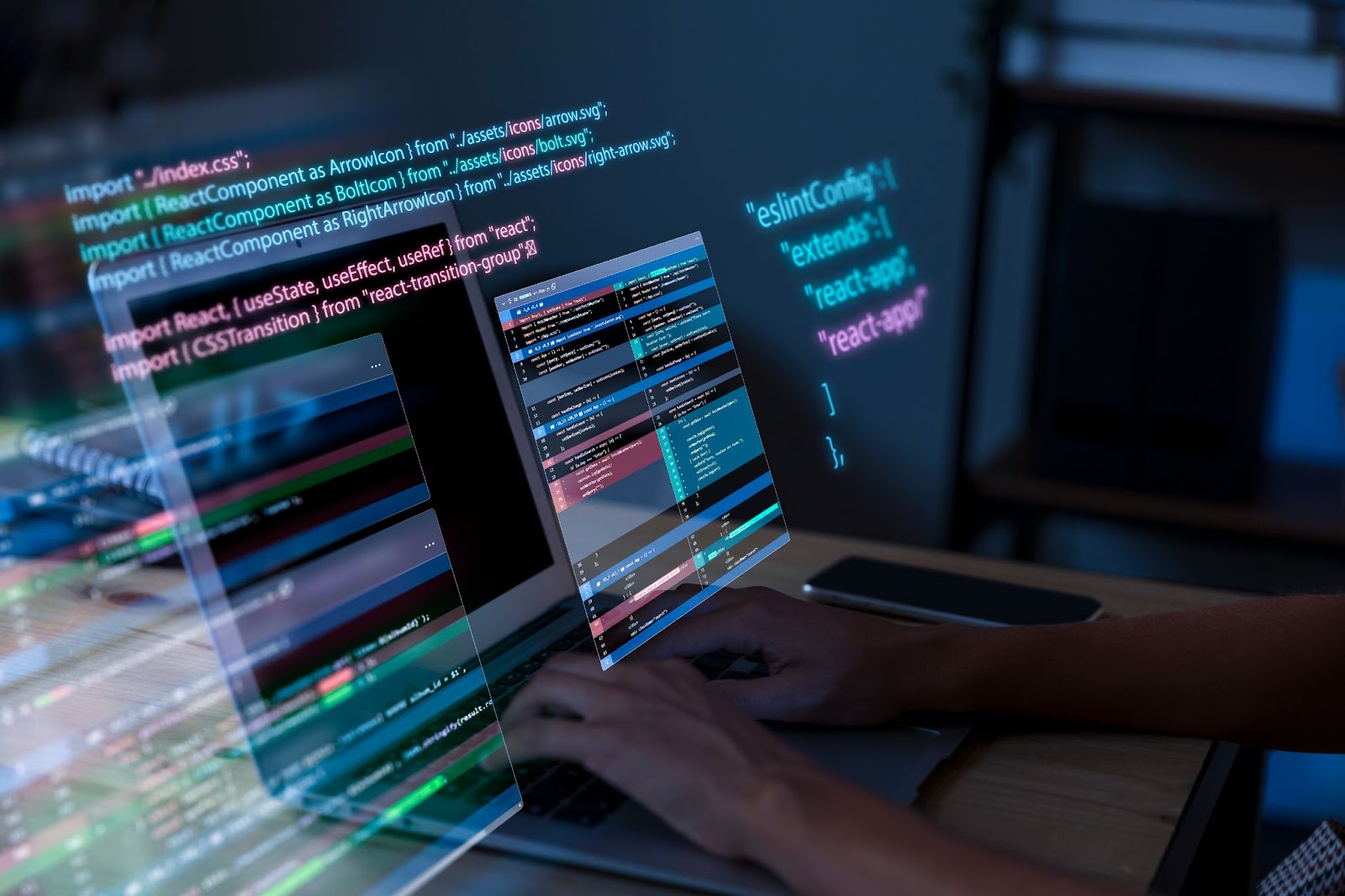
PHP, a widely used scripting language for web development, has evolved over the years to meet the demands of modern web applications. While PHP’s primary use case has traditionally been serving web pages, it has increasingly been employed for a broader range of tasks, including server-side scripting, CLI (Command Line Interface) applications, and even data processing. With the growing need for efficient and high-performance applications, the question of multithreading and parallel execution in PHP has gained prominence.
In this article, we will delve into the fascinating world of multithreading and parallel execution within PHP. We will explore the challenges developers face when attempting to introduce concurrency in a traditionally single-threaded language, as well as the tools, techniques, and libraries available to harness the power of multiple threads for improved performance and responsiveness in PHP applications.
