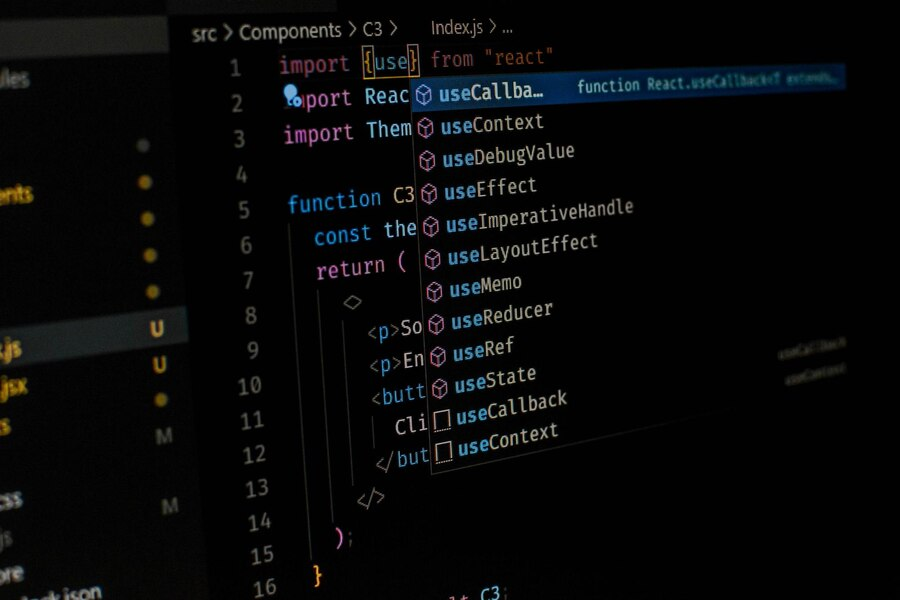
Defining the array_map() Function
The array_map() function stands as a pivotal utility in PHP, enabling developers to apply a specified user-defined function to each item within one or more arrays. It accepts a callback function and an array (or arrays) as parameters, processes each element through the callback function, and generates a new array featuring the transformed elements.
Syntax Overview
The general syntax for the array_map() function is as follows:
array_map(callback, array1, array2, …) |
Here, the callback represents the function to be applied to each array element, array1 is the compulsory initial array, and array2, … denote optional subsequent arrays.
Implementing array_map() – Basic Applications
Example 1: Transforming a Single Array
To illustrate, consider a scenario where we aim to square every number in an array:
function square($n) { return $n * $n;} $numbers = [1, 2, 3, 4, 5];$result = array_map(‘square’, $numbers); print_r($result); |
Output:
Array( [0] => 1 [1] => 4 [2] => 9 [3] => 16 [4] => 25) |
Example 2: Merging Multiple Arrays
Next, we demonstrate adding corresponding elements from two distinct arrays:
function add($a, $b) { return $a + $b;} $array1 = [1, 2, 3, 4, 5];$array2 = [6, 7, 8, 9, 10];$result = array_map(‘add’, $array1, $array2); print_r($result); |
Output:
Array( [0] => 7 [1] => 9 [2] => 11 [3] => 13 [4] => 15) |
Advanced Application: Utilizing Anonymous Functions
array_map() also supports the use of anonymous functions, offering a concise syntax for on-the-fly computations:
$numbers = [1, 2, 3, 4, 5];$result = array_map(function($n) { return $n * $n;}, $numbers); print_r($result); |
Output:
Array( [0] => 1 [1] => 4 [2] => 9 [3] => 16 [4] => 25) |
Comparative Analysis: array_map() vs. Other Array Functions in PHP
To further understand the array_map() function’s unique position within PHP’s array manipulation capabilities, let’s compare it with similar functions, highlighting their uses, advantages, and differences.
Feature/Function | array_map() | array_filter() | array_reduce() |
---|---|---|---|
Purpose | Applies a callback to each element of one or more arrays. | Filters elements of an array using a callback function. | Iteratively reduces an array to a single value using a callback function. |
Return Value | A new array containing all the elements after applying the callback function. | A new array containing elements that pass the callback function test. | A single value resulting from the cumulative application of the callback function. |
Callback Function | Mandatory; defines the operation to be applied to each element. | Optional; determines which elements to include based on a truth test. | Mandatory; defines how to combine elements. |
Multiple Arrays | Supports multiple arrays. | Operates on a single array. | Operates on a single array. |
Example Use Case | Transforming each element of an array (e.g., squaring numbers). | Removing elements that don’t meet certain criteria (e.g., filtering out odd numbers). | Accumulating values (e.g., summing numbers). |
This table delineates the functional distinctions between array_map(), array_filter(), and array_reduce(), each serving different purposes in array manipulation. While array_map() excels in applying transformations across array elements, array_filter() is optimal for extracting subsets based on conditions, and array_reduce() is key for aggregating array elements into a single outcome. Understanding these differences is crucial for selecting the most appropriate function for a given task, thereby enhancing the efficiency and readability of your PHP code.
Conclusion
Through exploring the array_map() function, its syntax, and practical applications, we’ve highlighted its versatility and power in processing array elements in PHP. Whether applying simple transformations or engaging in more complex data manipulation, array_map() offers a robust solution for enhancing your coding efficiency and capabilities.