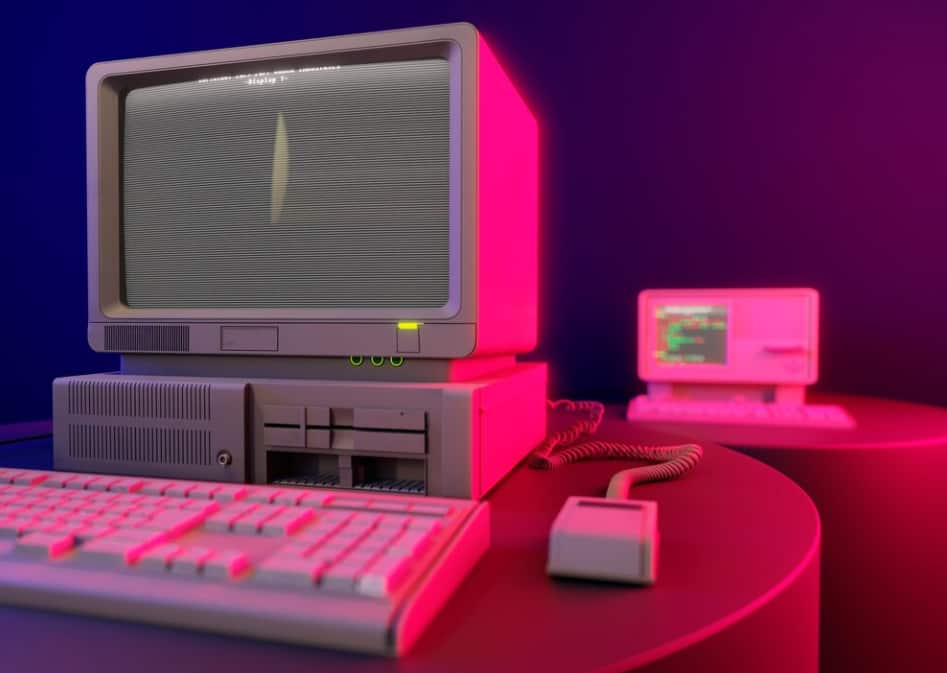
WebSockets for Real-Time Communication in Web Applications
WebSockets provide a significant enhancement to web application capabilities by enabling real-time, two-way communication between web clients and servers. They are essential for developers who aim to create highly interactive web applications. These applications include features such as live chat, real-time alerts, online gaming, and collaborative tools, all of which update and respond in real-time without needing to reload the webpage.
In contrast to the traditional HTTP connections, which are stateless and close after each transaction, WebSockets establish a continuous connection. This connection stays open, facilitating an ongoing exchange of data. This feature is extremely useful for real-time web applications that require a constant flow of data, such as financial trading platforms or live sports score updates.
Moving on from the topic of PHP WebSockets, let’s now focus on a basic yet essential feature of PHP – the array_values function, which is key for handling arrays in PHP applications.
Implementing WebSockets in PHP Applications
PHP, primarily used for server-side scripting, lacks built-in support for managing persistent WebSocket connections, a limitation due to its stateless design. Despite this, PHP developers can successfully integrate WebSocket servers in their applications by using specific libraries like Ratchet or Thruway. These libraries provide the necessary infrastructure for enabling real-time communication in PHP, thereby overcoming its inherent limitations.
Among these, Thruway is particularly advantageous for PHP development. It is designed for ease of use and is compatible with the Web Application Messaging Protocol (WAMP). WAMP extends the capabilities of WebSockets by adding more complex communication patterns, including publish/subscribe mechanisms and remote procedure calls (RPC). This functionality enhances WebSockets, allowing for more sophisticated real-time interactions in PHP applications, such as continuous data streams and interactive user interfaces. The integration of these technologies transforms PHP into a more capable platform for developing modern web applications that require real-time data communication.
Setting Up Thruway for WebSocket Server in PHP Projects
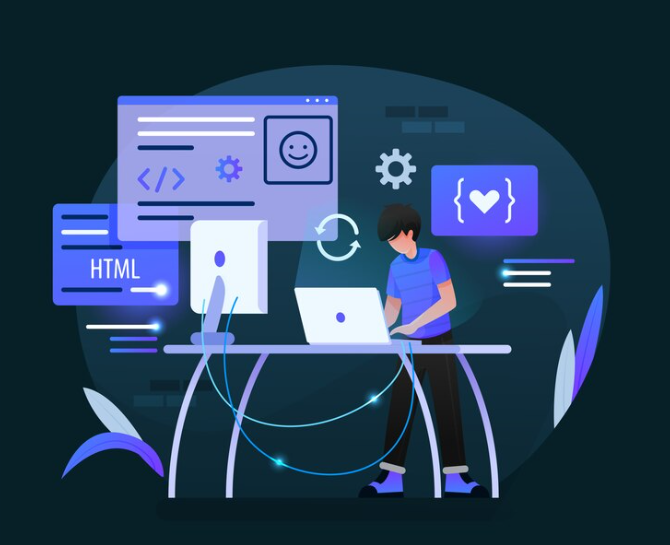
To begin using Thruway in your PHP project, the first step is to install it using Composer, PHP’s dependency manager. Execute the following command in the root directory of your project:
composer require voryx/thruway
After installing Thruway, you can set up a WebSocket server. This involves writing a PHP script to initiate the Thruway server and manage WebSocket connections:
<?php
use Thruway\ClientSession;
use Thruway\Peer\Router;
use Thruway\Transport\RatchetTransportProvider;
$router = new Router();
$transportProvider = new RatchetTransportProvider('127.0.0.1', 8080);
$router->addTransportProvider($transportProvider);
$router->start();
?>
This script represents a basic configuration. In a real-world application, further development is needed. You should create event handlers and develop business logic that allows interaction with clients through the WebSocket connection.
