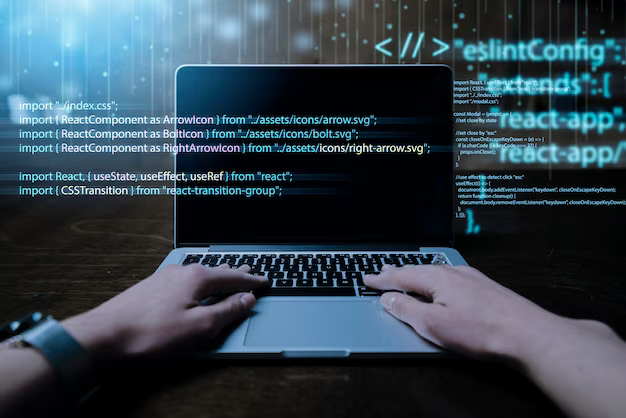
PHP is a powerful scripting language particularly suited for web development. Combining it with the MVC design pattern can yield remarkable results. This comprehensive guide outlines how to build an MVC framework from scratch using PHP. However, remember that applying MVC in PHP web development requires a robust understanding of both PHP and the MVC pattern.
One cannot emphasize enough how a solid comprehension of the MVC framework can skyrocket your value as a PHP developer or even if you aim to hire PHP developers. Mastery over MVC lends you an edge as it not only reflects in the quality of your work, but it also equips you with the knowledge to appreciate the architecture of popular PHP frameworks like Laravel and CodeIgniter.
Prerequisites
Before immersing into this instructive guide, it’s essential to equip yourself with some foundational knowledge. A rudimentary understanding of PHP and its object-oriented programming components is crucial. Additionally, getting acquainted with the PHP package manager, Composer, will certainly be of great assistance.
Step 1: Project Initialization
Kick-start your project by establishing a new directory for it and navigating to it via the terminal:
mkdir php-mvc-framework
cd php-mvc-framework
Next, initialize a new Composer project:
composer init
The system will guide you with a series of prompts to set your project parameters. You may leave the sections asking for dependencies blank at this juncture.
Step 2: Structuring the Directory
A well-organized directory structure is crucial for streamlined project management. For your project, execute the following structure:
src/
Controllers/
Models/
Views/
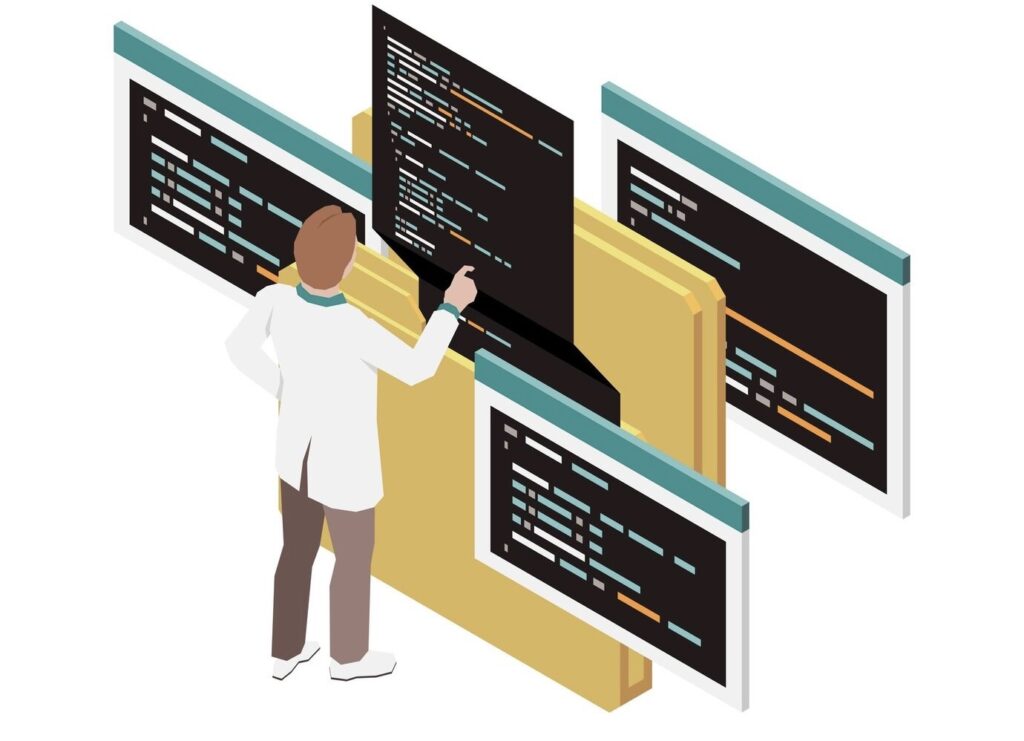
Step 3: Construction of Core Elements
Router
Start by crafting a new file titled ‘Router.php’ in the ‘src/’ directory. This crucial file will harbor the main routing logic of your framework.
