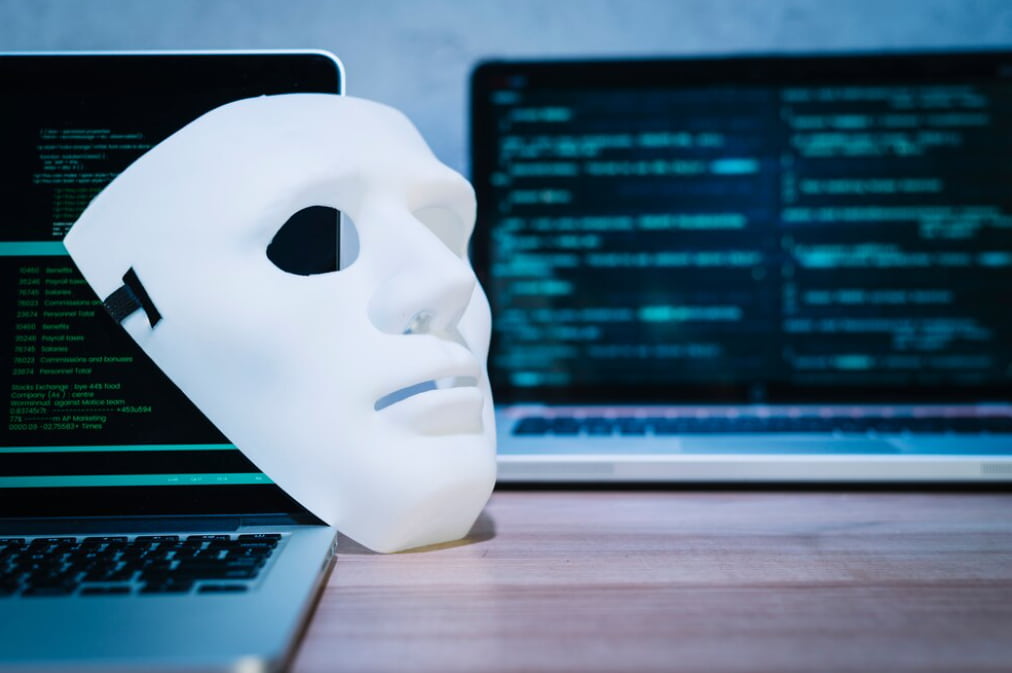
In this tutorial, we’ll take a closer look at PHP closures, specifically anonymous functions and variable scoping. PHP closures are a valuable feature that allows developers to create versatile and functional code. By the end of this tutorial, you’ll have a solid understanding of closures and how to use them effectively in your projects.
While discussing the theme of PHP Anonymous Functions, it’s essential to consider their practical applications, such as those within datetime manipulation in PHP.
Closures in PHP: Anonymous Functions and Practical Usage
Closures, in programming, represent functions that encapsulate and retain their surrounding context, including any variables provided to them. In PHP, these closures are realized through anonymous functions, that lack a formal name. This feature endows it with unique capabilities, enabling it to be treated as variables, passed as arguments, or returned as values from other functions.
- Closures are particularly advantageous in scenarios where you need to create reusable and self-contained blocks of code;
- They offer benefits like encapsulation of variables, enabling cleaner and more modular code;
- Additionally, closures facilitate the creation of callback functions, making them integral in scenarios such as event handling, asynchronous programming, and more.
