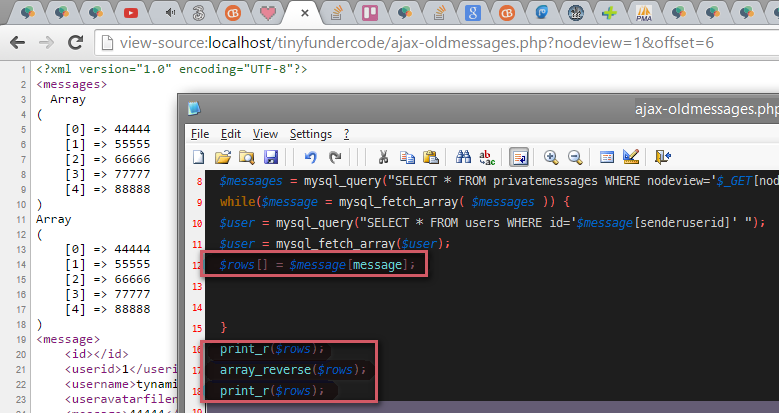
Arrays are fundamental data structures in PHP, offering a versatile way to store and manipulate data. One essential operation developers frequently encounter is reversing an array. PHP provides a built-in function, array_reverse(), which simplifies this task. In this tutorial, we’ll explore the various aspects of using array_reverse() effectively.
Understanding array_reverse()
Before diving into practical examples, let’s examine the syntax of array_reverse():
php
array array_reverse ( array $array [, bool $preserve_keys = FALSE ] )
- $array: The input array to be reversed;
- $preserve_keys (optional): If set to TRUE, the original keys will be preserved. Default is FALSE.
Reversing Basics
Let’s start with a simple example:
php
$fruits = array("apple", "banana", "cherry"); $reversed_fruits = array_reverse($fruits); print_r($reversed_fruits);
The output will be:
csharp
Array ( [0] => cherry [1] => banana [2] => apple )
By default, array_reverse() preserves the original array’s keys.
Reversing Indexed Arrays
php
$numbers = array(1, 2, 3, 4, 5); $reversed_numbers = array_reverse($numbers); print_r($reversed_numbers);
Output:
csharp
Array ( [0] => 5 [1] => 4 [2] => 3 [3] => 2 [4] => 1 )
Preserving Keys
To preserve keys:
php
$colors = array("red" => "apple", "yellow" => "banana", "purple" => "grape"); $reversed_colors = array_reverse($colors, true); print_r($reversed_colors);
Output:
csharp
Array ( [purple] => grape [yellow] => banana [red] => apple )
Reversing Multidimensional Arrays
Consider a multidimensional array:
php
$matrix = array( array(1, 2, 3), array(4, 5, 6), array(7, 8, 9) ); $reversed_matrix = array_reverse($matrix); print_r($reversed_matrix);
Output:
csharp
Array ( [0] => Array ( [0] => 7 [1] => 8 [2] => 9 ) [1] => Array ( [0] => 4 [1] => 5 [2] => 6 ) [2] => Array ( [0] => 1 [1] => 2 [2] => 3 ) )
Applying array_reverse() Conditionally
You might want to reverse an array based on a certain condition. Let’s say you want to reverse the array only if it contains more than three elements:
php
$data = array(1, 2, 3, 4, 5); if (count($data) > 3) { $reversed_data = array_reverse($data); print_r($reversed_data); } else { print_r($data); }
Performance Considerations
When dealing with large arrays, be mindful of performance implications. Reversing an array involves rearranging elements, so use array_reverse() judiciously in performance-critical scenarios.
The term “performance considerations” is quite broad, and the specifics of what I can tell you depend on the context. To give you the most relevant and helpful information, I need some more details. For example, are you interested in:
- Software performance: This could include factors like response time, resource usage, scalability, and stability. Are you building a website, mobile app, backend system, or something else? What are your performance goals?;
- Hardware performance: This could involve processor speed, memory capacity, storage bandwidth, and network latency. What type of hardware are you concerned about (computers, servers, embedded systems)? What tasks do you need it to perform efficiently?;
- Algorithmic performance: This refers to the efficiency of different algorithms for solving a particular problem. Are you trying to optimize a specific algorithm or compare different approaches?;
- Other types of performance: The term can also be used in various contexts, such as financial performance, project performance, or even athletic performance. What specific area are you interested in?
Once you provide me with more context, I can offer you specific advice, resources, and tools to help you optimize performance in your chosen area.
When working with large arrays, it’s essential to be mindful of performance considerations. Reversing a massive dataset can impact the execution time. Let’s explore a simple example to illustrate the potential performance impact and a strategy to mitigate it:
php
<?php // Generate a large array with 1 million elements $largeArray = range(1, 1000000); // Measure the time taken to reverse the array without optimization $start_time = microtime(true); $reversedArray = array_reverse($largeArray); $end_time = microtime(true); $execution_time = ($end_time - $start_time) * 1000; // Convert to milliseconds echo "Time taken without optimization: {$execution_time} ms\n"; // Optimize the reversal process using array pointer manipulation $start_time = microtime(true); $reversedArray = []; while ($element = array_pop($largeArray)) { $reversedArray[] = $element; } $end_time = microtime(true); $execution_time = ($end_time - $start_time) * 1000; echo "Time taken with optimization: {$execution_time} ms\n";
In this example, we generate a large array with 1 million elements using the range() function. We then measure the time it takes to reverse the array using the array_reverse() function. Additionally, we demonstrate an optimized approach using array pointer manipulation with array_pop().
Running this code will likely show that the optimized method is faster than using array_reverse() for large arrays. However, it’s crucial to note that the optimization strategy might not be suitable for all scenarios, and the choice depends on the specific requirements of your application.
Always consider the nature of your data and the operations you need to perform. Profiling tools and benchmarking can be valuable for assessing the performance impact of different approaches in real-world scenarios.
Chaining array_reverse()
You can chain multiple array_reverse() calls to achieve complex transformations:
php
$transformed_data = array_reverse(array_reverse($data), true);
Conclusion
In this tutorial, we’ve explored the array_reverse() function in PHP, covering its syntax, practical applications, and best practices. Understanding how to reverse arrays is crucial for efficient array manipulation in your PHP projects. By applying the knowledge gained here, you can enhance your coding skills and create more robust and readable code. Happy coding!