Implementing Real-Time Features in PHP with Thruway Server
Once your server is operational, you can start integrating real-time functionalities into your PHP application.
- For instance, if you’re building a live chat application, you’d set up event handlers;
- These handlers are designed to distribute messages to all connected clients whenever a new message comes in;
- Thruway simplifies this process with its publish/subscribe messaging pattern.
Below is a basic example of how you could manage incoming chat messages:
<?php
// ... Initialization code as before
$router->getEventDispatcher()->addListener('message.received', function ($event) {
// Broadcast message to all subscribed clients
$event->session->publish('chat.channel', [$event->message], [], ["acknowledge" => true]);
});
// ... The rest of the server code
?>
This section of code establishes a listener for the ‘message.received’ event. Upon the event’s activation, it broadcasts the message to the ‘chat.channel’ topic. Subscribed users will then be able to receive and view these messages, facilitating live updates within the chat application.
Connecting to a PHP WebSocket Server Using JavaScript
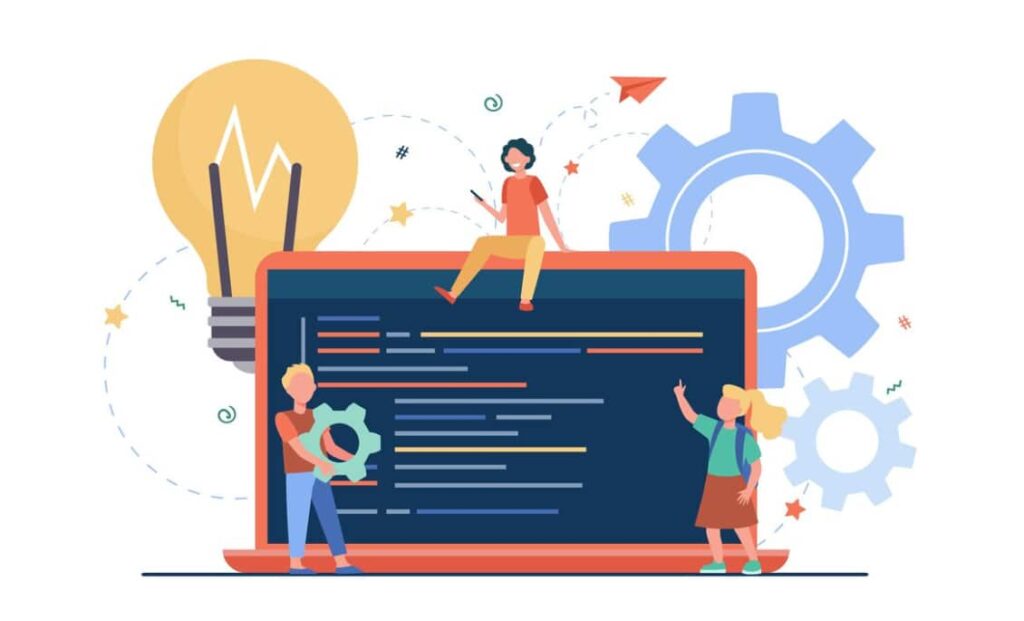
In the realm of client-side development, JavaScript plays a pivotal role in establishing a WebSocket connection with a PHP server. Developers have the option of utilizing the native WebSocket API, which provides fundamental capabilities for establishing and managing connections, or they can opt for more sophisticated libraries such as AutobahnJS. AutobahnJS offers an array of advanced features, including automatic reconnection mechanisms, support for WebSocket subprotocols, and robust error handling, making it well-suited for handling complex applications.
- JavaScript’s core responsibilities within this framework encompass the initiation and maintenance of the WebSocket connection with the PHP server, a fundamental element for ensuring continuous and real-time data exchange;
- Moreover, it manages the process of subscribing to various topics or channels made available by the server, allowing the client to selectively receive targeted data streams;
- Furthermore, JavaScript is responsible for transmitting messages or data back to the WebSocket server, facilitating seamless bidirectional communication.
This framework is integral for developing a variety of real-time applications, such as interactive chat systems, live notification services, real-time updates in web games, and collaborative online tools. JavaScript’s flexibility, combined with WebSocket’s real-time capabilities, provides a powerful solution for incorporating real-time features in PHP-based web applications, catering to a range of interactive and dynamic user experiences.
Conclusion
Utilizing WebSockets and Thruway together offers a solid framework for integrating real-time features into PHP applications. However, as your application scales to handle numerous concurrent connections, it’s advisable to think about incorporating a message broker like RabbitMQ or Redis. This will help efficiently manage the increased traffic.
Moreover, security should be a top priority for your application. Implementing WebSocket security best practices, such as thorough validation of input data, is essential to minimize potential vulnerabilities. Additionally, using WebSocket Secure (wss) for encrypted connections is crucial for enhancing your application’s security and protecting its users.