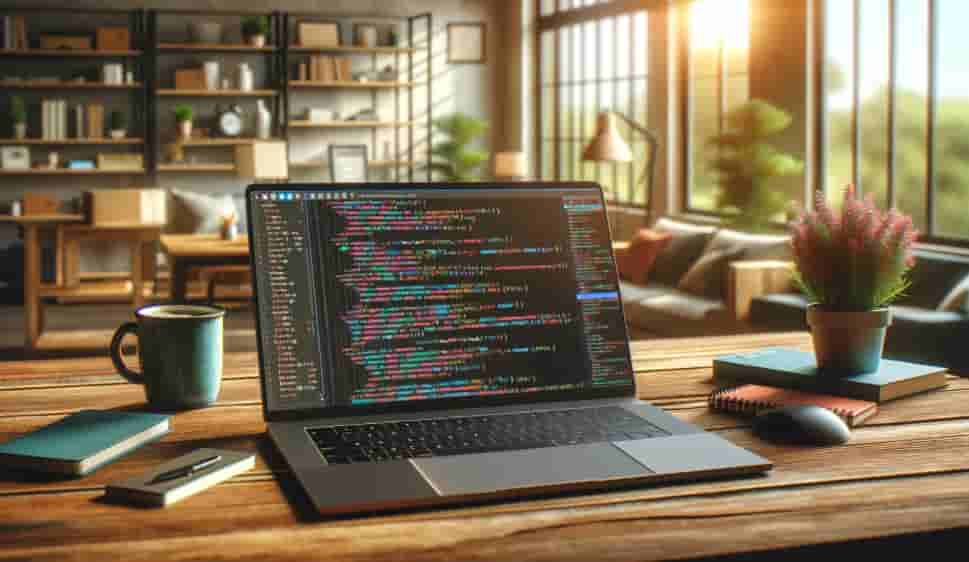
This guide aims to demystify the Standard PHP Library (SPL), a robust set of interfaces and classes embedded within PHP to tackle frequent programming challenges. SPL is a treasure trove of data structures, iterators, and classes for exception and file handling, enhancing both the efficiency and readability of PHP code. A significant advantage is its inclusion in PHP’s core, eliminating the need for additional installations.
Overview of SPL
At its core, the Standard PHP Library (SPL) is an integral part of PHP, offering a plethora of tools designed for efficient problem-solving in programming. The SPL’s arsenal is diverse, encompassing a wide range of data structures, iterators, and specialized classes for managing exceptions and files, thus standing as a testament to its versatility and importance in the realm of PHP development.
Key elements of SPL include:
- – **Data Structures**: SPL is renowned for its rich set of data structures. This includes familiar constructs like arrays, but also extends to more complex types such as linked lists, queues, stacks, and heaps. These structures are optimized for various operations, allowing developers to choose the most efficient one for their specific needs;
- – **Iterators**: SPL revolutionizes data traversal with its iterators. These tools provide a uniform way to cycle through elements of different data structures, thus abstracting the complexities of each structure. Iterators like `ArrayIterator`, `DirectoryIterator`, and more specialized ones like `RecursiveIteratorIterator`, make data handling more intuitive and less error-prone;
- – **Exception Handling Classes**: The library offers a comprehensive set of classes for exception handling, including but not limited to `BadFunctionCallException`, `InvalidArgumentException`, and `RuntimeException`. These classes enable developers to handle errors more precisely and create more robust, error-resistant applications;
- – **File Handling Classes**: SPL enhances file and directory operations through classes like `SplFileInfo`, `SplFileObject`, and `DirectoryIterator`. These classes provide an object-oriented approach to file handling, making operations like file reading, writing, and meta-information retrieval more streamlined and efficient.
By delving into these features, developers can leverage the full power of SPL to create efficient, robust, and maintainable PHP applications. Whether it’s optimizing data handling with advanced structures, managing files and directories more effectively, or writing error-resistant code through precise exception handling, SPL emerges as a cornerstone in the toolkit of any PHP programmer. The beauty of SPL lies in its integration with PHP’s core, offering seamless accessibility and a high degree of compatibility with existing PHP codebases.
