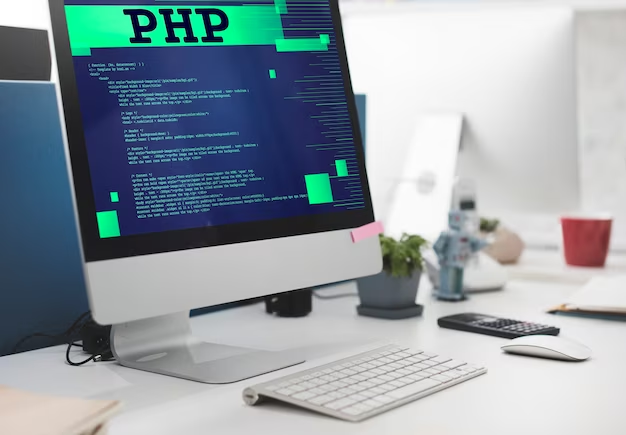
Microservices, small and autonomous services comprising a complex software system, have gained prominence for enhancing modularity, scalability, and flexibility in software development. This tutorial provides a thorough understanding of constructing and managing PHP-based microservices.
Advantages of PHP in Microservice Architecture
PHP, a widely-used programming language, is increasingly preferred for microservice development due to:
- Enhanced Performance: Recent versions of PHP, such as 7.x and 8.x, offer considerable performance improvements, making it a strong candidate for efficient microservice construction;
- Robust Frameworks: PHP boasts a range of mature frameworks like Laravel and Symfony, offering essential tools and infrastructure for microservice development;
- Extensive Community Support: PHP’s large and active community provides ample resources and support for developers.
Key Principles for Designing Microservices
Effective microservice architecture necessitates meticulous planning and design. Consider these critical aspects:
- Domain-Driven Design (DDD): Emphasize modeling your system around real-world business domains, identifying key business components or “bounded contexts” to structure your microservices;
- API Design: Microservices communicate through APIs.
Carefully design APIs with attention to versioning, authentication, and error handling to ensure seamless interaction between services.
- Data Management: Each microservice should possess its data store. Avoid direct data access between services; utilize APIs to maintain clear boundaries and data integrity.
Building Microservices with PHP: A Step-by-Step Approach
Using the Laravel framework as an example, let’s explore how to implement PHP microservices:
Setting Up a Laravel Project
Begin by creating a new Laravel project with Composer:
composer create-project –prefer-dist laravel/laravel my-microservice |
Defining Routes and Controllers
In routes/api.php, define routes for your microservice’s functions. For instance, in a user management microservice, you might include routes for user creation, retrieval, and updating. Subsequently, develop controllers to manage these routes.
// Example routes in routes/api.phpRoute::post(‘users’, ‘UserController@store’);Route::get(‘users/{id}’, ‘UserController@show’);Route::put(‘users/{id}’, ‘UserController@update’); // Corresponding controller in app/Http/Controllers/UserController.phpclass UserController extends Controller { // Implement the necessary methods} |
Implementing Business Logic
Within your controllers, implement the specific business logic of your microservice. Utilize Laravel’s features like Eloquent ORM and validation to facilitate this process.
class UserController extends Controller { public function store(Request $request) { // Validate and create a user } // Additional methods} |
Strategies for Deploying and Managing PHP Microservices
Deployment Options
- Docker: Containerize your microservice for deployment on platforms like Kubernetes or Docker Swarm;
- Serverless Platforms: Use platforms like AWS Lambda or Google Cloud Functions with the Bref PHP runtime.
Management Tools
- API Gateways: Manage tasks like load balancing and authentication with tools like Kong or Amazon API Gateway;
- Service Discovery: Enable service communication with tools like Consul or etcd;
- Monitoring: Monitor service health and performance using tools like Prometheus or Datadog.
Comparative Analysis: Traditional vs. Microservice Architecture in PHP
Aspect | Traditional Architecture | Microservice Architecture |
---|---|---|
Design Approach | Monolithic, with all components integrated | Modular, with independent services |
Scalability | Scaled as a whole unit | Services scaled independently |
Flexibility | Changes impact the entire system | Easier to modify or add functionality |
Deployment | Single deployment for the entire application | Independent deployment for each service |
Resilience | Failure can impact the entire system | Isolated failures, limited impact |
Data Management | Shared database for all components | Separate database for each service |
Understanding the PHP POST Method in Form Handling
The Role of POST in PHP
The POST method in PHP is crucial for securely transmitting sensitive data in form submissions. Unlike GET, which appends data to the URL, POST sends data in the request body, hiding it from the browser’s address bar.
Implementing POST in PHP
POST is particularly useful in microservices for handling user authentication, data submission, and other scenarios where data confidentiality is key.
Example of using POST in PHP:
<!– HTML Form –><form action=”submit.php” method=”post”> <label for=”name”>Name:</label> <input type=”text” name=”name” id=”name”> <input type=”submit” value=”Submit”></form> |
// PHP Script (submit.php)$name = $_POST[“name”];echo “Name received: ” . $name; |
Conclusion
This tutorial has provided an in-depth guide to designing, implementing, and managing microservices in PHP, using Laravel as an illustrative framework. By adhering to these practices, you can create scalable, flexible, and resilient PHP microservices. If you need skilled PHP developers, consider utilizing this tutorial as a resource for their training and development.