<?php
namespace MVC;
class Router {
protected $routes = [];
public function addRoute($route, $controller, $action) {
$this->routes[$route] = ['controller' => $controller, 'action' => $action];
}
public function dispatch($uri) {
if (array_key_exists($uri, $this->routes)) {
$controller = $this->routes[$uri]['controller'];
$action = $this->routes[$uri]['action'];
$controller = new $controller();
$controller->$action();
} else {
throw new \Exception("No route found for URI: $uri");
}
}
}
Base Controller
Following that, generate a new file named ‘Controller.php’ in the ‘src/’ directory. This file will house the base controller class, which all other controllers will extend.
<?php
namespace MVC;
class Controller {
protected function render($view, $data = []) {
extract($data);
include "Views/$view.php";
}
}
Step 4: Implementing a Sample Application
Model Creation
To begin, construct a new file termed ‘User.php’ in the ‘src/Models/’ directory. This model will symbolize a user within your application.
<?php
namespace MVC\Models;
class User {
public $name;
public $email;
public function __construct($name, $email) {
$this->name = $name;
$this->email = $email;
}
}
Controller Creation
Following this, generate a new file titled ‘UserController.php’ in the ‘src/Controllers/’ directory. This controller will regulate user-related functions.
<?php
namespace MVC\Controllers;
use MVC\Controller;
use MVC\Models\User;
class UserController extends Controller {
public function index() {
$users = [
new User('John Doe', '[email protected]'),
new User('Jane Doe', '[email protected]')
];
$this->render('user/index', ['users' => $users]);
}
}
View Creation
Then, develop a new file named ‘index.php’ in the ‘src/Views/user/’ directory. This view will exhibit a list of users.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>User List</title>
</head>
<body>
<h1>User List</h1>
<ul>
<?php foreach ($users as $user): ?>
<li><?= $user->name ?> (<?= $user->email ?>)</li>
<?php endforeach; ?>
</ul>
</body>
</html>
Routing Configuration
Craft another new file titled ‘routes.php’ in the ‘src/’ directory. This file will define your application’s routing system.
<?php
use MVC\Router;
use MVC\Controllers\UserController;
$router = new Router();
$router->addRoute('/', UserController::class, 'index');
Application Testing
Lastly, create a new file named ‘index.php’ in your project’s root directory. This file will act as the gateway to your application.
<?php
require 'vendor/autoload.php';
$uri = $_SERVER['REQUEST_URI'];
$router = require 'src/routes.php';
$router->dispatch($uri);
For application testing, run the built-in PHP web server:
php -S localhost:8000
Visit http://localhost:8000 in your preferred web browser to view the list of users.
PHP Array Filter
In PHP web development, particularly within MVC, the “PHP array filter” function is essential. This function is crucial for filtering and manipulating data arrays, becoming an indispensable tool for developers.
The PHP array filter enables developers to efficiently sort through arrays, selecting elements that fulfill certain criteria. This is particularly useful when handling large datasets or extracting specific data from an array.
Conclusion
Following this in-depth tutorial will assist you in enhancing your PHP skills and your understanding of the MVC design pattern. MVC’s ability to separate the application logic, data, and presentation makes it a favorite amongst developers. With a solid understanding of MVC frameworks, you can streamline your projects, making them more efficient and scalable.