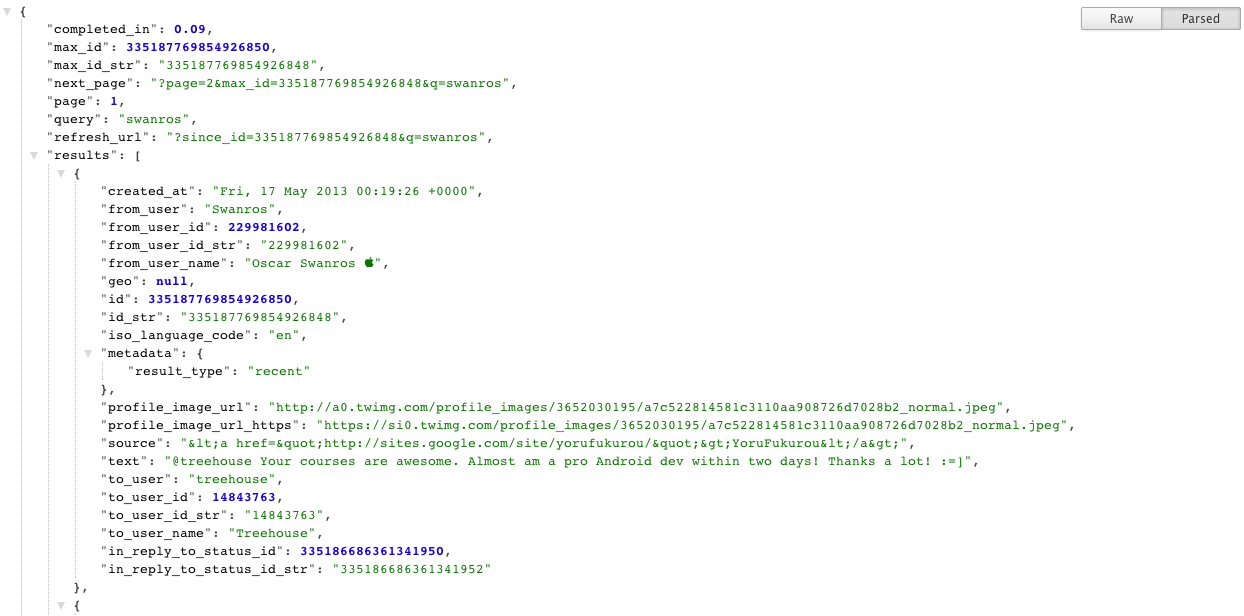
In the dynamic landscape of web development, effective data exchange is crucial. JSON (JavaScript Object Notation) serves as a common format for data interchange. PHP, being a versatile server-side scripting language, provides the json_encode function to facilitate the conversion of PHP data structures into JSON format. In this tutorial, we’ll delve into the intricacies of json_encode and explore how it can be harnessed to enhance the efficiency of your PHP applications.
Understanding json_encode
The json_encode function in PHP is pivotal for transforming PHP data structures into JSON-encoded strings. It takes a PHP value (array, object, scalar, or a combination) and converts it into a JSON representation. This encoded JSON string can then be easily transmitted to other systems or stored for later use.
Let’s delve into a detailed explanation of the json_encode code example provided in the tutorial:
php
$data = array( 'name' => 'John Doe', 'age' => 30, 'city' => 'New York' ); $jsonString = json_encode($data); echo $jsonString;
In summary, this code demonstrates a basic usage of json_encode to convert a simple PHP associative array into a JSON-encoded string. This is a fundamental step when preparing data for communication between different systems or when storing data in a format that is easily transportable and compatible with various programming languages.
Basic Usage
Let’s start with a simple example. Suppose you have an associative array in PHP:
php
$data = array( 'name' => 'John Doe', 'age' => 30, 'city' => 'New York' ); $jsonString = json_encode($data); echo $jsonString;
In this case, json_encode converts the PHP array into a JSON-encoded string, producing:
json
{"name":"John Doe","age":30,"city":"New York"}
Handling Options
json_encode allows you to customize the encoding process using optional parameters. For instance, you can use JSON_PRETTY_PRINT to format the JSON string for better human readability:
php
$prettyJsonString = json_encode($data, JSON_PRETTY_PRINT); echo $prettyJsonString;
This produces a neatly formatted JSON string:
json
{ "name": "John Doe", "age": 30, "city": "New York" }
Dealing with Complex Data
When dealing with more complex data structures, such as nested arrays or objects, json_encode seamlessly handles the encoding process. Consider the following example:
php
$complexData = array( 'user' => array( 'name' => 'Jane Doe', 'age' => 25, 'address' => array( 'city' => 'Los Angeles', 'zipcode' => '90001' ) ), 'status' => 'active' ); $complexJsonString = json_encode($complexData); echo $complexJsonString;
This yields a JSON string representing the nested structure:
json
{ "user": { "name": "Jane Doe", "age": 25, "address": { "city": "Los Angeles", "zipcode": "90001" } }, "status": "active" }
Handling Errors
Error handling is crucial when working with JSON encoding. PHP provides the json_last_error() function to retrieve the last JSON error that occurred during the encoding process. Checking for errors ensures robust code that gracefully handles unexpected scenarios:
php
$jsonString = json_encode($invalidData); if (json_last_error() !== JSON_ERROR_NONE) { echo 'Error encoding JSON: ' . json_last_error_msg(); } else { echo $jsonString; }
This prevents potential issues and provides insights into the nature of any encountered errors.
Conclusion
Mastering json_encode in PHP empowers developers to seamlessly serialize PHP data for efficient communication with other systems, especially in the context of web APIs and data exchange. Whether dealing with basic arrays or complex data structures, understanding the nuances of json_encode is a valuable skill. With this tutorial, you’re now equipped to leverage the power of JSON encoding in your PHP applications, enhancing their flexibility and interoperability.