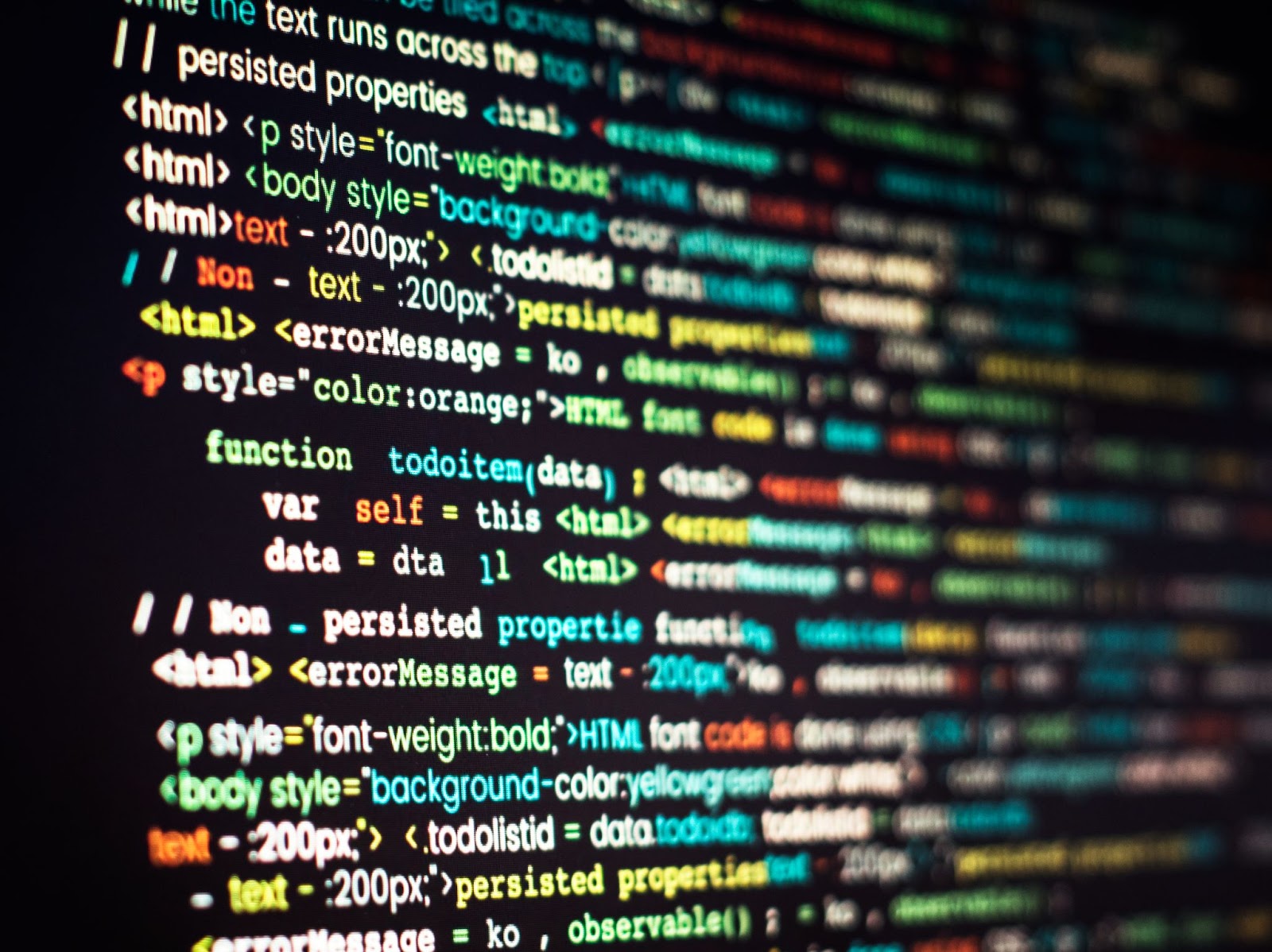
In this instructional guide, we will delve into the art of streamlining iteration processes within PHP by harnessing the capabilities of generators, in conjunction with the yield keyword. Generators represent a potent innovation that was introduced in PHP 5.5, affording developers the opportunity to craft code that not only conserves memory but also exhibits a heightened level of cleanliness and elegance. By acquiring a comprehensive understanding of generator mechanics and mastering the art of leveraging the yield keyword, you will empower yourself to enhance your proficiency in PHP and elevate the efficiency of your development endeavors.
Understanding Generators in Programming
Generators represent a specialized category of iterators, distinct in their handling and functionalities compared to traditional data structures like arrays. Their primary attribute lies in their efficient memory management, especially when dealing with extensive data sets. Let’s delve into the intricacies of generators, exploring their creation, operation, and practical applications.