Unlocking the Power of Threads in PHP with the pthreads Extension
If you’re looking to supercharge your PHP applications and enhance their performance, threading can be a game-changer. However, before you can dive into the world of concurrent programming in PHP, you need to install the pthreads extension. Unlike some PHP modules, pthreads doesn’t come bundled with the default PHP installation, so you’ll need to set it up separately. In this comprehensive guide, we’ll walk you through the installation process step by step, ensuring you’re well-prepared to harness the full potential of threads in PHP.
Step 1: Installing the PHP Development Package
The first step on your journey to harnessing the power of threads in PHP is to ensure you have the necessary tools installed. This includes the PHP development package, which provides essential libraries and headers required for compiling PHP extensions. If you don’t already have it, follow these steps to get it:
Recommendation: Keep your system updated by running sudo apt-get update before proceeding with the installation.
Open your terminal and execute the following command to install the PHP development package:
$ sudo apt-get install php-dev
This command will fetch and install the necessary development tools for PHP on your system.
Step 2: Installing the PHP pthreads Extension
With the PHP development package in place, you’re now ready to install the pthreads extension using the PECL package manager. PECL makes it easy to fetch and install PHP extensions effortlessly. Follow these instructions:
Pro Tip: Ensure that your PHP installation is up to date before proceeding.
Launch your terminal and execute the following command to install the pthreads extension:
$ sudo pecl install pthreads
PECL will handle the download and installation of pthreads for you. It may prompt you for confirmation during the process, so be sure to confirm when necessary.
Step 3: Configuring PHP to Use the pthreads Extension
Now that you’ve successfully installed pthreads, it’s time to configure your PHP environment to recognize and utilize the extension. This step is crucial for making threads accessible within your PHP applications. Follow these instructions to configure PHP:
Insight: PHP extensions are usually configured through the php.ini file, which holds various PHP settings. Here, we’ll add the pthreads extension to your php.ini.
Open your php.ini configuration file. You can find its location by running:
$ php -i | grep "php.ini"
Once you’ve identified the php.ini location, open it in your preferred text editor. For example:
$ sudo nano /path/to/your/php.ini
Add the following line to your php.ini file:
extension=pthreads.so
Ensure that this line is placed under the “Dynamic Extensions” section of the php.ini file. Save and close the php.ini file.
Exploring Threads in PHP: A Comprehensive Guide
Threads are an essential concept in PHP for advanced programming tasks. Below is a detailed exploration of threads in PHP, aimed at enhancing the reader’s understanding and practical skills.
Fundamental Concepts of Threads in PHP
- Definition of a Thread:
- A thread is a separate path of execution in a program. It is an independent unit that runs a specific sequence of coded instructions;
- Threads have their own program counter, stack, and a set of instructions but can share common data with other threads.
- Thread Synchronization:
- This process involves the management and coordination of multiple threads in a program;
- It’s crucial for maintaining data integrity and preventing conflicts when threads simultaneously access shared resources;
- Techniques include using locks, mutexes, or semaphores to manage resources.
- Inter-Thread Communication:
- Threads can communicate through shared data structures or by passing messages;
- Effective communication ensures seamless operation between concurrent threads.
Creation and Management of Threads in PHP
PHP provides the Thread class from the pthreads extension to facilitate thread management. Here’s an overview of its usage:
- Utilizing the Thread Class:
- The Thread class contains methods for initiating and controlling thread behavior;
- Key methods include start(), join(), isRunning(), and isTerminated().
- Practical Implementation;
- start(): Initiates the thread’s execution.
- join(): Halts the execution of the current script until the thread completes;
- isRunning(): Determines if a thread is actively executing;
- isTerminated(): Checks if a thread has finished its execution.
Example of Thread Creation in PHP:
class MyThread extends Thread {
public function run() {
echo "Hello, I'm a new thread!" . PHP_EOL;
}
}
$thread = new MyThread();
$thread->start();
$thread->join();
Enhancing Thread Synchronization and Communication in PHP
Thread synchronization and communication in PHP can significantly boost the efficiency and performance of your applications, especially for CPU-intensive tasks. The pthreads extension offers a valuable resource in the form of the Threaded class, equipped with several methods designed to facilitate synchronization and communication among threads. Let’s delve deeper into this topic, exploring the methods and providing a practical example of how to use them effectively.
