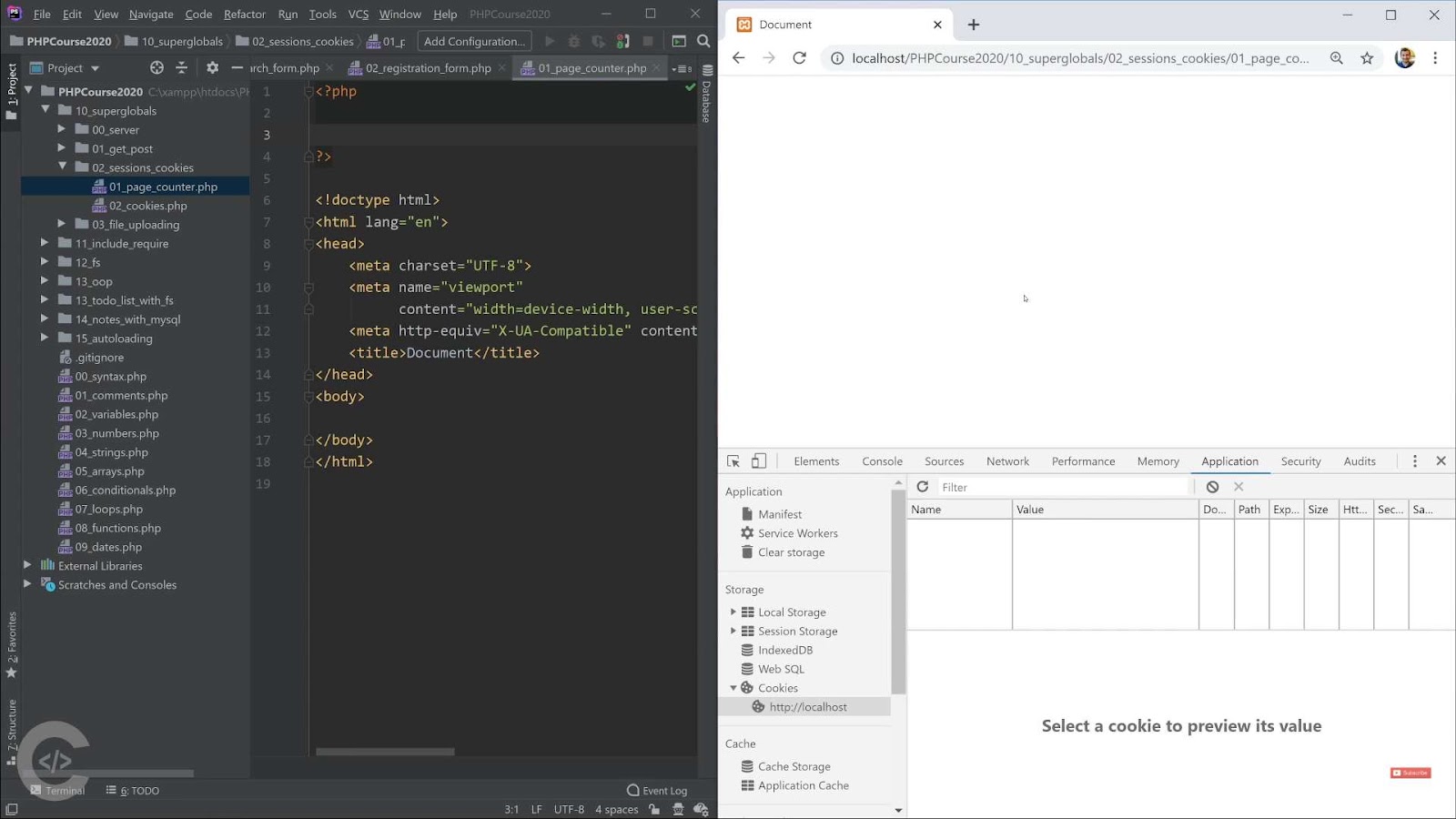
In this guide, we shall delve into the significance of sessions and cookies within PHP, exploring strategies for their efficient administration. Sessions and cookies constitute indispensable elements within the realm of web development, particularly in the context of user authentication and the seamless preservation of user-specific information across the entire application. If you are contemplating the recruitment of PHP developers, it is imperative to possess a profound comprehension of sessions and cookies.