Exploring Data Structures
SPL enhances PHP’s capability with several data structures, outperforming traditional PHP arrays in certain scenarios.
SPLFixedArray
For instance, SPLFixedArray is a memory-efficient alternative for large, fixed-size arrays. Here’s an example:
```php
$array = new SPLFixedArray(5);
$array[0] = 'A'; // and so on for other elements
echo $array->getSize(); // and a loop to display array elements
```
SPLDoublyLinkedList
Another example is SPLDoublyLinkedList, ideal for frequent list insertions and deletions due to its lower complexity compared to arrays.
```php
$list = new SPLDoublyLinkedList();
$list->push('A'); // and more elements
echo $list->pop(); // and operations to manipulate and traverse the list
```
Understanding Iterators
SPL offers a suite of iterators for efficient data structure traversal and manipulation.
ArrayIterator
ArrayIterator, for example, provides a more flexible way to traverse and modify arrays and objects compared to the traditional foreach loop.
```php
$array = array('A', 'B', 'C');
$iterator = new ArrayIterator($array);
// Code to modify and display the iterator
```
FilterIterator
Furthermore, FilterIterator, an abstract class, allows for the creation of custom iterator filters.
```php
$numbers = new ArrayIterator(array(1, 2, 3, 4, 5, 6, 7, 8, 9));
// Code for EvenFilterIterator and iteration example
```
Mastering Exception Handling
SPL’s array of exception handling classes aids in catching and managing specific error types, promoting robust and clear code. Some common classes include BadFunctionCallException, InvalidArgumentException, and RuntimeException, among others. Here’s how InvalidArgumentException is used:
```php
// Example of a divide function using InvalidArgumentException
```
Navigating File Handling
SPL enhances PHP’s capabilities with its object-oriented approach to file and directory management, significantly simplifying and streamlining these operations. Key classes like `SplFileInfo` and `DirectoryIterator` stand out for their utility and ease of use. `SplFileInfo` provides a high-level interface to file information, allowing developers to query and manipulate file metadata effortlessly. It simplifies tasks like checking file types, sizes, and modification dates, making file handling more intuitive.
DirectoryIterator
`DirectoryIterator`, on the other hand, is a powerful tool for traversing directory contents. It allows developers to iterate over files and directories within a specified path with ease, as shown in the provided code snippet. This class eliminates the need for cumbersome and error-prone directory traversal using traditional PHP functions. It offers methods to easily assess file attributes and perform operations based on them, such as filtering files, calculating directory sizes, or even performing bulk operations.
With these classes, developers can write more elegant and maintainable file management code. They enable handling complex file operations with fewer lines of code and greater reliability. By leveraging these SPL classes, PHP developers can focus more on the logic and functionality of their applications, rather than the intricacies of file system handling, thus boosting productivity and code quality.
Summing Up
This guide has offered an in-depth exploration of the Standard PHP Library (SPL), emphasizing its critical role in crafting more efficient and maintainable PHP code. The utilization of SPL’s diverse and robust features can lead to a remarkable enhancement in both the performance and quality of PHP applications. SPL’s comprehensive collection of data structures empowers developers to handle complex data more effectively. Its iterators simplify the process of traversing and manipulating data, making code more readable and less prone to errors. Moreover, SPL’s advanced exception handling classes enable more precise and granular error management, fostering the development of robust applications that can handle unexpected scenarios gracefully.
The file handling classes in SPL provide a more sophisticated and object-oriented approach to file operations, paving the way for more secure and reliable file manipulation. This versatility makes SPL an invaluable resource for PHP developers, allowing for more streamlined and optimized coding practices. By integrating SPL effectively into PHP projects, developers can achieve high-performance applications with cleaner, more organized, and scalable code. In essence, SPL is not just a library; it’s a fundamental enhancement to the PHP language, offering tools and functionalities that propel PHP development into a realm of heightened efficiency and sophistication.