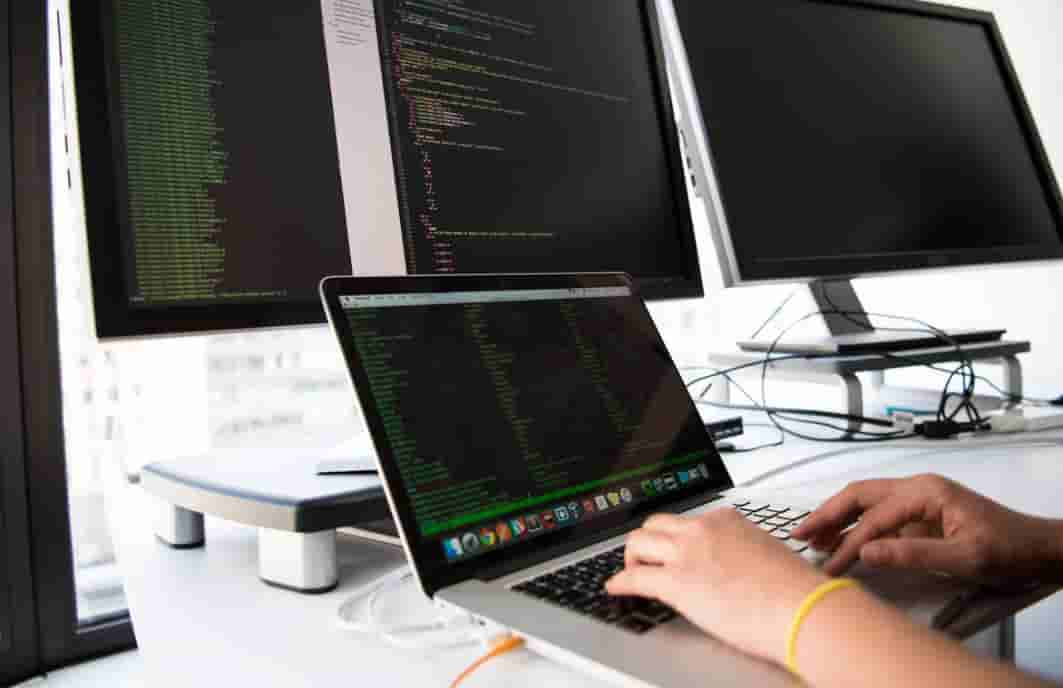
PHP, a server-side scripting language, is a staple in web development due to its extensive built-in functions and ability to create dynamic web applications. A key player in PHP’s function arsenal is the `array_search()` function, pivotal for searching and filtering within arrays. This tutorial aims to demystify `array_search()` and demonstrate its practical application in PHP development.
The Mechanics of `array_search()`
At its core, the `array_search()` function serves to locate a specific value within an array, returning the key associated with the value if successful. Its syntax is straightforward:
array_search(value, array, strict)
The function’s parameters include:
- value: This is the element that the function aims to find within the array. It can be of any data type, such as a string, integer, or even an object. The versatility in the ‘value’ parameter allows for a broad range of search possibilities within an array;
- array: As the name suggests, this parameter represents the array in which the search is to be conducted. The array can be indexed, associative, or multidimensional, giving the function a wide application scope in different array structures;
- strict (optional): This boolean parameter determines the nature of the comparison during the search process. When set to true (strict), the function compares both the value and the data type, ensuring an exact match. In contrast, a false value (loose comparison) disregards the data type, focusing solely on the value. This flexibility is crucial for precision in searches where data type consistency might vary.
Upon executing the search, if the ‘value’ is found within the ‘array’, `array_search()` returns the key associated with the first occurrence of that value. This aspect is particularly important when dealing with arrays containing duplicate values. However, if the value is not found, the function yields false, indicating the absence of the searched element in the array. This return behavior of `array_search()` makes it a reliable tool for not only searching arrays but also for validating the existence of elements within them.
The function’s ability to handle both strict and loose comparisons makes it adaptable for various scenarios. For example, in data processing where type consistency is not guaranteed, the loose comparison (false) is beneficial. Conversely, in scenarios demanding data type integrity, the strict comparison (true) ensures more accurate search results. This versatility underscores the utility of `array_search()` in diverse PHP programming contexts.
Practical Application of `array_search()`
Consider an array of numbers, with the task of identifying the key for the value 5:
```php
$numbers = array(1, 2, 3, 4, 5, 6, 7, 8, 9);
$key = array_search(5, $numbers);
echo $key;
```
Here, `array_search()` returns 4, pinpointing the location of the value 5 in the array.
Implementing Strict Comparison
By default, `array_search()` operates with loose comparison, which can yield unexpected results due to different data types. Enforcing strict comparison can be achieved by setting the third parameter to true:
```php
$numbers = array(1, 2, '3', 4, '5', 6, 7, 8, 9);
$key = array_search(5, $numbers, true);
echo $key;
```
In this case, the function returns false, as ‘5’ (string) is not strictly equal to 5 (integer).
Array Filtering with `array_search()`
`array_search()` is adept at array filtering, as illustrated in the following scenario:
```php
$users = array(
array('name' => 'John', 'role' => 'admin'),
array('name' => 'Jane', 'role' => 'editor'),
array('name' => 'Jack', 'role' => 'admin'),
array('name' => 'Jill', 'role' => 'subscriber')
);
$admins = array_filter($users, function($user) {
return array_search('admin', $user) !== false;
});
print_r($admins);
```
In this example, users with the ‘admin’ role are isolated using `array_filter()` in conjunction with `array_search()`.
Conclusion
This tutorial has offered a comprehensive exploration of the `array_search()` function, a cornerstone in PHP programming. Grasping the intricacies of this function is vital for developers who wish to efficiently manage array operations, a common task in web development. By mastering `array_search()`, developers can significantly reduce the complexity of their code, making it more readable and maintainable. This function is especially useful in scenarios involving data filtering, validation, and transformation within PHP applications, enhancing both the developer’s productivity and the application’s performance.
Moreover, the use of `array_search()` in various contexts, from simple value searches to complex data filtering, highlights its adaptability and power in PHP development. It’s a testament to PHP’s flexibility and robustness as a server-side scripting language, capable of handling diverse programming needs.
For developers less familiar with PHP or those tackling complex projects, seeking the expertise of experienced PHP developers is invaluable. These professionals not only bring a deep understanding of functions like `array_search()` but also provide insights into best practices and advanced techniques in PHP. Their guidance can be instrumental in navigating the intricacies of PHP development, ensuring the creation of efficient, scalable, and high-quality web applications. Thus, investing in professional assistance is a wise decision for those aiming to leverage PHP to its fullest potential.