- r: Reading from the start;
- r+: Reading and writing from the start;
- w: Writing, truncating, or creating files;
- w+: Reading, writing, and file truncation;
- a: Writing, appending to the file’s end;
- a+: Reading and writing, appending to the end.
Example:
$filename = “example.txt”;$file = fopen($filename, “r”) or die(“Unable to open file!”); |
This code opens “example.txt” in read mode with an error message for failure.
Data Writing with fwrite()
fwrite() in PHP writes data to a file, requiring the file pointer (file), the data (string), and an optional maximum length (length).
Example:
$filename = “example.txt”;$file = fopen($filename, “w”) or die(“Unable to open file!”);$data = “This is sample text.\n”;fwrite($file, $data);fclose($file); |
Here, “example.txt” is opened for writing, data is written, and the file is closed.
Finalizing Operations with fclose()
Using fclose() in PHP is crucial for closing open files, freeing resources, and preventing data issues.
Example:
$filename = “example.txt”;$file = fopen($filename, “r”) or die(“Unable to open file!”);// file operationsfclose($file); |
This demonstrates opening, operating, and closing “example.txt”.
Comparative Analysis: PHP File Functions
Function | Purpose | Parameters | Use Cases |
---|---|---|---|
fopen() | Opens a file or URL | filename (file/URL), mode (access mode) | File reading, writing, appending |
fwrite() | Writes data to a file | file (file pointer), string (data), length (optional) | Data writing, file content modification |
fclose() | Closes an open file | file (file pointer) | Finalizing file operations, resource management |
This table provides a concise comparison of the PHP functions fopen(), fwrite(), and fclose(), highlighting their primary purposes, parameters, and typical use cases. It serves as a quick reference for understanding the distinct roles and applications of each function in PHP file management.
Utilizing usleep() in PHP
The usleep() function is a valuable tool in PHP for introducing microsecond delays in script execution. This can be particularly useful in file handling scenarios where timing is crucial, such as when waiting for a file to be released by another process or implementing retry logic in case of temporary file access issues.
- Syntax of usleep():
usleep(microseconds); |
Here, microseconds is the delay period in microseconds (1 millionth of a second).
- Example of using usleep() in file handling:
$filename = “example.txt”;$file = fopen($filename, “w”) or die(“Unable to open file!”);$data = “Sample text.\n”;fwrite($file, $data);usleep(100000); // Delay for 100 millisecondsfclose($file); |
Conclusion
This tutorial has comprehensively covered the key PHP file handling functions: fopen(), fwrite(), and fclose(). These functions form the cornerstone of effective and reliable file management in PHP development. Mastery of these tools is crucial for any PHP developer seeking to create robust applications. To further advance your skillset, consider exploring additional PHP file-handling functions such as fread(), fgets(), and file_put_contents().