Anonymous Functions in PHP
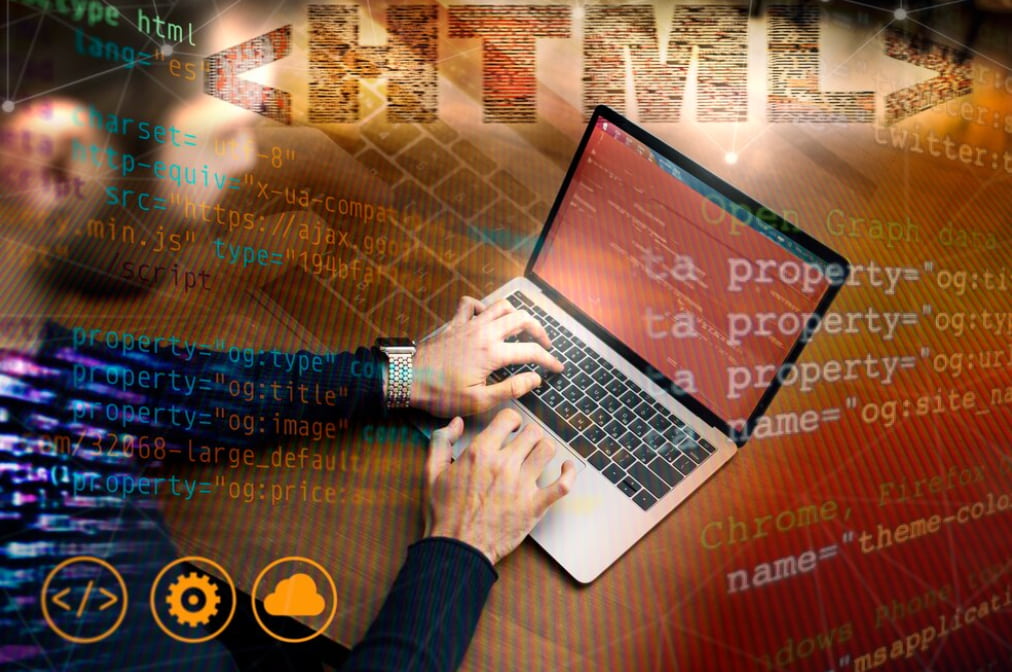
Anonymous functions, frequently known as lambda functions, stand as a cornerstone within PHP programming. Their distinctive attribute is their lack of a formal name, which makes them particularly handy for one-off or specialized tasks. They are constructed using the ‘function’ keyword, accompanied by a parameter list and a function body, encapsulating a set of instructions to be executed. The syntax for creating an anonymous function follows this pattern:
$variable = function($parameter) {
// function body
};
Here’s a straightforward example of an anonymous function that adds two numbers together:
$add = function($a, $b) {
return $a + $b;
};
echo $add(1, 2); // Output: 3
In this example, an anonymous code block takes in two parameters, $a and $b, and performs the addition operation on them, returning their sum. This code block is assigned to a variable named $add, which enables you to execute the code block as if it were a regular callable entity in PHP.
It serves various purposes in PHP programming. They are commonly used as callback functions for sorting arrays, applying filters, or handling events. Additionally, they are valuable when you need to create small, self-contained code blocks for specific tasks, enhancing code modularity and maintainability.
Variable Scoping in PHP
In PHP, variables declared within a function usually possess local scope, which confines their usage exclusively to that particular function. Nevertheless, closures offer a mechanism for accessing variables from the parent scope by utilizing the ‘use’ keyword. To provide a concrete demonstration of this concept, consider the following practical example:
$message = "Hello, world!";
$sayHello = function() use ($message) {
echo $message;
};
$sayHello(); // Output: Hello, world!
The variable $message is defined in the parent scope. By employing the ‘use’ keyword within the closure, we gain the ability to access this external variable. When the $sayHello function is invoked, it successfully outputs the value stored in the $message variable.
Understanding variable scoping and the use of closures in PHP is crucial for writing efficient and maintainable code. It enables developers to create modular and reusable code by selectively accessing variables from higher scopes when needed. This discussion will delve deeper into variable scoping concepts, including local and parent scope, and explore various practical scenarios where closures are beneficial for variable access and manipulation.
