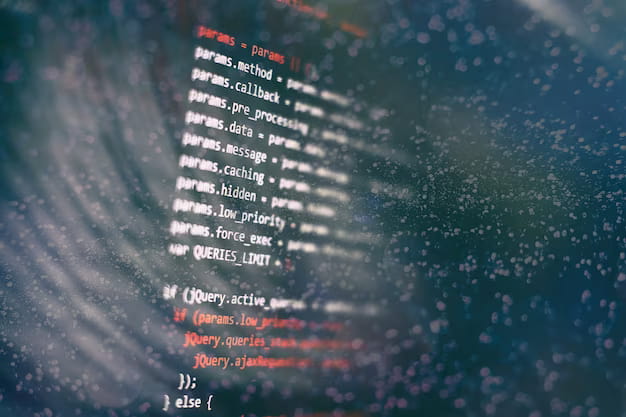
This comprehensive guide will delve deep into one of PHP’s most potent functionalities – the array_filter() function. Readers will explore how this function can be mastered and leveraged to its full potential, particularly for array filtering tasks.
With this in-depth exploration, readers should gain essential insights into:
- Grasping the fundamental application of the array_filter() function;
- Creating personalized callback functions for sophisticated filtering operations;
- Applying the array_filter() function to sieve arrays using keys;
- Melding array_filter() along with other array operations for intricate tasks.
More explicitly, we will showcase how the array_filter() function operates, provide a step-by-step tutorial on its use, and reveal how to tune this function to perform complex and tailored tasks.
Basic Usage of array_filter()
The array_filter() function in PHP comprises the power to sieve elements of an array based on a particular criteria defined in a callback function. For each element in the input array, the function applies the callback function. When the callback function returns true, the element is retained in the output array. If it returns false, the element gets excluded.
The elementary syntax for using array_filter() would be:
$filtered_array = array_filter($original_array, $callback_function);
Let’s delve into an example to filter out all the odd numbers from an array:
$numbers_sequence = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
$filtered_odd_numbers = array_filter($numbers_sequence, function($number) {
return $number % 2 === 1;
});
print_r($filtered_odd_numbers);
The output for the above code would be:
Array
(
[0] => 1
[2] => 3
[4] => 5
[6] => 7
[8] => 9
)
The array_filter() function has indeed only retained the odd numbers, demonstrating its basic functionality. The array keys from the original array are also preserved, which is why the keys are not in a continuous sequence.
Employing Custom Callback Functions with array_filter()
The array_filter() function exhibits its true versatility when paired with custom callback functions, enabling data filtration based on intricate conditions. This approach becomes particularly useful when presented with complex array structures or needing to filter under specific conditions.
Let’s evaluate a scenario where an array of individuals’ data is present, and our task is to extract only those who are at least 18 years old.
$individuals = [
['fullName' => 'Alice', 'age' => 30],
['fullName' => 'Bob', 'age' => 15],
['fullName' => 'Carol', 'age' => 20],
['fullName' => 'Dave', 'age' => 10],
];
$valid_age_individuals = array_filter($individuals, function($person) {
return $person['age'] >= 18;
});
print_r($valid_age_individuals);
In the code above, the $valid_age_individuals array contains only the individuals who satisfy the condition set within the custom callback function.
Running this code will produce the following output:
Array
(
[0] => Array
(
[fullName] => Alice
[age] => 30
)
[2] => Array
(
[fullName] => Carol
[age] => 20
)
)
In the output above, it is evident that the array_filter() function, coupled with a custom callback function, has successfully filtered out individuals below 18 years of age.
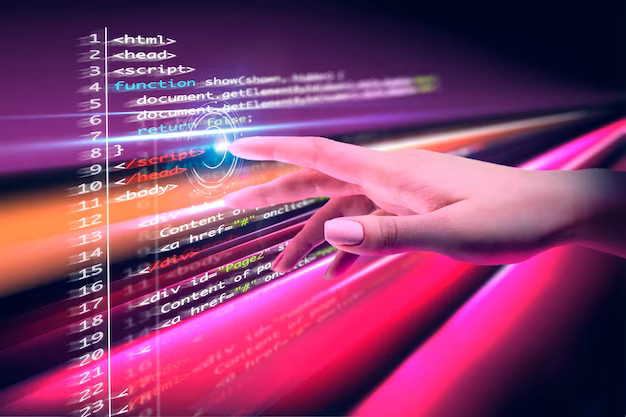
Filtering by Keys
PHP’s array_filter() function typically focuses on array values when performing filtration. Nonetheless, a handy feature allows developers to shift the focus to the keys, thanks to the ARRAY_FILTER_USE_KEY constant.
Here’s a quick demonstration of how to exploit this feature:
$fruits = [
'apple' => 1,
'banana' => 2,
'cherry' => 3,
];
$key_filtered = array_filter($fruits, function($key) {
return strlen($key) > 5;
}, ARRAY_FILTER_USE_KEY);
print_r($key_filtered);
In the example above, an array of fruits is subjected to the array_filter() function. A callback function checks the length of keys and filters out those that have a length of less than or equal to five characters. The ARRAY_FILTER_USE_KEY constant directs array_filter() to apply the callback function to the keys rather than the values.
Array
(
[banana] => 2
)
Combining `array_filter()` with Other Array Functions
Among the multiple facets of the array_filter() function, harmonizing it with other array functions for more intricate operations is undoubtedly an efficient way to enhance its functionality.
Let’s dwell on a situation where we want to employ array_map() to alter the filtered elements once they pass through the array_filter() function.
$number_sequence = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
$odd_numbers = array_filter($number_sequence, function($number) {
return $number % 2 !== 0;
});
$squared_odd_numbers = array_map(function($number) {
return $number ** 2;
}, $odd_numbers);
print_r($squared_odd_numbers);
The snippet starts by first generating a processed array, $odd_numbers, which is an extraction of odd numbers from the original number sequence (1 to 10). It uses the array_filter() function, with a callback function that retains only odd numbers in the array.
Next, it employs the array_map() function to go through the $odd_numbers array and return a new array, $squared_odd_numbers, where each element is the square of the corresponding element in the $odd_numbers array.
The output from the above code is as follows:
Array
(
[0] => 1
[2] => 9
[4] => 25
[6] => 49
[8] => 81
)
Conclusion
In this tutorial, we have learned how to master PHP’s `array_filter()` function for filtering arrays. We have explored its basic usage, implemented custom callback functions for advanced filtering, filtered arrays by keys, and combined it with other array functions for more complex operations. This powerful function is an essential tool for software developers, especially those who hire PHP developers for web development projects.