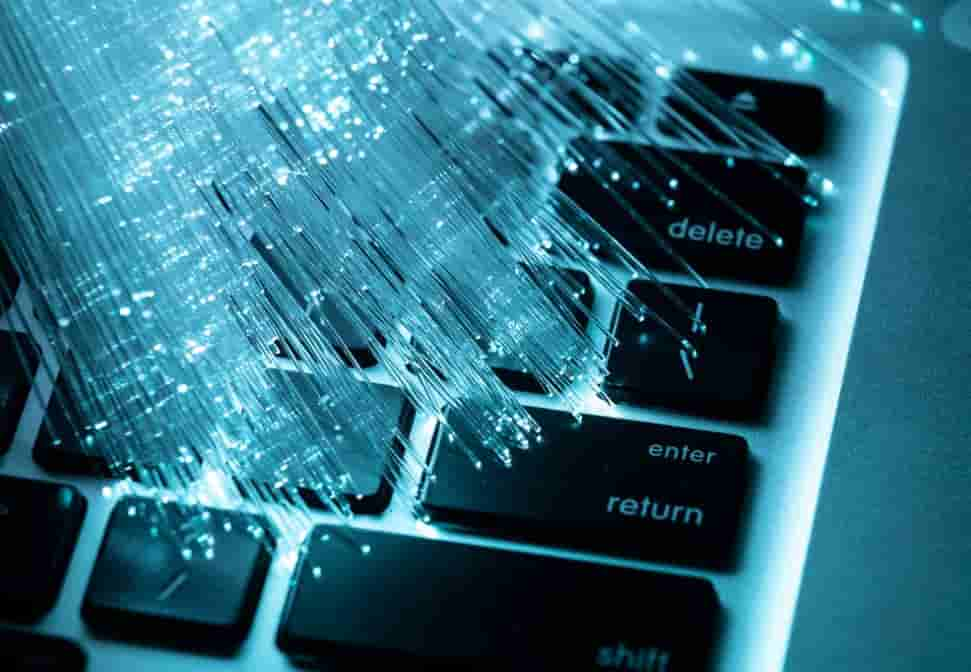
In this tutorial, we will explore the PHP array_values() function and its practical applications for array reindexing. By the end of this guide, you will have a clear understanding of how to use array_values() to optimize your code. Let’s begin!
While discussing PHP’s array_values() function, it’s worth noting that PHP Websockets can also be a valuable tool for real-time communication in web applications.
array_values() Function in PHP
The array_values() function in PHP is a native function designed to retrieve all the values from an input array and return them in a new indexed array. This operation essentially reindexes the input array, ensuring that the keys are continuous without any gaps in the sequence.
Here’s the syntax for using the array_values() function:
array_values(array $input): array;
As depicted in the syntax, this function takes an input array and generates a fresh array where the keys have been reindexed for smooth and uninterrupted numerical sequence.
Benefits of Using PHP’s array_values() Function
The utilization of PHP’s array_values() function can bring several advantages to your code, making it a valuable tool in various scenarios:
- Resetting Keys: In situations where you remove an element from an array using functions like unset() or other methods, the keys are not automatically reindexed. This can result in discontinuous index sequences with gaps. By employing the array_values() function, you can effectively reset the keys, ensuring a smooth and continuous sequence;
- Converting Associative Arrays to Indexed Arrays: If you have an associative array and the need arises to convert it into an indexed array, the array_values() function is your solution. It simplifies the conversion process, providing you with an indexed array format;
- Removing Duplicate Values: Combining the array_values() function with other functions such as array_unique() enables you to not only eliminate duplicate values from an array but also reindex the keys simultaneously. This can lead to cleaner and more efficient data structures in your code.
Practical Applications of PHP’s array_values() Function
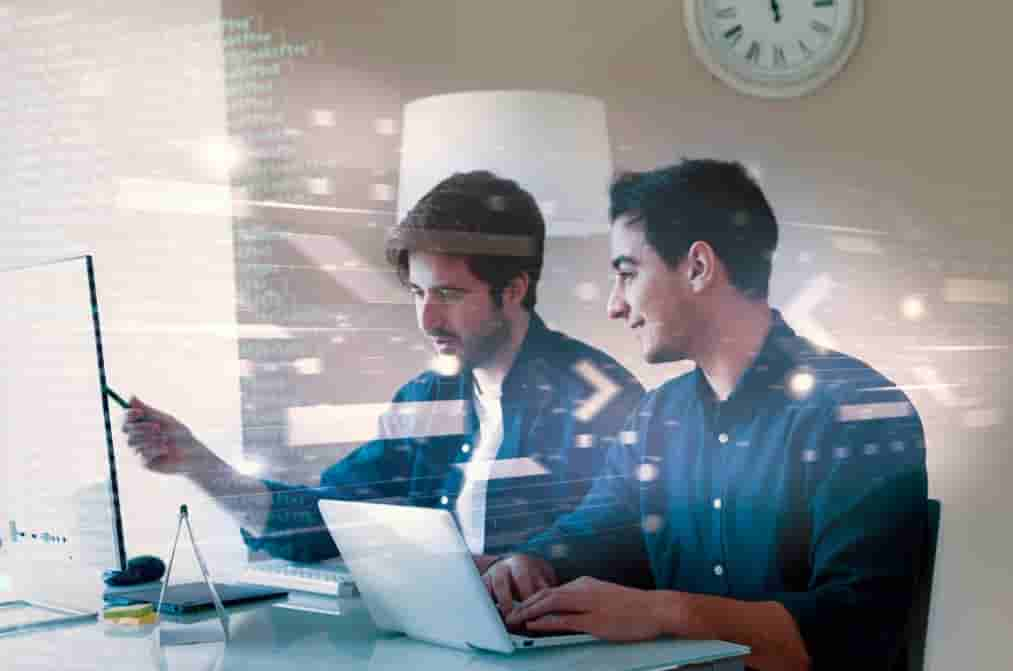
Now that we have established the purpose and advantages of the array_values() function, let’s proceed to examine concrete, real-world instances of its utilization. These practical applications will help reinforce your understanding of this versatile PHP function.
