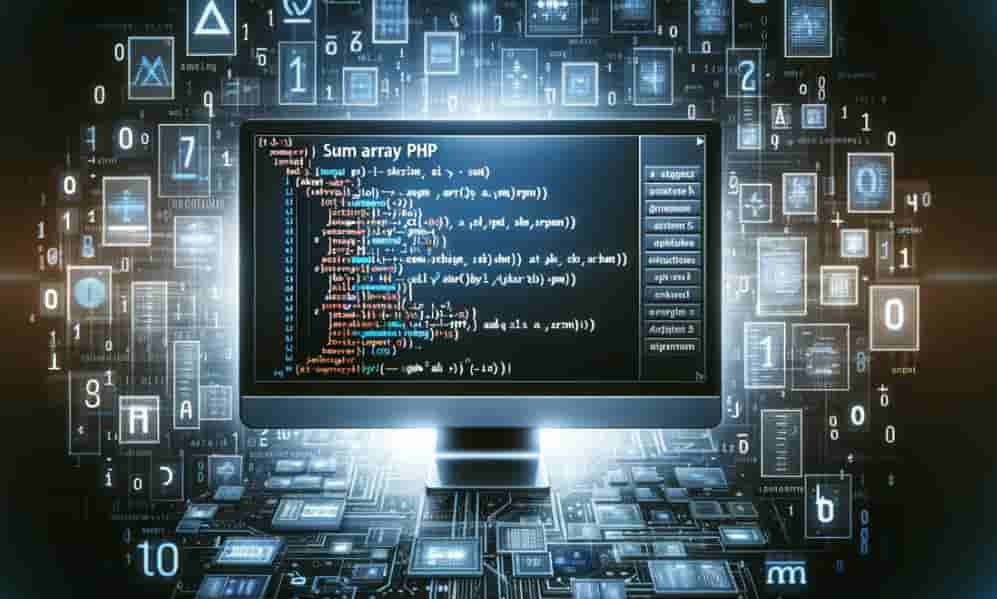
This detailed guide offers an exploration into the world of PHP’s `array_sum()` function from a third-person perspective. Readers will delve into the intricacies of the function’s usage, syntax, and several practical examples. Aimed at both novices and seasoned PHP developers, the tutorial seeks to enrich the audience’s understanding and proficiency with the `array_sum()` function.
The Essence of `array_sum()`
PHP’s `array_sum()` function is a versatile built-in tool that aggregates the values within an array. It adeptly processes numeric and string elements, automatically converting strings to numbers where feasible. Non-numeric elements are excluded from the sum.
The syntax of `array_sum()` is straightforward:
```php
array_sum(array $array): float|int
```
Here, `$array` represents the input array whose elements are to be totaled. The function returns a result as either a float or an integer, contingent on the array’s contents.
Practical Implementation of `array_sum()`
To grasp the functionality of `array_sum()`, consider some basic examples:
Example 1: Numeric Array Summation
In this segment, the process of summing a simple numeric array using PHP’s `array_sum()` function is demonstrated. The array, `$numbers`, is defined with a straightforward sequence of integers: 1, 2, 3, 4, and 5. This type of array is common in programming tasks where numerical data needs to be aggregated or analyzed.
```php
$numbers = array(1, 2, 3, 4, 5);
$sum = array_sum($numbers);
echo "The sum of array values is: " . $sum;
```
Upon invoking `array_sum($numbers)`, PHP internally iterates through each element of the `$numbers` array, accumulating their values. This function is highly efficient for such operations, eliminating the need for manual looping and summation logic. It’s a clear example of PHP’s capability to handle array operations with minimal code, making it a preferred choice for developers dealing with numerical data sets.
The output of this code snippet, as expected, is `The sum of array values is: 15`. This result is displayed through PHP’s `echo` statement, confirming that the function correctly computes the total sum of the numeric values in the array. Such an operation is foundational in many PHP applications, ranging from simple tasks like averaging scores to more complex financial calculations in business applications. The simplicity and versatility of the `array_sum()` function make it an invaluable tool in a PHP programmer’s toolkit, especially when working with arrays containing numerical data.
Example 2: Summation with Mixed Elements
An array composed of mixed elements (numeric and string) can be summed up in a similar fashion:
```php
$mixedValues = array(1, "2", "3.5", "four", 5.5, "10.5");
$sum = array_sum($mixedValues);
echo "The sum of array values is: " . $sum;
```
Resulting Output: `The sum of array values is: 22.5`
In this instance, the non-numeric value “four” is disregarded, and string numbers are converted before summing.
Advanced Uses of `array_sum()`
Beyond basic sums, `array_sum()` finds application in more complex scenarios:
Example 3: Average Calculation
For calculating an array’s average:
```php
$grades = array(85, 90, 78, 92, 88);
$sum = array_sum($grades);
$average = $sum / count($grades);
echo "The average grade is: " . $average;
```
Output: `The average grade is: 86.6`
Example 4: Weighted Average Computation
The computation of a weighted average in PHP, demonstrated in this code snippet, is a slightly more advanced operation that showcases the flexibility and power of PHP’s array functions. Here, two arrays are used: `$values`, containing the data points, and `$weights`, holding the corresponding weights for each data point.
```php
$values = array(85, 90, 78, 92, 88);
$weights = array(0.1, 0.2, 0.3, 0.1, 0.3);
$weightedValues = array_map(function($value, $weight) {
return $value * $weight;
}, $values, $weights);
$weightedAverage = array_sum($weightedValues);
echo "The weighted average is: " . $weightedAverage;
```
In this example, `array_map()` is ingeniously employed to create a new array, `$weightedValues`, where each value from `$values` is multiplied by its corresponding weight from `$weights`. This function illustrates PHP’s ability to handle complex array operations efficiently, applying a callback function to each element of one or more arrays.
The final step involves using `array_sum()` on the `$weightedValues` to calculate the total sum, which is then directly interpreted as the weighted average. The versatility of PHP’s array handling is further highlighted here, as it simplifies what would otherwise be a tedious task of manually iterating through each array element and performing the multiplication and summation.
The code outputs `The weighted average is: 84.6`, demonstrating the accuracy and effectiveness of the approach. This kind of operation is particularly useful in scenarios such as statistical analysis, financial modeling, or in any context where weighted evaluations are needed. It reflects PHP’s capabilities not just as a web development tool, but also as a language suitable for more complex numerical computations.
Conclusion
The guide concludes by emphasizing the importance of the `array_sum()` function in PHP programming. It serves as a fundamental tool for developers, particularly in array manipulation. Understanding and utilizing this function can greatly enhance a developer’s efficiency and effectiveness in PHP projects. This knowledge is a stepping stone towards mastering array operations in PHP, paving the way for more advanced coding endeavors.