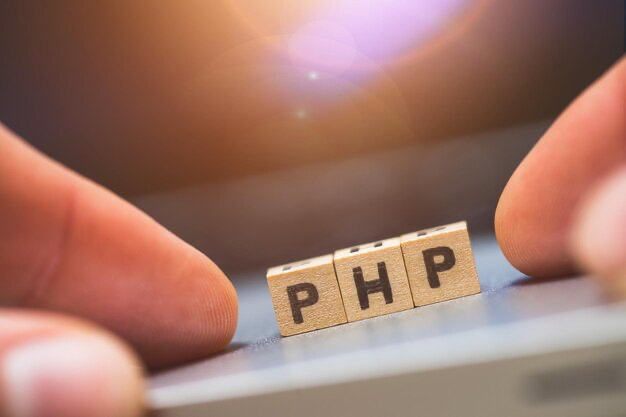
PHP, a robust server-side scripting language, empowers developers to create dynamic web applications. A frequent task in PHP development is string handling. This guide focuses on string manipulation and the use of regular expressions in PHP. Completing this tutorial will give you a comprehensive knowledge of managing strings in PHP and utilizing regular expressions for text matching and alteration. Additionally, if you’re considering hiring PHP developers, this tutorial will serve as a valuable benchmark for evaluating their capabilities.
String Manipulation in PHP
PHP serves developers with a plethora of built-in functions to skillfully manipulate strings. We’ll delve into some of the most popular ones to give you a practical understanding:
- strlen(): Fetches the length of a string;
- substr(): Assists in extracting a specific segment of a string;
- strpos(): Locates the position of the first instance of a substring;
- strstr(): Searches for the first occurrence of a substring;
- str_replace(): Substitutes all instances of a search string with a replacement string;
- strtolower(): Transforms all string characters into lowercase;
- strtoupper(): Transforms all string characters into uppercase.
Example: String Manipulation
To make this technical exploration more effective, let’s dive into an example. Imagine we have the following string:
$string = "Greetings, PHP Developers!";
Now, let’s see some PHP string manipulation techniques in action:
// Retrieve the length of the string
$length = strlen($string);
echo $length; // Output: 26
// Extract a segment of the string
$substring = substr($string, 0, 9);
echo $substring; // Output: Greetings
// Locate the position of the first instance of a substring
$position = strpos($string, "PHP");
echo $position; // Output: 11
// Search for the first occurrence of a substring
$occurrence = strstr($string, "PHP");
echo $occurrence; // Output: PHP Developers!
// Substitute all instances of a search string with a replacement string
$changed_string = str_replace("PHP", "Ruby", $string);
echo $changed_string; // Output: Greetings, Ruby Developers!
// Transform all string characters into lowercase
$lowercase = strtolower($string);
echo $lowercase; // Output: greetings, php developers!
// Transform all string characters into uppercase
$uppercase = strtoupper($string);
echo $uppercase; // Output: GREETINGS, PHP DEVELOPERS!
Regular Expressions in PHP
Regular expressions, commonly referred to as regex, allow for robust pattern matching and manipulation and are well-integrated into PHP. The language accommodates regular expressions through intrinsic functions like preg_match(), preg_replace(), and preg_split(), all of which utilize the Perl Compatible Regular Expressions (PCRE) library. These functionalities encapsulate the versatility of PHP, allowing it to efficiently handle complex pattern identifications and alterations.
Example: Using preg_match()
The preg_match() function is a practical tool for executing a regular expression match. It will return 1 if the pattern is found within the string, 0 if it isn’t, and FALSE if an error arises. Let’s illustrate with an example:
$string = "The intrepid brown fox effortlessly leaps over the languid dog.";
// Verifying if the string comprises the term "fox"
if (preg_match("/fox/", $string)) {
echo "The phrase indeed includes the term 'fox'.";
} else {
echo "The term 'fox' was not found in the phrase.";
}
Example: Using preg_replace()
The preg_replace() function is designed to perform a regular expression search, followed by a replacement. It requires three arguments – the pattern, the replacement, and the initial string. Here is an example to depict its operation:
$string = "The intrepid brown fox effortlessly leaps over the languid dog.";
// Substitute all occurrences of "fox" with "cat"
$modified = preg_replace("/fox/", "cat", $string);
echo $modified; // Output: The intrepid brown cat effortlessly leaps over the languid dog.
Example: Using preg_split()
The preg_split() function is employed to divide a string based on a regular expression. It necessitates two arguments – the pattern, and the string to be split. Let’s go through an example:
$string = "The intrepid brown fox effortlessly leaps over the languid dog.";
// Segment the string by spaces
$segments = preg_split("/\s+/", $string);
print_r($segments);
This command will result in the following output:
Array
(
[0] => The
[1] => intrepid
[2] => brown
[3] => fox
[4] => effortlessly
[5] => leaps
[6] => over
[7] => the
[8] => languid
[9] => dog.
)
Exploring the Realm of PHP Socket Programming
PHP socket programming equips developers with the ability to establish communication channels, facilitating real-time data exchange between server and client applications. The amalgamation of string manipulation, regular expressions, and socket programming expands the horizons of what PHP can achieve, empowering developers to craft dynamic, interactive web applications with seamless data transmission.
Summary
This guide explored the fundamental aspects of string manipulation and the use of regular expressions in PHP. Through PHP’s comprehensive built-in functions, string manipulation and intricate pattern matching and text processing become straightforward. For those seeking to recruit PHP developers, proficiency in these areas is crucial.