Example 1: Reindexing Array Keys
Consider the following array:
$array = [1, 2, 3, 4, 5];
If we remove the element with the key 2 using the unset() function, we obtain the following array:
unset($array[2]);
print_r($array); // Array ( [0] => 1 [1] => 2 [3] => 4 [4] => 5 )
As observed, there is a gap in the index sequence. To reset the keys and create a continuous index sequence, we can employ the array_values() function, as demonstrated below:
$newArray = array_values($array);
print_r($newArray); // Array ( [0] => 1 [1] => 2 [2] => 4 [3] => 5 )
In this example, the array_values() function successfully reindexes the array keys, resulting in a sequential and gap-free index sequence.
Example 2: Transforming Associative Arrays into Indexed Arrays
Suppose we have the following associative array:
$assocArray = ['a' => 'apple', 'b' => 'banana', 'c' => 'cherry'];
To convert this associative array into an indexed array, we can readily employ the array_values() function, as illustrated below:
$indexedArray = array_values($assocArray);
print_r($indexedArray); // Array ( [0] => apple [1] => banana [2] => cherry )
In this example, the array_values() function effectively transforms the associative array into an indexed array, preserving the values while eliminating the associative keys.
Example 3: Eliminating Duplicate Values and Reindexing Keys
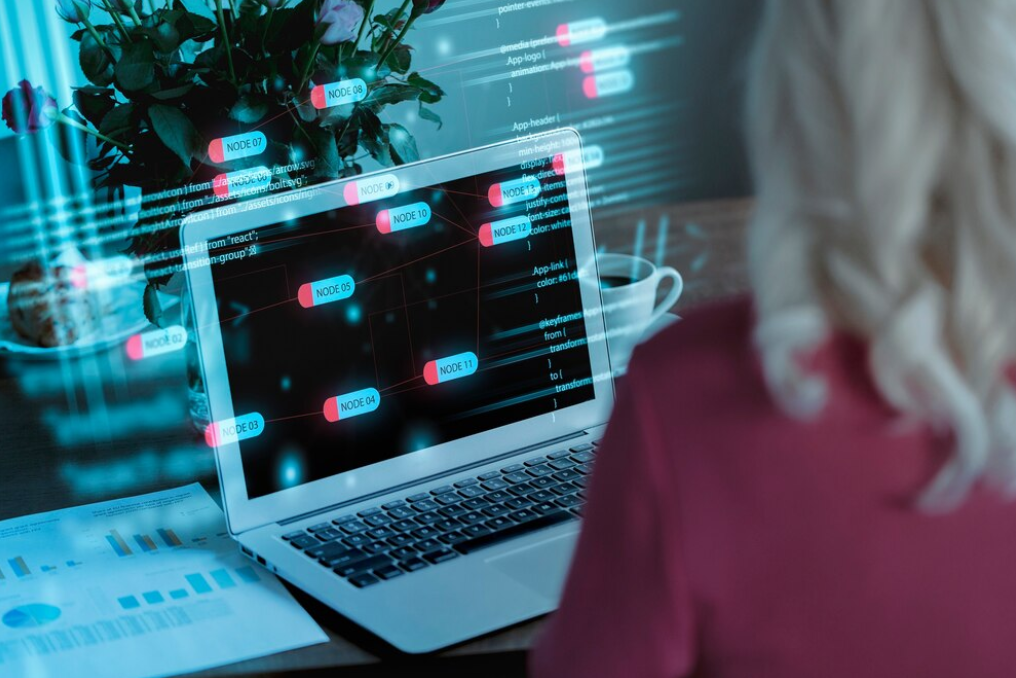
Imagine we have the following array containing duplicate values:
$duplicatesArray = [1, 2, 3, 2, 4, 1, 5, 3];
To eliminate the duplicate values and reindex the keys, we can effectively combine the array_values() function with the array_unique() function, as demonstrated in the code snippet below:
$uniqueArray = array_values(array_unique($duplicatesArray));
print_r($uniqueArray); // Array ( [0] => 1 [1] => 2 [2] => 3 [3] => 4 [4] => 5 )
In this example, the combined use of array_values() and array_unique() efficiently removes duplicate values from the array and simultaneously reindexes the keys, resulting in a clean and streamlined array structure.
Conclusion
Throughout this tutorial, we have delved into the functionality of the array_values() function in PHP and its practical applications for array reindexing. We’ve examined various use cases and offered illustrative examples to equip you with the knowledge needed to seamlessly integrate this function into your coding endeavors.