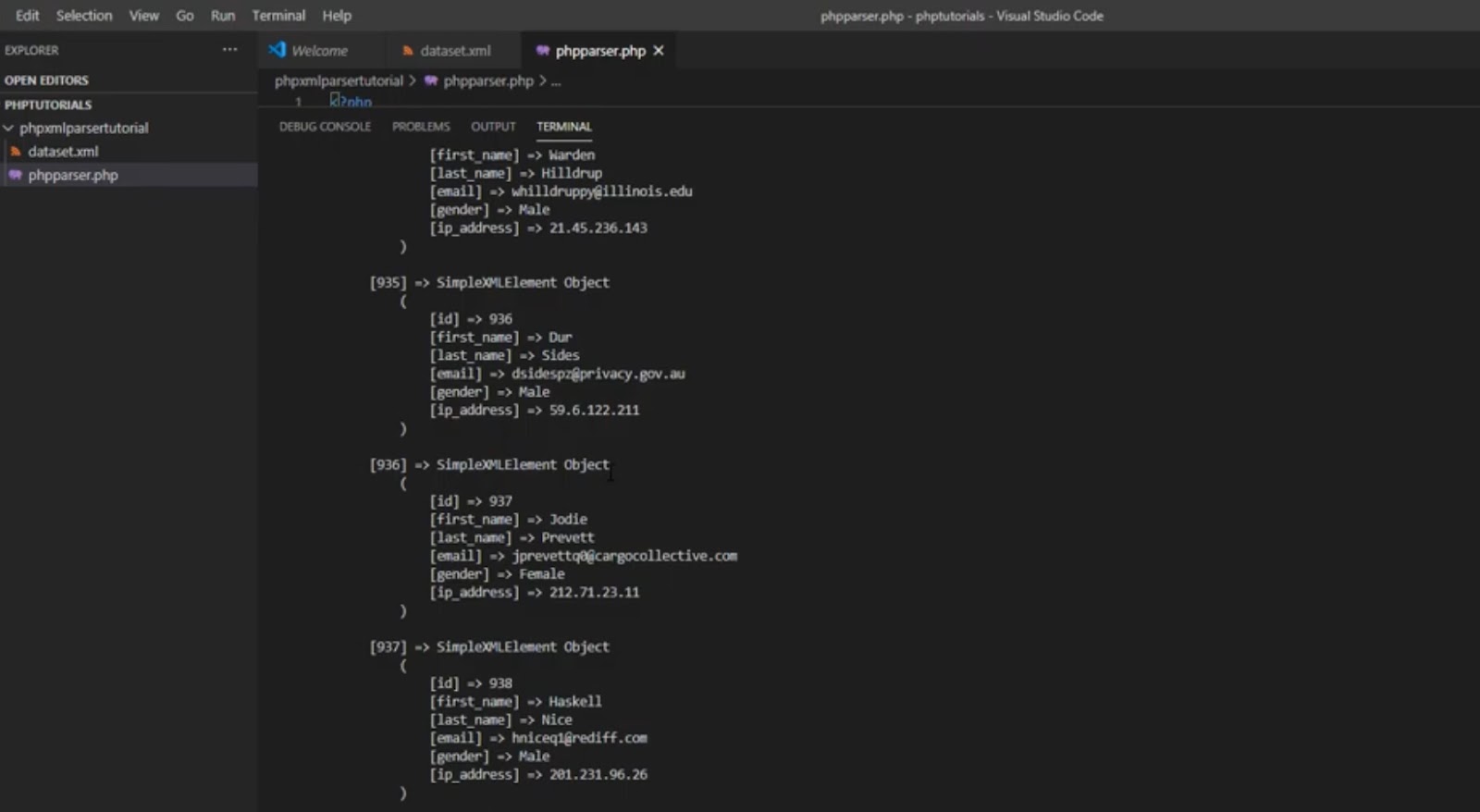
In this detailed tutorial, software developers will embark on an educational journey to master the intricacies of working with PHP and XML. This guide is a treasure trove of knowledge, encompassing the essentials of parsing, validation, and transformation of XML using PHP. XML, a ubiquitous format for data storage and exchange on the web, can be adeptly handled and modified using PHP. This tutorial is not just a learning pathway but also a crucial resource for those seeking to employ PHP developers skilled in XML manipulation.
Parsing XML Using PHP
When it comes to parsing XML in PHP, two prevalent techniques emerge: SimpleXML and XMLReader.
The SimpleXML Approach
Included in PHP, SimpleXML is a user-friendly extension designed to simplify interactions with XML data. It transforms XML into an object, facilitating its processing with standard object-oriented programming methods.
Loading XML Data via SimpleXML
To initiate XML data loading with SimpleXML, one may utilize either `simplexml_load_string()` or `simplexml_load_file()` functions. Here’s how they work:
```php
$xml_string = '<root><element>Hello, World!</element></root>';
$xml = simplexml_load_string($xml_string);
$xml = simplexml_load_file('example.xml');
```
Navigating XML Elements and Attributes in SimpleXML
SimpleXML allows for the effortless navigation of XML elements and attributes using standard object notation:
```php
echo $xml->element; // Accessing an element
echo $xml->element['attribute']; // Accessing an attribute
```
Delving into XMLReader
XMLReader offers a more sophisticated and efficient approach for XML parsing in PHP. Being stream-based, it is particularly adept at handling large XML files.
Employing XMLReader for XML Data Loading
The `XMLReader::open()` function is the gateway to loading XML data in XMLReader:
```php
$reader = new XMLReader();
$reader->open('example.xml');
```
The Process of Reading and Handling XML Data with XMLReader
XMLReader allows for the sequential reading and processing of XML data, typically through a while loop:
```php
while ($reader->read()) {
// Processing logic here
}
```
Validating XML with PHP
PHP serves as a powerful tool for XML data validation against XML schemas, ensuring the data adheres to specific structures and formats.
Utilizing DOMDocument for XML Validation
The DOMDocument class in PHP is instrumental in XML validation. The `schemaValidate()` method checks the XML against a given schema:
```php
$dom = new DOMDocument();
$dom->load('example.xml');
if ($dom->schemaValidate('schema.xsd')) {
echo 'XML is valid';
} else {
echo 'XML is not valid';
}
```
Transforming XML with PHP
PHP also excels in transforming XML data into various formats, like HTML, through XSLT.
Preparing XML and XSL Data for Transformation
The DOMDocument class is used to load both XML data and XSL stylesheets:
```php
$xml = new DOMDocument();
$xml->load('example.xml');
$xsl = new DOMDocument();
$xsl->load('stylesheet.xsl');
```
The Transformation Process Using XSLTProcessor
The XSLTProcessor class in PHP facilitates the application of XSLT stylesheets to XML data:
```php
$processor = new XSLTProcessor();
$processor->importStylesheet($xsl);
$result = $processor->transformToXML($xml);
echo $result;
```
With this comprehensive guide, software developers now possess a robust understanding of how to effectively leverage PHP with XML for parsing, validation, and transformation. It’s an invaluable tool for those looking to augment their team with PHP developers who have XML expertise.
Conclusion
In summary, this guide has meticulously explored the realms of parsing, validating, and transforming XML data using PHP, providing a rich resource for software developers. SimpleXML and XMLReader stand as pivotal tools for parsing, DOMDocument shines in validation, and XSLTProcessor excels in transformation. This knowledge not only enhances a developer’s skillset but also broadens their capabilities in managing web data efficiently. As an essential reference, this tutorial is invaluable for those intending to hire PHP developers with XML proficiency, ensuring a solid foundation in these critical aspects of web development.