Key Characteristics of Generators
- Memory Efficiency: Unlike arrays that store entire data sets in memory, generators dynamically produce data elements one at a time. This trait significantly reduces memory usage, particularly beneficial when processing large volumes of data;
- On-Demand Data Generation: Generators create data elements on-the-fly during iteration. This means data is generated only when required, enhancing performance and resource utilization.
How Generators are Created
- Use of yield Keyword: A hallmark of generator functions is the use of the yield keyword. This keyword, when used in a function, transforms it into a generator. It essentially pauses the function’s execution and returns a value, resuming from the same point upon the next call;
- Generator Objects: When a generator function is invoked, it doesn’t execute its code immediately. Instead, it returns a generator object. This object adheres to the iterator protocol, enabling you to traverse through the data set using loops, typically a foreach loop in many programming languages.
The Magic of the Yield Keyword: Enhancing Generators in Programming
Generators are a fascinating concept in programming, allowing you to work with potentially infinite sequences of data in a memory-efficient way. At the heart of this magic is the “yield” keyword, a powerful tool for producing values during iterations. In this article, we’ll dive into the world of generators and explore the versatile capabilities of the yield keyword.
Understanding the Yield Keyword
Imagine a generator function as a special type of function that can pause its execution and then resume it, all while maintaining its internal state. The yield keyword is the key player in this process. Here’s a closer look at how it works:
- Pause and Produce: When a generator function encounters a “yield” statement, it momentarily pauses its execution and returns the yielded value to the caller. This ability to pause and produce results is what makes generators unique;
- Resuming Execution: The magic happens when you call the generator function again. Execution resumes immediately after the last “yield” statement, allowing the function to pick up right where it left off. This feature is invaluable for maintaining state across multiple function calls.
A Practical Example
To solidify our understanding, let’s explore a basic example in PHP:
function countToThree() {
yield 1;
yield 2;
yield 3;
}
$generator = countToThree();
foreach ($generator as $value) {
echo $value . PHP_EOL;
}
In this code snippet:
- We define a generator function called countToThree that yields the values 1, 2, and 3;
- We create a generator instance with $generator = countToThree();
- Using a foreach loop, we iterate over the generator and echo each value.
The output will be:
1
2
3
Expanding Your Understanding of the Yield Keyword
Now that we’ve covered the basics, let’s delve deeper into the potential of the yield keyword:
- Infinite Sequences: Generators are excellent for handling sequences that are too large to fit into memory. With the yield keyword, you can create generators for infinite sequences, like the Fibonacci sequence or prime numbers, without worrying about memory constraints;
- Dynamic Data Generation: Generators are dynamic. You can use conditional logic, mathematical operations, or even input from external sources to yield values. This flexibility makes them ideal for generating data on-the-fly;
- Stateful Iterations: The ability to pause and resume execution allows you to maintain state. You can use this to implement complex algorithms or simulations where maintaining context between iterations is essential;
- Memory Efficiency: Unlike arrays or lists, generators don’t store all values in memory at once. They produce values on-demand, making them memory-efficient for working with large datasets;
- Exception Handling: You can also use the yield keyword to handle exceptions gracefully within your generator functions. This can enhance the robustness of your code;
- Iterating over Custom Data Sources: Generators can be used to iterate over custom data sources, like files or network streams. You can read and yield data in chunks, reducing the need to load the entire dataset into memory;
- Generators in Different Languages: While we’ve focused on PHP, the yield keyword is available in various programming languages, including Python, JavaScript (ES6), and Ruby. Learning how to use it in your preferred language can unlock new programming possibilities.
Utilizing Yield for Efficient Processing of Large Data Sets
Imagine you’re dealing with a massive CSV file packed with user data, and your task is to process each row of this behemoth. The traditional approach of loading the entire file into memory can quickly turn your system sluggish and unresponsive. However, fret not! There’s a more efficient and memory-friendly way to tackle this challenge using PHP generators.
The Power of Generators
In PHP, generators offer a dynamic solution to this problem. They allow you to read and process each row one at a time, conserving precious memory resources. Let’s dive into this practical example to grasp the concept better.
Creating the CSV Generator Function
function readCsvFile($filename) {
$file = fopen($filename, 'r');
while (($row = fgetcsv($file)) !== false) {
yield $row;
}
fclose($file);
}
In the above code snippet:
- We define a function named readCsvFile that takes the CSV file’s filename as an argument.
- Inside the function, we open the file in read mode using fopen;
- We then enter a while loop that iterates through the file’s contents line by line;
- For each line, we use the fgetcsv function to extract the data as an array, which represents a single row of the CSV;
- Crucially, we employ the yield keyword to return each row as a generator element. This is the magic that ensures memory-efficient processing.
