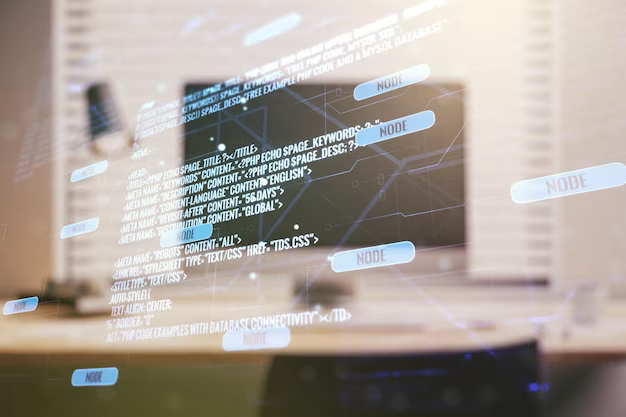
In this guide, we’ll delve into PHP’s native functions urlencode() and urldecode(), designed for encoding and decoding URL strings. These crucial tools for web developers help guarantee that URLs are accurately processed by web servers and browsers.
Introduction to URL Encoding and Decoding
URL encoding, sometimes referred to as percent-encoding, involves transforming characters within a URL into a format suitable for secure transmission across the internet. This step is crucial since certain characters, like spaces and special symbols, hold specific significances in URLs, leading to errors if not encoded correctly.
Conversely, URL decoding is about reverting these encoded characters to their original state. This process is essential when handling URLs in PHP scripts, enabling you to interact with the real data as opposed to its encoded form.
Harnessing PHP’s urlencode() Function
In PHP, the urlencode() function is a powerful tool that transmutes a simple string into a URL-friendly version.
Explaining the Syntax
Looking at its syntax, it’s quite straightforward:
string urlencode(string $string);
In essence, it accepts a string as an argument and turns it into a URL-friendly string.
Real-Life Example
To comprehend it better, let’s consider a real-world example. Suppose you have the following string you wish to encode:
$string = "Hello, world!";
In this scenario, the urlencode() function comes into play to encode the string:
$encoded_string = urlencode($string);
echo $encoded_string; // Output: Hello%2C+world%21
As observed, the function replaced the space with a plus symbol (+) and the special characters with their corresponding percent-encoded values (also known as percent-encoding).
Using PHP’s urldecode() Function
While PHP’s urlencode() function transmutes a simple string into a URL-friendly version, the urldecode() function performs the opposite task. It converts an encoded URL back into an understandable string.
Understanding the urldecode() Function
The urldecode() function syntax can be summed up as follows:
string urldecode(string $string);
It essentially accepts an encoded string as an argument and restores it back to its original format.
Taking a Practical Approach
To illustrate this, let’s say you have the following encoded string:
$encoded_string = "Hello%2C+world%21";
In this case, you can use the urldecode() function to retrieve the original string:
$decoded_string = urldecode($encoded_string);
echo $decoded_string; // Output: Hello, world!
Leveraging PHP’s urlencode() and urldecode() for GET Parameters
When working with GET parameters in PHP, it’s imperative to use the urlencode() and urldecode() functions aptly. Proper handling of these parameters can help you prevent potential errors and ward off security issues. Let’s take a detailed look at how to effectively use these functions with GET parameters:
Encoding GET Parameters with urlencode()
The urlencode() function can be used to encode GET parameters when creating a URL. Here’s an illustrative example:
$search_query = "The Ultimate Guide to PHP's urlencode() function";
$encoded_query = urlencode($search_query);
// Construct a URL with the encoded query parameter
$url = "https://yourwebsite.com/resources.php?topic=" . $encoded_query;
In this example, the search query is suitably encoded using the urlencode() function before being appended to the URL.
Decoding GET Parameters using urldecode()
When it’s time to process GET parameters in your PHP script, you’d need to decode them back to their original form for efficient handling. Here’s how you can accomplish this:
// Extract the encoded query parameter from the URL
$encoded_query = $_GET['topic'];
// Decipher the query parameter
$decoded_query = urldecode($encoded_query);
Efficient URL Handling in PHP: Mastering Best Practices
Working with URLs in PHP implies engaging in a delicate balancing act, where precision and security go hand in hand. Following best practices while using urlencode() and urldecode() can not only avert errors but also bolster security. Here are some key guidelines to consider when dealing with URLs in PHP:
- Encode with Vigilance: When constructing URLs, especially ones incorporating GET parameters, always encode the parameters using urlencode(). This practice helps in mitigating errors and augmenting security;
- Decode Before Processing: When you’re ready to process GET parameters in PHP scripts, ensure to decode them first using urldecode(). This step transforms the encoded parameters back to their original format, making them ready for processing;
- Avoid Double-Encoding: Double-encoding refers to a situation where a string is encoded more than once, leading to issues during decoding. This can trigger errors and should be avoided for smooth operations;
- Consider rawurlencode() and rawurldecode(): If you’re dealing with URLs that adhere to the RFC 3986 standard (which encodes spaces as %20, not +), consider using rawurlencode() and rawurldecode(). These functions can be particularly useful in maintaining compliance with this standard.
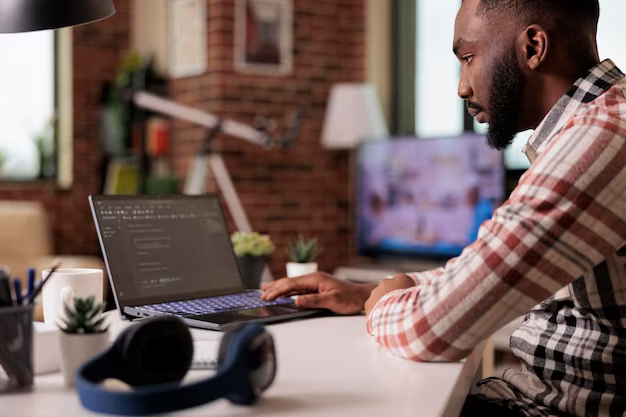
PHP Alternatives for URL Encoding and Decoding
PHP offers a pair of functions, aside from urlencode() and urldecode(), specifically for URL encoding and decoding:
- rawurlencode(): Differing from urlencode() in encoding spaces as %20 instead of a plus sign, this function adheres to the RFC 3986 standard;
- rawurldecode(): Acting as the complement to rawurlencode(), it decodes strings encoded in URL format according to the RFC 3986 standard.
These functions serve the same purposes as urlencode() and urldecode(), but are better suited for scenarios like interfacing with APIs that demand URLs compliant with RFC 3986.
Generating PDF Files in PHP
Expanding our horizons within the realm of PHP, let’s briefly touch upon the topic of generating PDF files. PHP provides robust libraries and tools for creating PDF documents dynamically, enriching the versatility of your web applications. Whether it’s generating invoices, reports, or interactive forms, PHP equips you with the means to produce high-quality PDF files effortlessly. By integrating PDF generation into your PHP projects, you enhance their capabilities and cater to a wider range of user needs.
Conclusion
This guide has delved into the functionalities of PHP’s urlencode() and urldecode(), highlighting their crucial role in developing secure and user-friendly web applications. Gaining proficiency in encoding and decoding URL strings not only enhances your ability to effectively utilize PHP but also empowers you to recruit skilled PHP developers and construct dependable web applications.