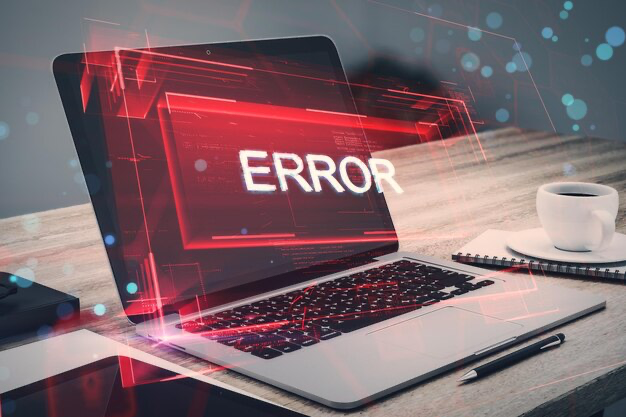
This guide offers a comprehensive overview of PHP’s error_reporting() function, a critical debugging and error management tool for PHP scripts. We’ll explore its setup and application, providing practical code examples and recommended practices.
Exploring PHP Error Reporting
The error_reporting() function in PHP is essential for indicating which errors should be reported and displayed. It enables you to adjust the error_reporting directive during runtime, thus tailoring error reporting levels for your scripts. This functionality is invaluable for PHP application development and debugging, as it highlights potential code issues.
Setting Up Error Reporting
There are various methods to configure error reporting in PHP:
- php.ini: Define the error_reporting directive in the php.ini file;
- .htaccess: Specify the error_reporting directive in the .htaccess file (for Apache servers);
- Runtime: Adjust the error_reporting directive dynamically using the error_reporting() function.
While modifying the php.ini file is the preferred method for uniform application-wide error reporting settings, there are situations where runtime adjustments using the error_reporting() function are necessary.
Harnessing the Power of the error_reporting() Function: A Practical Guide
The error_reporting() function in PHP is a powerful tool that takes an integer value as a parameter to signify the precise level of error reporting desired. The function provides various predefined constants, allowing developers to tailor the error reporting level based on their specific needs:
- E_ALL: This value covers all types of errors and warnings, including E_STRICT;
- E_ERROR: Focused on fatal run-time errors;
- E_WARNING: Highlights run-time warnings that are non-fatal errors;
- E_PARSE: Pinpoints compile-time parse errors;
- E_NOTICE: Catches run-time notices which may indicate potential bugs or issues;
- E_STRICT: Provides run-time notices that propose enhancements to your code;
- E_DEPRECATED: Issues warnings about features that are deprecated.
To broaden your scope and report various types of errors, a bitwise OR operator (|) can be used to merge multiple error reporting levels. For instance, say you wish to report errors, warnings, and notices. Your code will look something like this:
error_reporting(E_ERROR | E_WARNING | E_NOTICE);
For a development environment, it’s often recommended to set the error reporting level to E_ALL. Why? Because this level brings the most exhaustive error reporting to the table:
error_reporting(E_ALL);
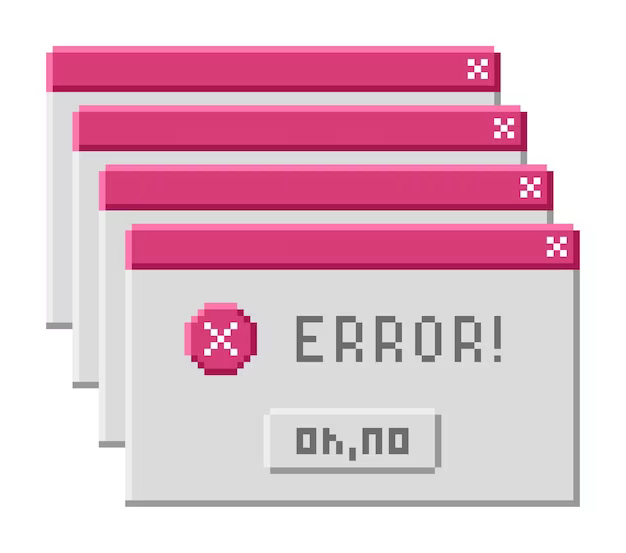
Best Practices for Error Reporting
Error reporting is an indispensable part of PHP development. However, just knowing how to use the error_reporting() function is not sufficient. Following field-tested best practices can help you extract the most value out of this essential function:
- Maximize Error Capture in Development Stage: When developing, it’s paramount to thoroughly inspect your code for potential challenges and bugs. Therefore, set your error reporting level to E_ALL. This configuration provides a comprehensive overview of all errors and warnings, including E_STRICT notices;
- Safeguard Production Environments: In production environments, error reporting can potentially expose sensitive data, thereby posing a security risk. It’s advisable to completely disable error reporting in such situations, ensuring that your users aren’t privy to any error messages. However, to maintain visibility over error occurrences, log them to a file for subsequent review and troubleshooting;
- Harness Custom Error Handlers: PHP allows you to design custom error handlers, granting you granular control over the error handling and reporting process. This feature can be invaluable in managing errors in a more refined manner, tailored to your specific needs;
- Maintain Code Modernity: One of the integral parts of PHP development is keeping your PHP versions and libraries up-to-date. Regular updates ensure that you are not using deprecated features, which can trigger E_DEPRECATED warnings. Furthermore, updates also bring improvements in performance and security, making your PHP applications more robust and efficient.
Exploring PHP Explode Function
In the expansive realm of PHP, the explode() function plays a pivotal role in parsing strings. This versatile function enables developers to split a string into an array based on a specified delimiter. The explode() function’s utilization can greatly assist in error handling and reporting, as it allows for the efficient extraction and manipulation of data contained within strings. By integrating the explode() function with the principles of error reporting elucidated in this guide, developers can achieve a more comprehensive and robust approach to handling errors and managing data in PHP-based applications.
Conclusion
Grasping the nuances of PHP’s error_reporting() function is essential for software developers aiming to master debugging and error handling in PHP-based applications. This tutorial has offered key best practices for employing the error_reporting() function effectively. By applying these principles diligently, developers can achieve robust and seamless performance in their PHP applications, ensuring they operate flawlessly in both development and production settings.