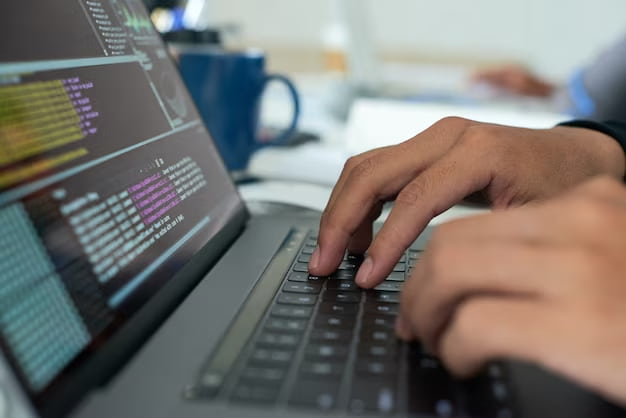
This tutorial focuses on mastering PHP’s sleep() and usleep() functions, essential for introducing time delays in PHP scripts. Such delays are crucial for simulating real-time processes or controlling script execution durations.
Exploring the sleep() Function
The sleep() function in PHP is designed to halt script execution for a set number of seconds. It accepts an integer parameter representing the pause duration in seconds and typically returns zero upon successful completion.
Example Usage of sleep():
echo “Start: ” . date(“h:i:s”) . PHP_EOL;sleep(3); // Halts execution for 3 secondsecho “End: ” . date(“h:i:s”) . PHP_EOL; |
Executing this script results in a three-second pause between the “Start” and “End” outputs.
Delving into the usleep() Function
The usleep() function offers more granular control over script pauses, allowing delays specified in microseconds. This function is particularly useful for precise timing requirements.
Example Usage of usleep():
echo “Start: ” . microtime(true) . PHP_EOL;usleep(500000); // Halts execution for 0.5 secondsecho “End: ” . microtime(true) . PHP_EOL; |
Running this script introduces a half-second delay in the execution.
Practical Application: Managing API Request Rates
A common use case for sleep() and usleep() is managing the rate of API requests. For APIs with request rate limits, these functions can be strategically used to prevent limit breaches.
Example for API Rate Limiting:
for ($i = 0; $i < 60; $i++) { // API request logic sleep(1); // Introduces a 1-second delay between requests} |
This approach helps to maintain compliance with API rate limits, such as 60 requests per minute.
PHP MSSQL Examples: Practical Implementation Scenarios
In this section, we explore various examples illustrating how PHP can be used to connect and interact with Microsoft SQL Server databases, covering scenarios from basic database operations to more complex transactions.
Example 1: Connecting to MSSQL Database
$serverName = “serverName\\sqlexpress”; //serverName\instanceName$connectionInfo = array(“Database”=>”dbName”, “UID”=>”userName”, “PWD”=>”password”);$conn = sqlsrv_connect($serverName, $connectionInfo); if ($conn) { echo “Connection established.”;} else { echo “Connection could not be established.”; die(print_r(sqlsrv_errors(), true));} |
This example establishes a connection to an MSSQL database using the sqlsrv_connect function.
Example 2: Executing a Simple Query
$query = “SELECT * FROM users”;$stmt = sqlsrv_query($conn, $query); while ($row = sqlsrv_fetch_array($stmt, SQLSRV_FETCH_ASSOC)) { echo $row[‘username’] . “, ” . $row[’email’] . “<br />”;} |
This snippet retrieves user data from the ‘users’ table, iterating over the results.
Example 3: Inserting Data
$query = “INSERT INTO users (username, email) VALUES (?, ?)”;$params = array(“newUser”, “[email protected]”); $stmt = sqlsrv_query($conn, $query, $params);if ($stmt === false) { die(print_r(sqlsrv_errors(), true));} |
This example demonstrates inserting data into the ‘users’ table.
Conclusion
This guide has elucidated the use of PHP’s sleep() and usleep() functions for implementing time delays in scripts. These functions are invaluable for scenarios like simulating real-time operations or managing API request rates, reinforcing their importance in PHP development. For projects requiring intricate timing control, consider engaging PHP developers well-versed in these functionalities.