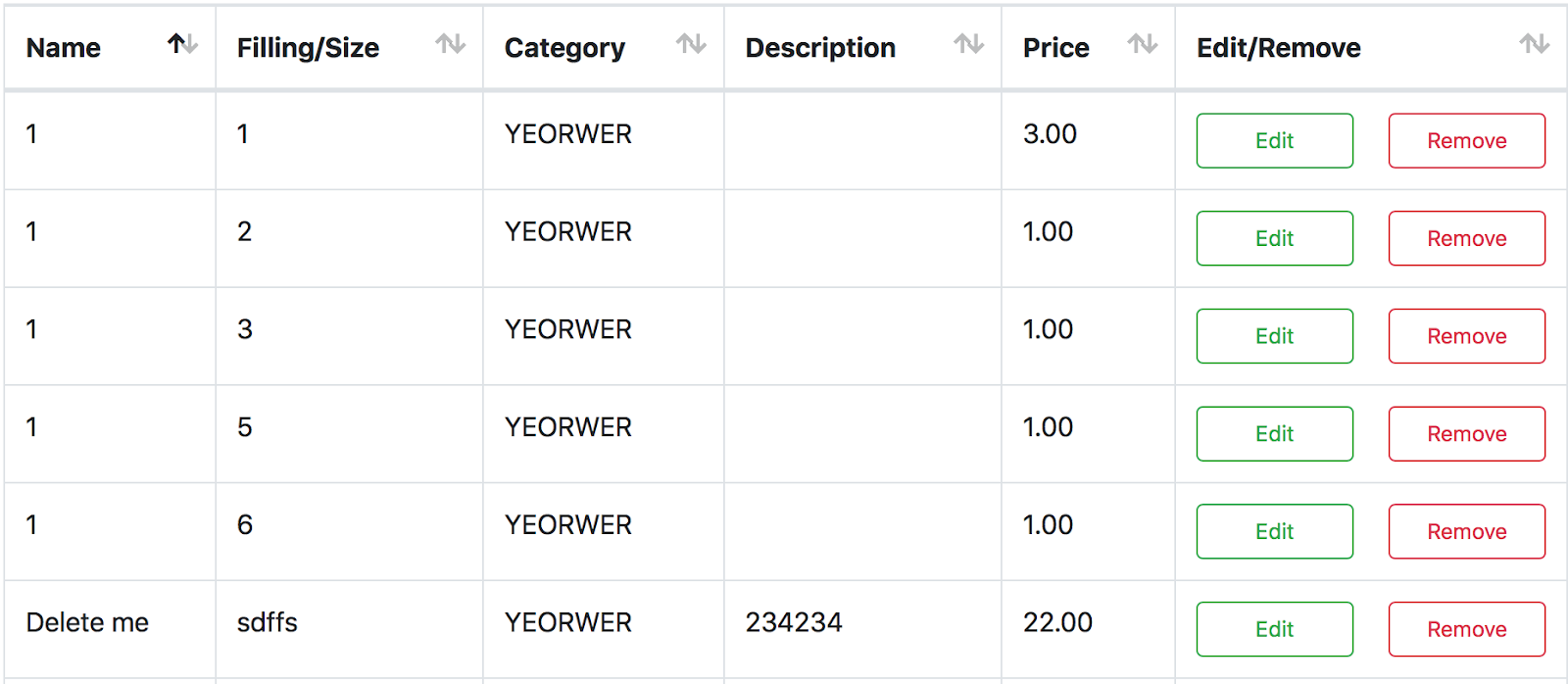
Immerse yourself in the capabilities of PHP, a versatile scripting language, with its powerful foreach loop crafted to streamline array traversal. Explore the intricacies of this loop to enhance your coding efficiency.
Understanding the Basics
The foreach loop is crafted for traversing arrays and other iterable objects with a Simple syntax
php
foreach ($array as $value) { // code executed for each $value }
In this structure, $array is the iterable variable and $value is the current element being processed. We will have a closer look at how this basic structure can be used in a variety of scenarios.
Associative Arrays
For associative arrays, where you need to access both keys and values, foreach is useful.
php
$person = array("name" => "John", "age" => 30, "city" => "New York"); foreach ($person as $key => $value) { echo $key . ": " . $value . "<br>"; }
In this case, the loop will iterate through the associative array and will print out both the key and the value. This ability to handle associative arrays effortlessly is one of the foreach loop’s strengths.
Nested Loops
Beneficial when dealing with complex data structures.
php
$matrix = array( array(1, 2, 3), array(4, 5, 6), array(7, 8, 9) );
foreach ($matrix as $row) {
foreach ($row as $value) {
echo $value . " ";
}
echo "<br>";
}
In this example, nested loops are traversing a two-dimensional array and printing its contents one line at a time. Proficiency in utilizing nested foreach loops amplifies your capability to navigate intricate data structures.”
Best Practices and Tips
To fully utilize foreach loops, follow these best practices:
- Avoid modifying array elements: Unpredictable results can result from modifying array elements during iteration;
- Use as value to reference: To modify array elements directly, use the as value syntax. This ensures that updates affect the original array; If modification is necessary, consider using;
- Check if the array is empty: Before using foreach, check that the array is empty to avoid unnecessary iterations. This improves code efficiency and reduces errors;
Enhance array destructuring by incorporating the list() function with foreach, simplifying the extraction of values.
For the majority of array traversal scenarios, the foreach loop yields code that is cleaner and more readable compared to traditional for loops. Reserve for loops for situations that necessitate precise index manipulation.
Conclusion
Developing expertise in the foreach loop is imperative for PHP developers aiming to create clean, efficient, and maintainable code. Its simplicity, versatility, and compatibility with various iterable structures make it a valuable asset in your programming toolkit. This tutorial offers examples and best practices, enabling you to unleash the full potential of the foreach loop in your PHP projects with confidence.