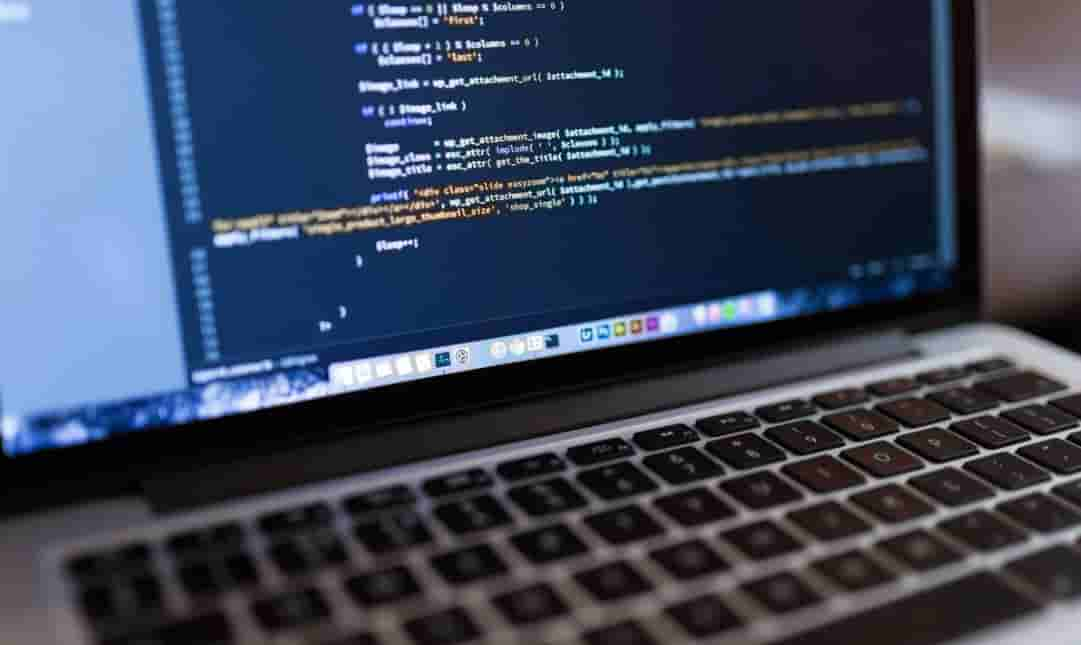
This comprehensive guide delves into the varied techniques of file manipulation using PHP. It highlights PHP’s robust set of functions for reading, writing, and uploading files, emphasizing its suitability for developers frequently dealing with file operations. By the conclusion of this guide, the reader will possess a thorough understanding of PHP’s capabilities in file handling. For those considering hiring PHP developers, Reintech comes highly recommended.
Initial Steps: Opening and Closing Files
File manipulation in PHP is a cornerstone of effective file management and data processing in web applications. The initial step in this process is to open the file using PHP’s `fopen()` function. This function is versatile, allowing developers to specify the mode in which the file should be opened, depending on the required operation. Common modes include ‘r’ for reading, ‘w’ for writing, ‘a’ for appending, and ‘x’ for creating a new file if it doesn’t already exist. For example, to open a file for reading, one would use:
```php
$file = fopen("example.txt", "r");
```
This line of code opens “example.txt” in read mode. After performing the necessary file operations, it is crucial to close the file using the `fclose()` function. Closing the file is not just a good practice but a necessary one. It ensures that all the resources tied to the file are properly released, and any data written to the file is properly saved. This step is crucial in maintaining data integrity and preventing resource leaks, which can affect the performance and stability of the application. Moreover, proper closing of files reinforces secure coding practices, an essential aspect in today’s data-sensitive programming environment.
Techniques for Reading Files
When it comes to reading files in PHP, developers have a suite of functions at their disposal, each tailored to different needs. The `fread()` function, for instance, is particularly useful when precise control over the amount of data read is required. It allows developers to specify the exact number of bytes to read from the file, making it ideal for handling large files or binary data.
The `fgets()` function, on the other hand, is optimized for reading files line by line. This makes it an excellent choice for processing text files where data is structured in lines, such as log files or configuration files. It simplifies the process of parsing each line individually, enhancing the readability and maintainability of the code.
In contrast, the `file_get_contents()` function is designed for simplicity and convenience, allowing for the entire content of a file to be read and returned as a single string. This is particularly advantageous when dealing with small to medium-sized files where the entire content is needed in one operation. It eliminates the need for the initial file opening and closing steps, streamlining the file-reading process.
Summarizing the different PHP functions for reading files:
`fread()` Function
- Use Case: Ideal for precise control over the amount of data read;
- Functionality: Allows specification of the exact number of bytes to read;
- Ideal For: Large or binary files where controlled, chunk-based reading is necessary.
`fgets()` Function
- Use Case: Optimized for reading files line by line;
- Functionality: Simplifies parsing each line individually;
- Ideal For: Text files structured in lines, like log files or configuration files.
`file_get_contents()` Function
- Use Case: Designed for simplicity and convenience;
- Functionality: Reads and returns the entire content of a file as a single string;
- Ideal For: Small to medium-sized files where entire content is needed at once.
These functions collectively provide PHP developers with a flexible toolkit for handling various file reading scenarios. Each function serves distinct purposes, from detailed, byte-level reading with `fread()`, to efficient line-by-line processing with `fgets()`, and straightforward full-file reading with `file_get_contents()`. This versatility is one of the reasons PHP remains a popular choice for server-side scripting, especially in applications where file manipulation is a frequent requirement.
Strategies for Writing to Files
In PHP, writing to files is a crucial functionality, and it is facilitated by functions like `fwrite()` and `file_put_contents()`. The `fwrite()` function is ideal for scenarios where there’s a need for appending or modifying data in a file incrementally. It offers greater control over what data is written and where it’s placed in the file. This precision makes `fwrite()` particularly useful for tasks like logging or updating specific parts of a file.
On the other hand, `file_put_contents()` is designed for convenience and simplicity, allowing developers to write data to a file in one straightforward operation. This function is particularly useful for overwriting a file with new content or creating new files with specific content. Its ease of use makes it a preferred choice for scenarios where the complexity of incremental writing is unnecessary, such as generating configuration files or storing simple data structures.
Together, these writing functions in PHP provide a comprehensive toolset, catering to both complex file manipulation tasks that require fine-grained control, and simpler, more direct file writing needs. This flexibility is a testament to PHP’s capability as a scripting language, particularly for backend development where file operations are a common requirement.
Uploading Files with PHP
Handling file uploads in PHP necessitates an HTML form set to `multipart/form-data` and a file input element. The PHP script then uses the `$_FILES` superglobal and `move_uploaded_file()` function to process the uploaded file.
Wrapping Up: Gaining Mastery in PHP File Operations
This guide provides insights into opening, closing, reading, writing, and uploading files using PHP. PHP’s comprehensive file operation functions make it an appealing choice for developers. For expert assistance in PHP development, consider exploring Reintech’s offerings.