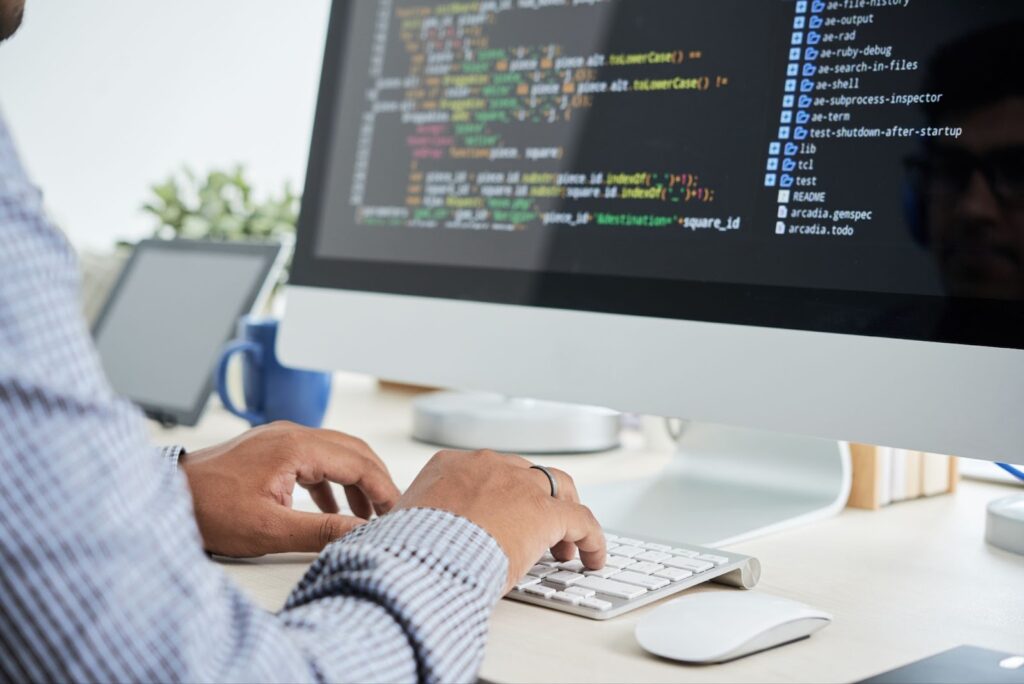
Threaded Class Methods for Synchronization and Communication
The Threaded class provides a set of methods to manage synchronization and communication between threads:
- synchronized();
- Acquires a lock on the object;
- Executes the provided callback in a thread-safe manner;
- Releases the lock;
- wait();
- Puts a thread to sleep until it receives a signal from another thread;
- notify();
- Sends a signal to wake up a waiting thread.
These methods are fundamental to building a reliable communication framework among threads. Now, let’s see how these methods come into play with a practical example.
Practical Example of Thread Synchronization and Communication
Consider a scenario where you have a producer thread adding data to a shared MyThreaded object and a consumer thread consuming this data. Here’s a step-by-step breakdown of the code:
class MyThreaded extends Threaded {
public function add($value) {
$this->synchronized(function (MyThreaded $threaded, $value) {
$threaded[] = $value;
$threaded->notify();
}, $this, $value);
}
}
class Producer extends Thread {
private $data;
public function __construct(MyThreaded $data) {
$this->data = $data;
}
public function run() {
for ($i = 0; $i < 10; $i++) {
$this->data->add($i);
echo "Added: $i" . PHP_EOL;
sleep(1);
}
}
}
class Consumer extends Thread {
private $data;
public function __construct(MyThreaded $data) {
$this->data = $data;
}
public function run() {
while (!$this->data->isEmpty()) {
$this->data->synchronized(function (MyThreaded $data) {
if (!$data->isEmpty()) {
echo "Consumed: " . $data->shift() . PHP_EOL;
}
$data->wait();
}, $this->data);
}
}
}
$data = new MyThreaded();
$producer = new Producer($data);
$consumer = new Consumer($data);
$producer->start();
$consumer->start();
$producer->join();
$consumer->join();
Explanation and Workflow:
- The producer thread adds data to the MyThreaded object and notifies the consumer thread;
- The consumer thread waits for the notification and then consumes the data;
- This cycle continues until there is no more data to consume;
- By implementing this pattern, you ensure that the producer and consumer threads work seamlessly, avoiding race conditions and ensuring safe data sharing.
Conclusion
In conclusion, exploring the world of multithreading and parallel execution in PHP opens up exciting opportunities for developers seeking to enhance the performance and scalability of their applications. While PHP traditionally hasn’t been known for its multithreading capabilities, recent developments and extensions have paved the way for concurrent processing and improved resource utilization.
By delving into the concepts of multithreading and parallel execution, PHP developers can harness the power of modern computing hardware and distribute tasks more efficiently. Whether it’s speeding up data processing, handling multiple concurrent requests, or optimizing resource-intensive operations, multithreading in PHP provides a valuable tool to achieve these goals.