Iterating Through the CSV Generator
Now, let’s put our generator to work:
$csvGenerator = readCsvFile('large_file.csv');
foreach ($csvGenerator as $row) {
// Process the row
}
Here, we create a $csvGenerator instance by calling the readCsvFile function with the filename of our large CSV file. As we iterate through the generator using a foreach loop, we can process each row individually, keeping memory consumption under control.
Unlocking the Power of Key-Yielding Generators
In the realm of programming, generators are versatile tools that not only yield values but also bestow the ability to yield keys alongside their corresponding values. This fascinating feature opens up a world of possibilities, allowing developers to construct associative arrays dynamically. In this comprehensive exploration, we will delve into the art of generating key-value pairs using generators in PHP. Prepare to expand your horizons and discover the potential that awaits.
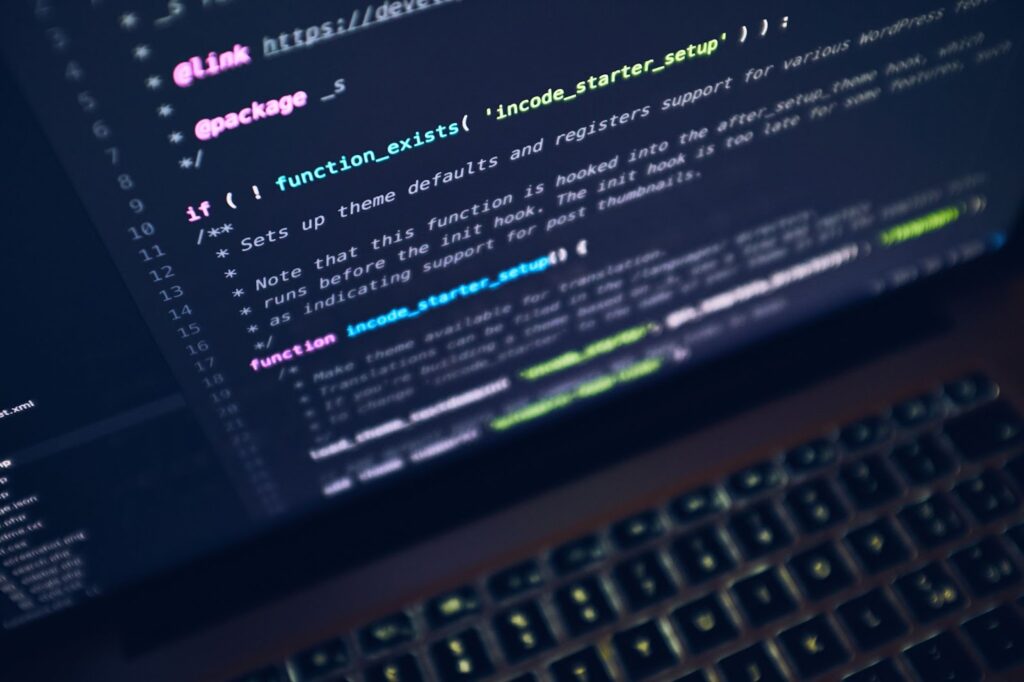
Creating Key-Value Pairs with Generators
To yield a key-value pair using generators in PHP, we employ a simple and elegant syntax:
yield $key => $value;
This compact piece of code packs a punch, as it enables us to define key-value pairs seamlessly. Let’s take a closer look at how this concept translates into action with a practical example.
A Practical Example: Key-Value Generator in PHP
Consider the following PHP code snippet:
function keyValueGenerator() {
yield "one" => 1;
yield "two" => 2;
yield "three" => 3;
}
$keyValueGenerator = keyValueGenerator();
foreach ($keyValueGenerator as $key => $value) {
echo $key . ': ' . $value . PHP_EOL;
}
This code snippet initiates a generator function named keyValueGenerator. Within this function, we yield key-value pairs, assigning keys (“one,” “two,” and “three”) to their respective values (1, 2, and 3). After initializing the generator, we traverse it within a foreach loop, which allows us to access and display the key-value pairs.
The Output: A Glimpse into the Generated Key-Value Pairs
When we run the above code, we are greeted with an output that reveals the magic of key-yielding generators:
one: 1
two: 2
three: 3
Conclusion
Generators, coupled with the ‘yield’ keyword, offer a potent means to streamline iteration in PHP, all the while curbing memory usage. Mastering the art of harnessing these functionalities empowers you to craft code that is not only more resource-efficient but also exhibits a higher degree of elegance. Consider enlisting the expertise of proficient PHP developers well-versed in leveraging generators to seamlessly incorporate these strategies into your project endeavors.