Understanding Sessions and Cookies: Enhancing User Experience
In the realm of web development, sessions and cookies are indispensable tools for enhancing user experiences by maintaining user-specific data across various interactions with a website. These mechanisms, though distinct, play harmoniously to ensure a seamless journey for every visitor. Let’s dive deeper into each of these concepts to grasp their significance:
Cookies: The Crumbs of User Data
Cookies are like the breadcrumbs that web browsers leave behind as you navigate through the internet. These small text files contain essential key-value pairs of data that help websites remember specific user preferences and settings. Think of them as the personalized touch that makes your online experience smoother. Here’s a more comprehensive look at cookies:
- Data Storage: Cookies hold data that ranges from user preferences, such as language settings, to shopping cart contents, login credentials, and even tracking information for online advertising;
- Lifespan: Cookies have an expiration date, which can be set by web developers. They can persist throughout a user’s session (session cookies) or remain stored on the user’s device for an extended period (persistent cookies);
- Functionality: Cookies are sent with every HTTP request made to a server. This constant exchange of cookie data allows websites to recognize users and maintain their unique states;
- Security Measures: While cookies are generally harmless, there are concerns about privacy and security. Developers must use them responsibly to avoid vulnerabilities or data breaches.
Sessions: The Backbone of Server-Side Storage
Sessions, on the other hand, serve as the robust backbone of server-side storage for user-specific information. They are the secret sauce behind personalized experiences that are not reliant solely on the user’s browser. Here’s a closer look at sessions:
- Unique Session ID: When a user visits a website, the server generates a unique session ID for that specific interaction. This session ID serves as the key to accessing the user’s session data;
- Storage Location: Contrary to cookies, which reside on the client-side, session data is securely stored on the server. The session ID, however, is often saved as a cookie on the user’s device to facilitate communication;
- Enhanced Security: Sessions offer a higher level of security compared to cookies because sensitive user information is kept away from prying eyes on the client-side;
- Flexibility: Web developers can tailor sessions to their specific needs, allowing for the storage of complex data structures and user states.
Mastering Cookie Management in PHP
Cookies are invaluable tools in web development, and PHP offers a straightforward way to create, manage, and utilize them effectively. Whether you want to personalize user experiences or store session information, mastering the art of cookies can significantly enhance your web applications. In this comprehensive guide, we’ll delve into creating and managing cookies in PHP, equipping you with the knowledge and skills to take full advantage of this powerful feature.
Creating Cookies with setcookie() Function
To begin harnessing the power of cookies, you’ll want to start by creating them using PHP’s setcookie() function. This versatile function allows you to set various parameters to control the behavior of your cookies. Let’s break down how to use it effectively:
<?php
// Create a basic cookie with a name, value, expiration time, and path
setcookie('user_name', 'John Doe', time() + 86400, '/');
?>
Parameters accepted by setcookie() function:
- Cookie name: A unique identifier for your cookie;
- Cookie value: The data you want to store in the cookie;
- Expiration time: Set the cookie’s expiration time in seconds since the Unix epoch. In the example above, we’ve set it to expire in 24 hours (86400 seconds);
- Path (optional): Define the path to restrict the cookie’s scope. By default, it applies to the current directory (‘/’);
- Domain (optional): Specify the domain where the cookie is accessible, which is often left to default (current domain);
- Secure (optional): If set to true, the cookie will only be transmitted over secure (HTTPS) connections;
- HttpOnly (optional): When true, the cookie can only be accessed through HTTP and not via JavaScript, enhancing security.
