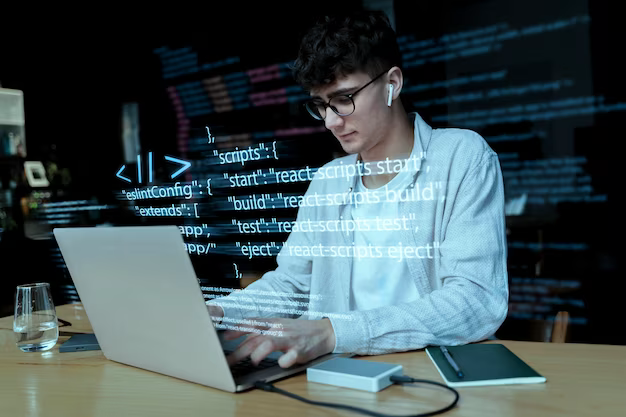
In this guide, we’ll explore the implementation of JWT (JSON Web Token) authentication in PHP-based applications. JWT offers a stateless and secure approach to user authentication, a method commonly employed in contemporary web apps. We’ll delve into the fundamentals of JWT, the process of generating and validating JWT tokens in PHP, and share some recommended practices for integrating JWT authentication into your PHP projects.
Demystifying JWT Authentication
JWT Authentication, or JSON Web Tokens, is an open standard (RFC 7519) that provides a secure and compact pathway for the exchange of information among parties encapsulated in a JSON object. This method of authentication is stateless and operates on a token-based system, alleviating the need for servers to hoard session details about the user.
The server is responsible for producing a JWT token composed of information related to the user, paired with a unique signature. The client receives this token and sends it back to the server during subsequent requests to access safeguarded resources. The server’s task is to validate the legitimacy of this token.
Each JWT token is a triad of crucial parts:
1. Header: It holds the token type (JWT) and the signing algorithm employed;
2. Payload: This section contains the claims that include data related to the user and supplementary metadata like the time of expiration;
3. Signature: A fusion of the header, payload, and a private key utilized to affirm the token’s authenticity.
These components, when Base64Url encoded, combined with a period separator (‘.’), and signed with a secret key, take the form of the JWT token.
Before You Begin
To follow along with this tutorial, make sure your system has PHP 7.0 or a higher version installed. Familiarity with the principles of PHP programming is also essential. Additionally, you will need the following PHP libraries:
- firebase/php-jwt: This PHP library allows seamless encoding and decoding of JSON Web Tokens;
- slim/slim: It is a lean PHP micro-framework that aids in developing RESTful APIs and web applications.
You can use Composer to install these libraries with the following commands:
composer require firebase/php-jwt
composer require slim/slim
Creating and Validating JWT Tokens in PHP
First, let’s create a function to generate a JWT token for a user. This function will take the user’s ID and a secret key as input, and return a signed JWT token containing the user’s ID and an expiration time.
use Firebase\JWT\JWT;
function carve_jwt_token($user_id, $private_key) {
$timestamp = time();
$expiry = $timestamp + (60 * 60); // token valid for 1 hour
$data = array(
'iat' => $timestamp,
'exp' => $expiry,
'sub' => $user_id
);
return JWT::encode($data, $private_key);
}
For our subsequent step, we will engineer a function designed to validate and decode a JWT token. This function requires a JWT token and a secret key as input and renders the decoded data payload given that the token stands valid.
