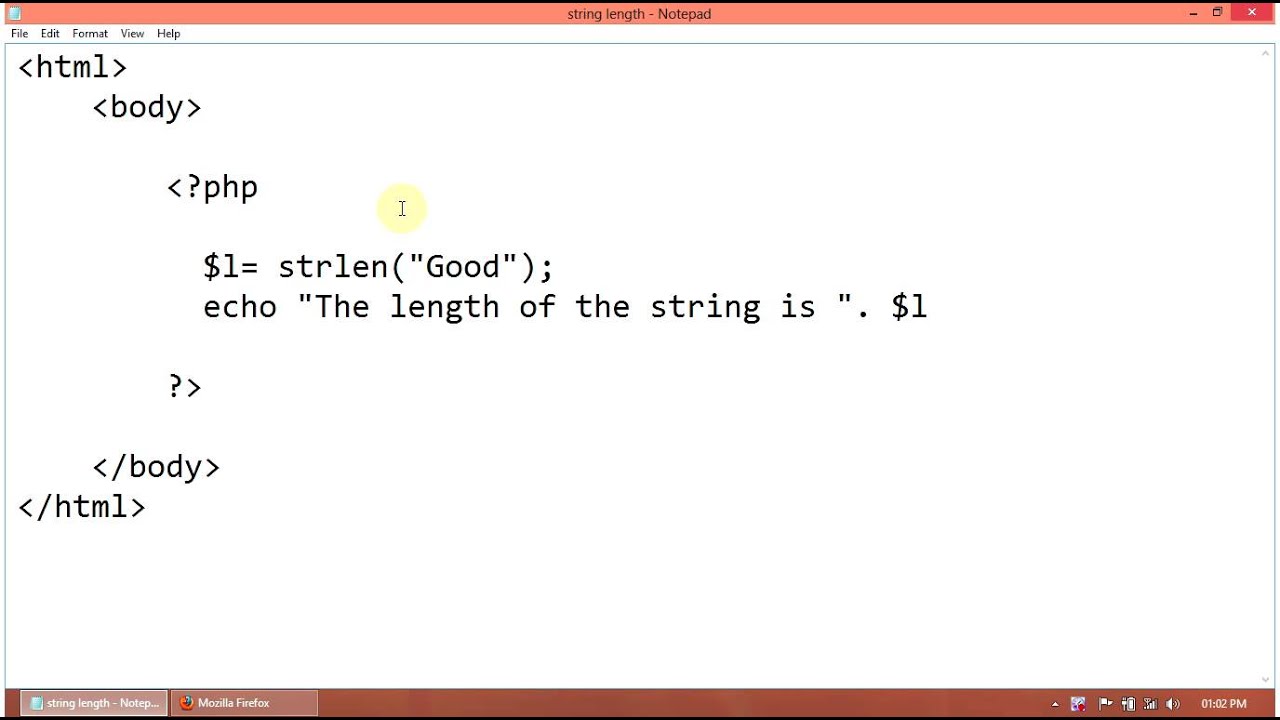
Understanding how to manipulate and determine the length of strings is a fundamental skill in PHP programming. Mastering string length functions is crucial whether you’re validating user input, truncating text, or performing complex string operations.
Getting Started
PHP’s simplest method of determining how long a string is is strlen(). This function returns the number of characters in a given string. Let’s start with a simple example:
php
<?php $str = "Hello, PHP!"; $length = strlen($str); echo "The length of the string is: " . $length; ?>
This will output: “The length of the string is: 12”. Keep in mind that strlen() counts each byte, not necessarily each visible character, so for multibyte character encodings, consider using mb_strlen().
Multibyte strings
When working with multibyte character encodings like UTF-8, mb_strlen() is essential for accurate string length calculation. This function considers the character encoding and provides a more precise result compared to strlen(). Here’s an example:
php
<?php $str = "こんにちは, PHP!"; $length = mb_strlen($str, 'UTF-8'); echo "The length of the string is: " . $length; ?>
This ensures that multibyte characters are treated correctly, preventing potential issues with string manipulation.
Trimming and Stripping
In real-world scenarios, you may need to trim or strip excess spaces from strings before determining their length. The trim() and str_replace() functions come in handy for these tasks. Consider the following example:
php
<?php $str = " PHP is powerful "; $trimmedLength = strlen(trim($str)); echo "The trimmed length of the string is: " . $trimmedLength; ?>
This not only finds the length of the string but also trims any leading or trailing spaces, providing a cleaner result.
Handling HTML Tags
When working with HTML content, especially if you are dealing with user-generated data, you may want to consider the impact of HTML tags on string length. The strip_tags() function helps remove HTML tags before calculating the string length. Here’s an example:
php
<?php $htmlStr = "<p>PHP is <strong>awesome</strong>!</p>"; $strippedLength = strlen(strip_tags($htmlStr)); echo "The stripped length of the string is: " . $strippedLength; ?>
This ensures that HTML tags don’t interfere with the accurate calculation of the string length.
Advanced String Length Techniques
In more complex scenarios, you might encounter situations where you need to handle string length dynamically, such as limiting the length of a string for display. The substr() function is useful in such cases. Consider the following example:
php
<?php $longStr = "This is a very long string with lots of content."; $maxLength = 20; if(strlen($longStr) > $maxLength) { $shortenedStr = substr($longStr, 0, $maxLength) . "..."; } else { $shortenedStr = $longStr; } echo "The processed string is: " . $shortenedStr; ?>
This example limits the length of the string to 20 characters and appends ellipses (…) if the string exceeds that limit.
Conclusion
Mastering string length manipulation in PHP is essential for efficient and error-free programming. From basic functions like strlen() to handling multibyte characters with mb_strlen() and performing advanced manipulations using functions like substr(), a solid understanding of these techniques empowers you to write cleaner, more robust PHP code. Incorporate these string length methods into your toolkit, and you’ll find yourself better equipped to handle a variety of real-world programming challenges. Happy coding!