Reading Cookie Values with $_COOKIE Superglobal
Once you’ve created cookies, you’ll often need to retrieve their values. PHP simplifies this process by using the $_COOKIE superglobal array. Here’s how to access and utilize cookie values:
<?php
if (isset($_COOKIE['user_name'])) {
echo "User name: " . $_COOKIE['user_name'];
} else {
echo "User name not set.";
}
?>
Deleting Cookies Effectively
Sometimes, you’ll need to remove cookies, whether it’s for logging users out or clearing stored data. The process is straightforward—set the cookie’s expiration time to a value in the past:
<?php
// Delete the 'user_name' cookie by setting its expiration time in the past
setcookie('user_name', '', time() - 3600, '/');
?>
Mastering Session Management in PHP
Sessions play a pivotal role in web development, allowing you to maintain user-specific data across multiple pages. In PHP, session management is made easy with built-in functions. Let’s dive into creating and managing sessions, exploring not only the basics but also some valuable tips and best practices.
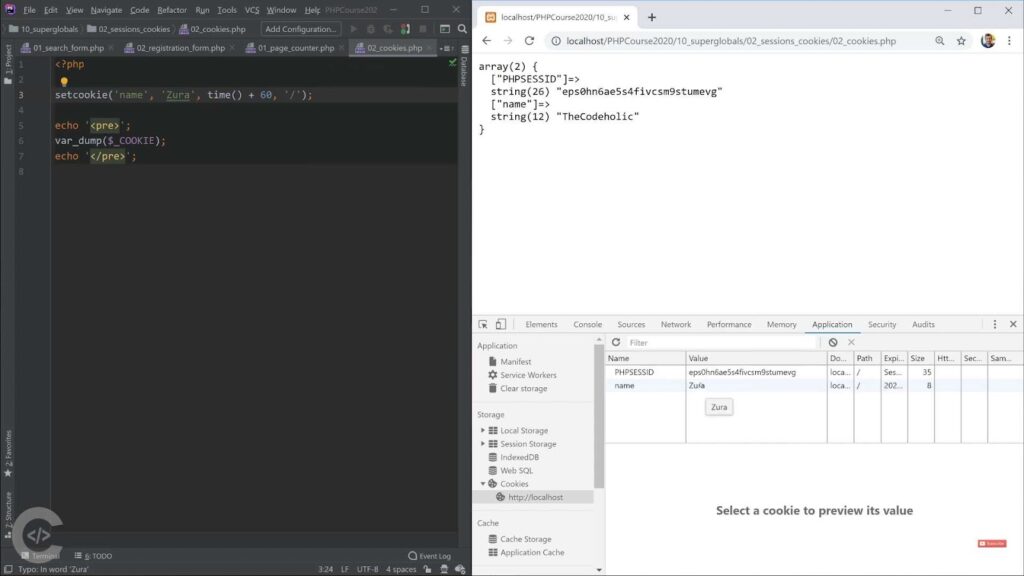
Starting a PHP Session
To initiate a session in PHP, you need to use the session_start() function. This function should be called at the very beginning of each page that requires session functionality. Here’s how to do it:
<?php
session_start();
?>
Storing Data in Sessions
Once a session is started, you can store user-specific data using the $_SESSION superglobal array. This array allows you to save variables that persist throughout the user’s session:
<?php
session_start();
$_SESSION['user_id'] = 1;
$_SESSION['user_name'] = 'John Doe';
?>
Retrieving Session Data
Reading session data is as straightforward as accessing values in the $_SESSION array:
<?php
session_start();
echo "User ID: " . $_SESSION['user_id'] . "<br>";
echo "User name: " . $_SESSION['user_name'];
?>
Deleting Session Variables
To remove a specific session variable, utilize the unset() function:
<?php
session_start();
unset($_SESSION['user_name']);
?>
Destroying a Session
When you want to end a user’s session entirely, the session_destroy() function comes to your rescue:
<?php
session_start();
session_destroy();
?>
Conclusion
In this comprehensive guide, we’ve delved into the core principles of handling sessions and cookies within PHP. These fundamental concepts lie at the heart of crafting a smooth user journey, upholding user data persistence, and fortifying the safeguarding measures for your application. Bear these invaluable insights in your memory and embark on an adventurous exploration of the more intricate aspects to elevate your prowess in the realm of PHP session and cookie administration.