Practical Usage of Closures in PHP: Illustrative Examples
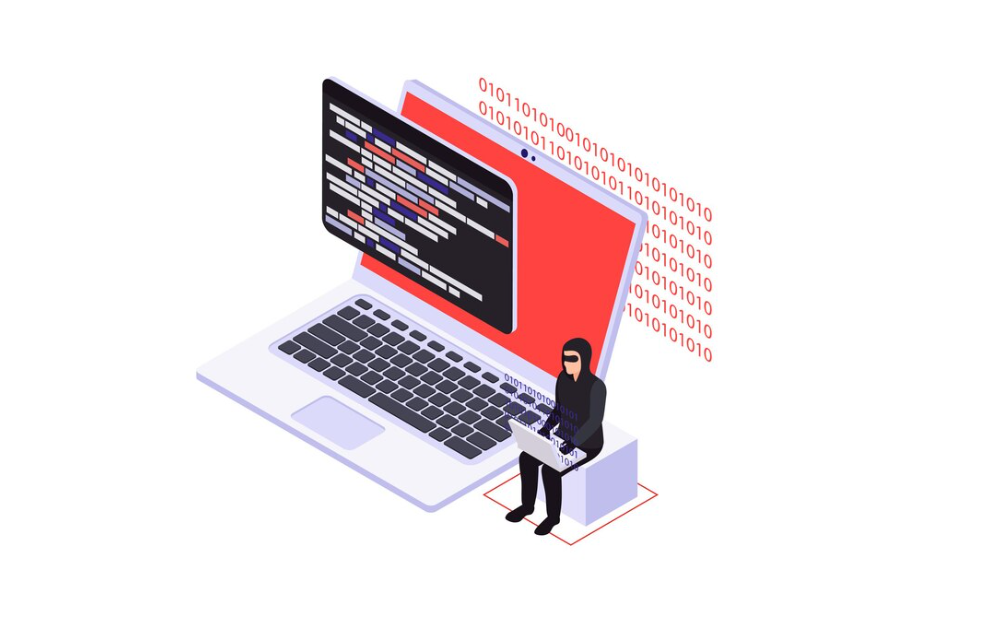
Having gained a foundational grasp of closures, it’s time to delve into concrete instances that showcase their utility in PHP. Explore the following practical examples to better understand how closures can be effectively employed in PHP programming.
Example 1: Array Filtering Using Closures
In this example, a closure is employed to perform array filtering, specifically extracting only the even numbers from an array. Here’s the code snippet that demonstrates this functionality:
$numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9];
$evenNumbers = array_filter($numbers, function($number) {
return $number % 2 == 0;
});
print_r($evenNumbers); // Output: Array ( [1] => 2 [3] => 4 [5] => 6 [7] => 8 )
The array_filter function is utilized here, taking an array and a callback function as its arguments. In this instance, we provide an anonymous function that checks whether a number is even or not. Subsequently, the array_filter function returns a new array containing exclusively the even numbers from the original input array.
Example 2: Array Sorting Using Closures
In this example, a closure is employed to perform the alphabetical sorting of an array of names. Here’s the code snippet that demonstrates this functionality:
$names = ["Alice", "Bob", "Charlie", "David"];
usort($names, function($a, $b) {
return strcmp($a, $b);
});
print_r($names); // Output: Array ( [0] => Alice [1] => Bob [2] => Charlie [3] => David )
The usort function is utilized here, requiring both an array and a comparison function as arguments. In this scenario, we provide an anonymous function responsible for comparing two strings using the strcmp function. Consequently, the usort function arranges the array elements based on the logic defined within the comparison function.
Conclusion
This tutorial delved into the intricacies of closures within PHP, with a specific focus on anonymous functions and variable scoping. In this exploration, we’ve delved into the construction of anonymous code blocks, delved into the mechanism for retrieving variables from the enclosing scope, and presented concrete examples of their utilization. Closures stand out as a potent and essential aspect of PHP, furnishing developers with the capacity to craft adaptable and highly functional code, thus enhancing their programming capabilities.