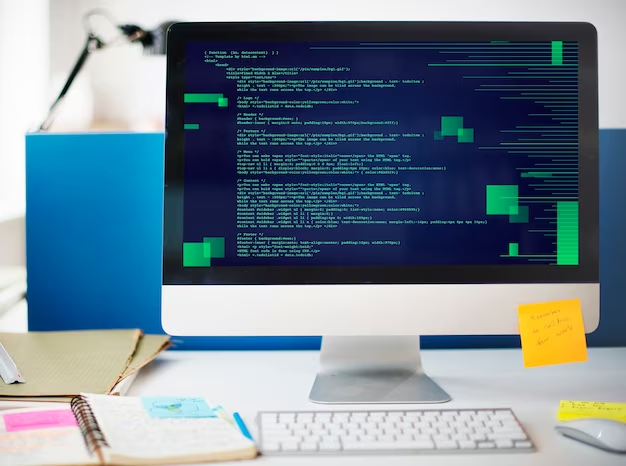
This tutorial focuses on managing PHP forms using the GET and POST methods, coupled with strategies for validating form data. This knowledge is vital for any PHP developer, as it guarantees the security and precision of user-submitted data. By the end of this tutorial, you will gain comprehensive insights into PHP form handling and validation. For those seeking skilled PHP developers, consider engaging with professionals from Reintech.
The GET Method: Data Retrieval in PHP Forms
PHP offers two primary methods for form submission handling: GET and POST. It’s crucial to select the right method based on the specific requirements.
GET Method
GET transmits data via URL query parameters, visible in the browser’s address bar. It’s suitable for simple, non-sensitive information like search terms.
- Example:
<form action=”search.php” method=”get”> <label for=”query”>Search:</label> <input type=”text” name=”query” id=”query”> <input type=”submit” value=”Search”></form> |
- Accessing GET data in PHP:
$query = $_GET[“query”];echo “You searched for: ” . $query; |
The POST Method: Secure Data Submission in PHP Forms
POST Method
POST conveys data within the request body, concealing it from the browser’s address bar. It’s ideal for sensitive data and large submissions.
- Example:
<form action=”login.php” method=”post”> <label for=”username”>Username:</label> <input type=”text” name=”username” id=”username”> <label for=”password”>Password:</label> <input type=”password” name=”password” id=”password”> <input type=”submit” value=”Login”></form> |
- Accessing POST data in PHP:
$username = $_POST[“username”];$password = $_POST[“password”];echo “Welcome, ” . $username . “!”; |
Techniques for Validating PHP Form Data
Required Fields
Use the empty() function to ensure required fields are filled. Display an error if a field is left empty.
- Example:
$error = “”;if ($_SERVER[“REQUEST_METHOD”] == “POST”) { if (empty($_POST[“username”])) { $error = “Username is required”; } else { $username = $_POST[“username”]; }} |
Sanitizing Input Data
Sanitize data to prevent security risks like XSS attacks with htmlspecialchars().
- Example:
function sanitize_input($data) { $data = trim($data); $data = stripslashes($data); $data = htmlspecialchars($data); return $data;} $username = sanitize_input($_POST[“username”]); |
Validating Specific Input Types
Utilize PHP’s inbuilt functions for validating types like emails and URLs.
- Email validation example:
if (!filter_var($_POST[“email”], FILTER_VALIDATE_EMAIL)) { $error = “Invalid email format”;} else { $email = $_POST[“email”];} |
- URL validation example:
if (!filter_var($_POST[“website”], FILTER_VALIDATE_URL)) { $error = “Invalid URL”;} else { $website = $_POST[“website”];} |
Comparative Analysis: GET vs. POST Methods and fopen() Usage
Feature | GET Method | POST Method | fopen() Function |
---|---|---|---|
Purpose | Data retrieval via URL | Secure data submission | File operations |
Visibility | Visible in URL | Hidden in request body | N/A |
Data Size | Limited by URL length | Suitable for large data | Depends on file size and mode |
Use Cases | Non-sensitive data (e.g., searches) | Sensitive data (e.g., logins) | File reading, writing, editing |
Additional Info | Bookmarkable | More secure | Requires proper file handling |
Introduction to PHP File Operations with fopen()
The fopen() function in PHP is a powerful tool for file handling, allowing developers to open
, read, write, and manipulate files. Understanding fopen() is crucial for managing file-related tasks in PHP applications.
fopen() in PHP
fopen() opens a file or URL and returns a file pointer resource on success or FALSE on failure. It requires two parameters: the filename and the mode of operation (e.g., r for read, w for write).
- Example of using fopen():
$file = fopen(“example.txt”, “r”) or die(“Unable to open file!”);// Read or write operationsfclose($file); |
This snippet demonstrates opening “example.txt” for reading. It’s important to close the file after operations using fclose().
Conclusion
This tutorial covered PHP form handling with GET and POST methods and various data validation techniques. By implementing these methods, you can ensure your PHP forms are both secure and accurate. For professional PHP development assistance, consider partnering with Reintech’s PHP experts.