use Firebase\JWT\ExpiredException;
use Firebase\JWT\SignatureInvalidException;
use Firebase\JWT\BeforeValidException;
use Firebase\JWT\JWT;
function authenticate_jwt_token($jwt_token, $secret_key) {
try {
return JWT::decode($jwt_token, $secret_key, array('HS256'));
} catch (ExpiredException $e) {
throw new Exception('Token has expired');
} catch (SignatureInvalidException $e) {
throw new Exception('Token signature does not match');
} catch (BeforeValidException $e) {
throw new Exception('Token not yet valid');
} catch (Exception $e) {
throw new Exception('Invalid token');
}
}
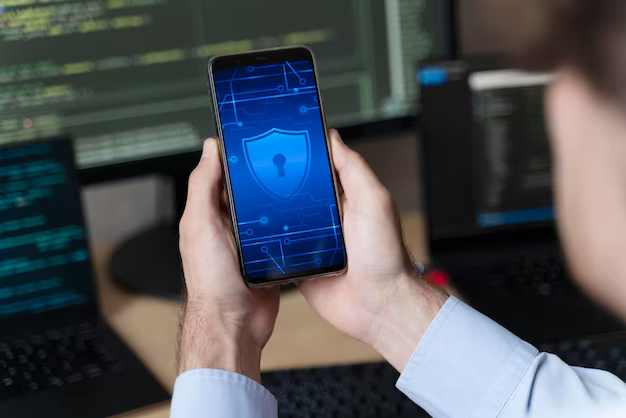
Implementing JWT Authentication in a PHP Application
Now that we have our JWT functions, let’s implement JWT authentication in a PHP application using the Slim framework. Here is a basic application structure:
- composer.json;
- composer.lock;
- index.php;
- vendor/.
In this structure, we will construct a rudimentary RESTful API. This API will provide three separate routes:
- /login: This function verifies the user, providing JWT token upon success;
- /protected: This function gives access to a resource that is only available to users with a valid JWT token;
- /public: This function gives access to a public resource, which can be accessed without a JWT token.
At the onset, it is crucial to import the relevant libraries and establish the Slim application. The necessary code for this step is:
require 'vendor/autoload.php';
use Slim\App;
$app = new App();
Following this, we need to create the /login route. Typically, under actual circumstances, a database or an alternate user storage system would be used to validate the user’s credentials. However, for the ease of understanding, it will be assumed that the user authentication is always successful and a JWT token would be returned:
use Psr\Http\Message\ResponseInterface as Response;
use Psr\Http\Message\ServerRequestInterface as Request;
$app->post('/login', function (Request $request, Response $response) {
$user_id = 1; // assuming the user is authenticated
$secret_key = 'your_secret_key';
$jwt_token = generate_jwt_token($user_id, $secret_key);
$response_data = array('token' => $jwt_token);
$response->getBody()->write(json_encode($response_data));
return $response->withHeader('Content-Type', 'application/json');
});
Subsequently, let’s create the /protected route. This route’s purpose is to require a valid JWT token in the Authorization header:
$app->get('/protected', function (Request $request, Response $response) {
$auth_header = $request->getHeader('Authorization');
if (empty($auth_header)) {
return $response->withStatus(401)->withHeader('Content-Type', 'application/json')->write('Unauthorized');
}
$jwt_token = str_replace('Bearer ', '', $auth_header[0]);
$secret_key = 'your_secret_key';
try {
$decoded_payload = validate_jwt_token($jwt_token, $secret_key);
$user_id = $decoded_payload->sub;
// Fetch the protected resource for the user
$resource = 'Protected resource for user ' . $user_id;
return $response->withHeader('Content-Type', 'application/json')->write($resource);
} catch (Exception $e) {
return $response->withStatus(401)->withHeader('Content-Type', 'application/json')->write('Unauthorized');
}
});
Lastly, we need to set up the /public route. This route can be accessed without a JWT token:
$app->get('/public', function (Request $request, Response $response) {
return $response->withHeader('Content-Type', 'application/json')->write('Public resource');
});
Once all the routes are established, we can run the Slim app:
$app->run();
Incorporating String Manipulation in PHP
String manipulation plays a pivotal role in PHP programming, complementing the JWT authentication framework discussed in this guide. PHP offers a plethora of functions and methods for manipulating strings, such as concatenation, substring extraction, and regular expression matching. Effective string manipulation is essential when handling user input, formatting data, or interacting with external APIs, enhancing the versatility and robustness of your PHP applications.
Summary
This guide has shown us the steps to integrate JWT authentication into PHP applications, utilizing the firebase/php-jwt library and Slim framework. We’ve seen that JWT offers a reliable, stateless way to authenticate users, fitting well with contemporary web apps and APIs. To elevate the security and functionality of your PHP applications, you might think about adding more security features like role-based access control and rate limiting. For the development of secure and efficient PHP applications, it’s beneficial to engage PHP developers who are well-versed in current web development techniques and security measures.