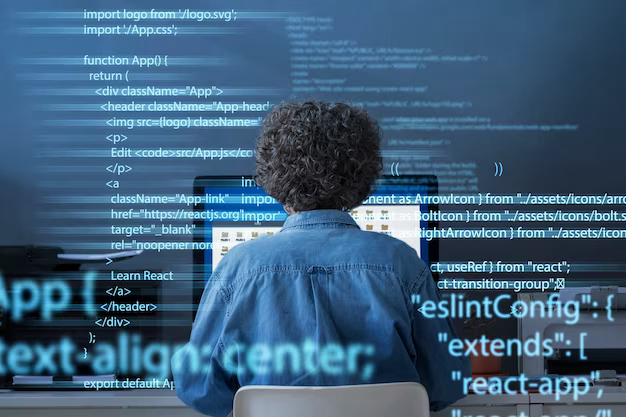
In this detailed guide, we delve into the intricacies of the PHP explode() function. We’ll cover its functionality, structure, various parameters, and provide a range of examples to enhance your comprehension and utilization in PHP programming. Grasping the explode() function is essential for effective string handling and data management in PHP, making it an indispensable skill for all PHP programmers.
Unraveling the PHP explode() Function
In the realm of PHP, one of the most common string manipulation tools we come across is the explode() function. This function essentially breaks down a string into a PHP array using a specified delimiter. It’s a handy tool for processing diverse sets of data, which can come from user inputs, files, and more. The explode() function is a lifesaver when dealing with comma-separated values (CSV), scrutinizing log files, or parsing through command-line arguments.
Syntax
Before we move further, let’s become familiar with its simple yet robust syntax:
array explode ( string $delimiter , string $string [, int $limit ] )
Parameters Explained
The explode() function takes in three parameters, two of which are mandatory while the third is optional:
- $delimiter – This parameter is also known as the boundary string and is used to define where the input string should be divided;
- $string – This is the input string that needs to be split up into an array;
- $limit (optional) – This parameter defines the maximum number of elements that the result array can contain. If specified, the returned array will contain a maximum of $limitelements with the last element containing the rest of the string. If $limit is negative, all elements barring the last -$limit elements are returned. The behavior with $limit set to zero may vary depending on the PHP version in use.
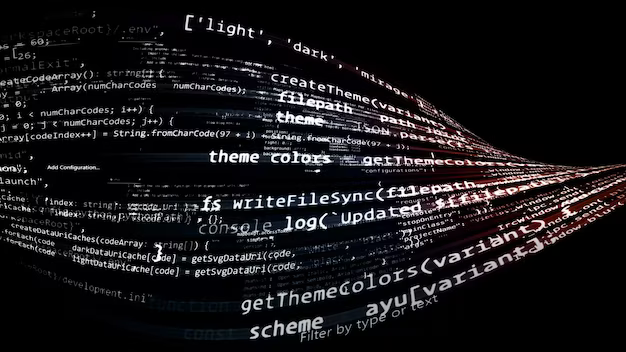
Practical Applications of the PHP explode() Function
To truly appreciate the versatility of the PHP explode() function, let’s delve into a few practical examples. Each example will depict how this function can be used in a real-world scenario.
Elementary Use Case
Consider a scenario where you have a string of names separated by commas, and you want to separate each name. This is a basic example of how explode() can be used:
$names = "Alice, Bob, Charlie, David";
$nameArray = explode(", ", $names);
print_r($nameArray);
The output will yield:
Array
(
[0] => Alice
[1] => Bob
[2] => Charlie
[3] => David
)
Delving into the $limit Parameter
To understand the $limit parameter, let’s look at a similar scenario but now with a limit of 2:
$names = "Alice, Bob, Charlie, David";
$nameArray = explode(", ", $names, 2);
print_r($nameArray);
The output in this case will be a bit different:
Array
(
[0] => Alice
[1] => Bob, Charlie, David
)
As seen above, the resulting array contains only two elements since we stipulated the $limit parameter to 2.
Tackling CSV Data
The explode() function truly shines when working with CSV data. For instance, consider the following data:
$data = "Alice, Doe, 25, female
Bob, Smith, 30, male";
This data can be processed and stored in a multi-dimensional array using explode(). Here’s how to do it:
$lines = explode("\n", $data);
foreach ($lines as $index => $line) {
$lines[$index] = explode(", ", $line);
}
print_r($lines);
The output will result in a well-structured array:
Array
(
[0] => Array
(
[0] => Alice
[1] => Doe
[2] => 25
[3] => female
)
[1] => Array
(
[0] => Bob
[1] => Smith
[2] => 30
[3] => male
)
)
In this example, the input data was first segmented into individual lines. Each line was then split into separate components, thereby transforming the CSV data into a multi-dimensional array for easier manipulation.
In Conclusion
The PHP explode() function is not just a tool, but rather a boost to making your PHP code more efficient and controllable. The examples provided in this article only scratch the surface of its potential. As you continue to hone your PHP skills, you’ll discover more unique and advanced ways of utilizing the explode() function.