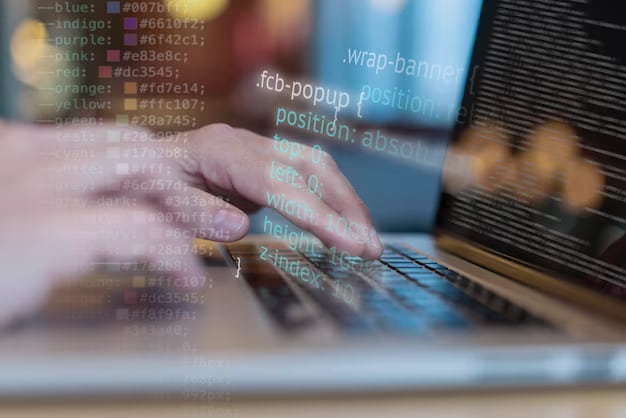
This tutorial embarks on a journey through integrating PHP applications with Microsoft SQL Server. It focuses on advanced functionalities, including CRUD operations, stored procedures, and transaction management, to provide a comprehensive understanding of PHP and MSSQL integration.
Environment Setup for MSSQL in PHP
To utilize MSSQL with PHP, specific prerequisites are necessary:
- PHP (Preferably version 7 or above);
- Microsoft SQL Server (2008 or newer);
- Microsoft ODBC Driver for SQL Server;
- PHP Extensions: SQLSRV and PDO_SQLSRV.
Installation and configuration of these components set the stage for seamless database interactions.
Establishing Database Connectivity in PHP
PHP’s PDO_SQLSRV extension offers a reliable means to connect to MSSQL databases. Here’s an example to establish a connection:
try { $connection = new PDO(“sqlsrv:server=localhost;Database=YourDatabase”, ‘YourUsername’, ‘YourPassword’); $connection->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);} catch (PDOException $e) { echo ‘Connection failed: ‘ . $e->getMessage();} |
Executing CRUD Operations
CRUD operations form the backbone of database interaction in applications. Below are examples of Create, Read, Update, and Delete operations using PHP and MSSQL:
- Create (Insert):
$insertQuery = “INSERT INTO users (username, email) VALUES (:username, :email)”; |
- Read (Select):
$selectQuery = “SELECT * FROM users”; |
- Update:
$updateQuery = “UPDATE users SET email = :email WHERE username = :username”; |
- Delete:
$deleteQuery = “DELETE FROM users WHERE username = :username”; |
Implementing Stored Procedures
Stored procedures enhance performance and simplify SQL management in applications. Example of executing a stored procedure in PHP:
$procedureCall = “EXEC getUserByEmail @Email=:email”; |
Managing Transactions Effectively
Transactions ensure the atomicity of a series of database operations. Here’s an illustration of transaction handling:
try { $connection->beginTransaction(); // Transactional queries $connection->commit();} catch (PDOException $e) { $connection->rollBack(); echo ‘Transaction failed: ‘ . $e->getMessage();} |
Comparative Table: CRUD Operations in PHP with MSSQL
Operation | Description | Example in PHP with MSSQL | Use Case |
---|---|---|---|
Create (Insert) | Adds new data to the database. | php $query = “INSERT INTO users (username, email) VALUES (:username, :email)”; | Adding a new user to the ‘users’ table. |
Read (Select) | Retrieves data from the database. | php $query = “SELECT * FROM users”; | Fetching all user records from the ‘users’ table. |
Update | Modifies existing data in the database. | php $query = “UPDATE users SET email = :email WHERE username = :username”; | Updating the email of a specific user in the ‘users’ table. |
Delete | Removes data from the database. | php $query = “DELETE FROM users WHERE username = :username”; | Deleting a user record from the ‘users’ table. |
Array_Slice in PHP: Enhancing Array Manipulation Capabilities
PHP’s array_slice() function is a powerful tool for developers, enabling them to extract specific parts of arrays efficiently. This function is invaluable for operations requiring modification or extraction of array segments without altering the original array.
Functionality and Usage
array_slice() allows slicing of an array starting at a specified index and for a given length. It’s particularly useful for scenarios where only a portion of an array is needed for processing.
Syntax:
array_slice(array $array, int $offset, int $length = null, bool $preserve_keys = false) |
- $array: The array to be sliced;
- $offset: The starting position of the slice. Positive values start from the beginning, negative values count from the end;
- $length (optional): The number of elements to extract. Absent or null extracts till the end;
- $preserve_keys (optional): When true, original keys are preserved in the sliced array.
Example Use Cases:
- Extracting a subset of an array for displaying a paginated list;
- Removing specific elements from an array for analysis or reporting.
Conclusion
This tutorial has comprehensively covered the aspects of PHP and MSSQL integration, focusing on database connectivity, CRUD operations, the usage of stored procedures, and transaction management. For those seeking expert assistance or looking to hire PHP developers with proficiency in MSSQL, Reintech offers professional support and resources.