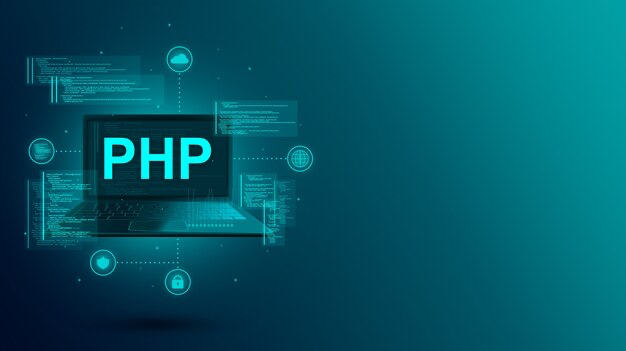
In this guide, we’ll explore the fundamentals of socket programming and its implementation in PHP, essential for network programming. Aimed at software developers, this tutorial offers a comprehensive understanding of how PHP can be used for network programming tasks.
Getting Started with Sockets
Sockets facilitate communication between computers across a network. They are a crucial component in network programming, enabling the exchange of data between devices. Within PHP, sockets establish a connection between a server and a client, making it possible to transfer data to and fro.
Getting Acquainted with Socket Functions in PHP
PHP offers a rich library of in-built functions tailored for socket interaction, presenting developers with a powerful toolkit for their applications. Let’s delve deeper into some of these critical functions:
- socket_create(): This function is akin to crafting a new digital bridge. It instantiates a new socket that can then be configured for communication;
- socket_bind(): Think of this function as assigning a mailing address to your digital bridge. It binds the newly created socket to a specific IP address and port, providing a unique identity for the socket in the network ocean;
- socket_listen(): With this function, the socket begins its vigil, keeping its ears open for any incoming connection requests from client applications;
- socket_accept(): This function is the gatekeeper. When a client application knocks, socket_accept() is responsible for opening the gate, accepting the client’s connection request;
- socket_read(): The function serves as the receiver of data packages that are being sent over the socket;
- socket_write(): This function is the flip side of socket_read(). It’s the function that enables sending or writing data to the socket, ready to be picked up by the receiving end;
- socket_close(): After the communication has concluded, this function rolls down the shutter, terminating the socket connection.
The correct and efficient use of these functions in PHP can result in high-performing and robust network services.
Creating a Socket Server in PHP
To create a socket server in PHP, follow these steps:
- Fabricate a New Socket: This is the first step on the road to creating a socket server. We can use socket_create() function in PHP for this purpose;
- Deploy the Socket: After the creation, the socket must be deployed to a specific address and port. It’s like setting up a unique address for your postal office in the digital world. The socket_bind() function comes in handy for this;
- Standby for Connections: Next, it’s time for the socket to start listening for incoming connections. It’s akin to having a receptionist waiting for incoming calls. PHP provides the socket_listen() function for this role;
- Embrace Incoming Connections: The socket now needs to accept incoming connections. Imagine opening the door when a guest arrives. socket_accept() function does exactly that for your socket server;
- Digest Client-sent Data: Once a connection is established, the socket server must then read the data sent from the client – a task performed by the socket_read() function;
- Respond to the Client: The socket server can also transmit data back to the client. This resembles writing a reply letter to the client in our postal office analogy. socket_write()function serves this purpose;
- Seal the Connection: Finally, the socket server must close the connection after the communication is finished, a task accomplished by the socket_close() function.
Let’s decode the construction of a basic socket server in PHP using an illustrative example:
$host = '127.0.0.1';
$port = 9999;
// Fabricate a new socket
$socket = socket_create(AF_INET, SOCK_STREAM, 0);
// Deploy the socket to a specific address and port
socket_bind($socket, $host, $port);
// Standby for incoming connections
socket_listen($socket);
// Embrace an incoming connection
$client = socket_accept($socket);
// Digest data from the connected client
$data = socket_read($client, 1024);
// Respond to the client
socket_write($client, 'Server: ' . $data);
// Seal the connection
socket_close($client);
socket_close($socket);
Creating a Socket Client in PHP
To create a socket client in PHP, follow these steps:
- Birthing a New Socket: The socket_create() function commences this journey, creating a new socket;
- Initiating Contact with the Server: The socket_connect() function serves to reach out to the server, initiating a connection;
- Dispatching Data to the Server: Once the connection is made, data is sent to the server using socket_write();
- Receiving Server’s Response: The client now anticipates the server’s response, which is read using the socket_read() function;
- Terminating the Connection: At the end of the conversation, the connection needs to be terminated, and socket_close() takes care of this.
Now, let’s bring these steps to life with a cohesive example:
$host = '127.0.0.1';
$port = 9999;
// Birthing a new socket
$socket = socket_create(AF_INET, SOCK_STREAM, 0);
// Initiating contact with the server
socket_connect($socket, $host, $port);
// Dispatching a message to the server
socket_write($socket, 'Hello, Server!');
// Waiting for the server's response
$data = socket_read($socket, 1024);
// Echoing the server's response
echo "Received from server: $data\n";
// Terminate the connection
socket_close($socket);
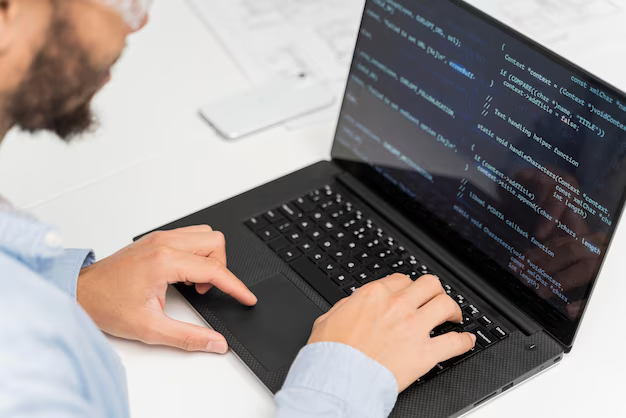
Data Exchange Between Client and Server
After establishing a connection between a client and a server, data transfer is possible using the socket_write() and socket_read() functions. In the provided examples, the server replicates and sends back the data received from the client. Nonetheless, depending on the specific needs of your application, more sophisticated communication methods can be implemented.
Managing Errors in Socket Programming
In socket programming, managing errors effectively is crucial. Utilize functions like socket_last_error() and socket_strerror() to gain insights into any issues that arise while using socket functions. Consider the following example:
$socket = socket_create(AF_INET, SOCK_STREAM, 0);
if ($socket === false) {
$errorCode = socket_last_error();
$errorMessage = socket_strerror($errorCode);
echo "Error in socket creation: $errorMessage\n";
}
It is a best practice to incorporate error handling for all socket-related functions. This ensures that your application remains robust and functions correctly, even when facing network-related problems.
Wrapping Up
This guide has taken you through the fundamental aspects of socket programming in PHP. We’ve explored the creation of socket servers and clients and delved into their communication mechanisms via sockets. This foundational understanding equips you with the skills necessary to craft robust network applications using PHP. Remember, when developing your applications, it’s crucial to engage PHP developers skilled in socket programming.